How to read and process emails using php extension IMAP
How to use PHP extension IMAP to read and process emails
Introduction:
IMAP (Internet Mail Access Protocol) is a protocol for receiving and managing email. In PHP, you can use the IMAP extension to read and process emails, which allows you to perform functions such as receiving emails, searching for emails, deleting emails, etc. This article explains how to use PHP's IMAP extension to read and process email, and provides some code examples.
1. Install the IMAP extension:
First, make sure your PHP has the IMAP extension installed. In PHP 7.0 and above, the IMAP extension has become a core module of PHP. If your PHP version is lower than 7.0, you will need to manually install the IMAP extension. You can find instructions on how to install the IMAP extension on the extensions page of the official PHP website.
2. Connect to the mailbox server:
Before using the IMAP extension to read emails, you need to establish a connection with the mailbox server. You can open an IMAP connection through the imap_open
function. The example is as follows:
$hostname = '{imap.example.com:993/imap/ssl}INBOX'; $username = 'your_username'; $password = 'your_password'; $mailbox = imap_open($hostname, $username, $password); if (!$mailbox) { die('Unable to connect to mailbox: ' . imap_last_error()); } // 其他操作... imap_close($mailbox);
In the above example, the $hostname
variable defines the address and port number of the email server. Among them, imap.example.com
is the domain name of the email server, and 993
is the default secure port number of IMAP. The $username
and $password
variables store the username and password of the mailbox respectively.
3. Read emails:
After connecting to the mailbox server, you can use the imap_search
function to search for emails and return the email identifier (UID). The example is as follows:
$mails = imap_search($mailbox, 'ALL'); if ($mails === false) { die('Unable to search for emails: ' . imap_last_error()); } foreach ($mails as $mailUID) { // 读取邮件 $header = imap_headerinfo($mailbox, $mailUID); $subject = $header->subject; $from = $header->from[0]->mailbox . '@' . $header->from[0]->host; $date = date('Y-m-d H:i:s', $header->udate); echo "Subject: $subject "; echo "From: $from "; echo "Date: $date "; // 读取邮件正文 $body = imap_body($mailbox, $mailUID); echo "Body: $body "; // 其他操作... echo "------------------------------------------------- "; }
In the above example, the imap_search
function receives two parameters, the first parameter is the mailbox connection handle, and the second parameter is the search condition. 'ALL'
means search all messages. The imap_headerinfo
function and the imap_body
function are used to read the header information and body content of the email respectively.
4. Delete emails:
Sometimes you need to delete emails. You can use the imap_delete
function to mark the emails as deleted, and then use the imap_expunge
function to permanently delete the emails. Marked emails, the example is as follows:
$mails = imap_search($mailbox, 'SUBJECT "Test Email"'); if ($mails === false) { die('Unable to search for emails: ' . imap_last_error()); } foreach ($mails as $mailUID) { // 标记邮件为已删除 imap_delete($mailbox, $mailUID); } // 永久删除已标记的邮件 imap_expunge($mailbox); // 其他操作...
In the above example, the second parameter of the imap_search
function specifies the search criteria, here SUBJECT "Test Email"## is used # To search for emails with the subject "Test Email". The
imap_delete function is used to mark messages as deleted, while the
imap_expunge function is used to permanently delete marked messages.
Reading and processing emails is easy using PHP’s IMAP extension. This article describes how to install the IMAP extension, connect to a mailbox server, read mail, and delete mail, and provides corresponding code examples. I hope this article helps you in your efforts to read and process email using PHP extension IMAP.
The above is the detailed content of How to read and process emails using php extension IMAP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
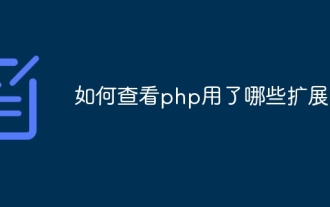
You can check which extensions are used by PHP by viewing the phpinfo() function output, using command line tools, and checking the PHP configuration file. 1. View the phpinfo() function output, create a simple PHP script, save this script as phpinfo.php, and upload it to your web server. Access this file in the browser and use the browser's search function. Just look for the keyword "extension" or "extension_loaded" on the page to find information about the extension.
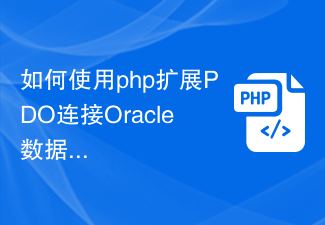
How to use PHP to extend PDO to connect to Oracle database Introduction: PHP is a very popular server-side programming language, and Oracle is a commonly used relational database management system. This article will introduce how to use PHP extension PDO (PHPDataObjects) to connect to Oracle database. 1. Install the PDO_OCI extension. To connect to the Oracle database, you first need to install the PDO_OCI extension. Here are the steps to install the PDO_OCI extension: Make sure
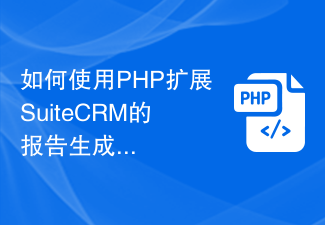
How to use PHP to extend the report generation function of SuiteCRM SuiteCRM is a powerful open source CRM system that provides rich functions to help enterprises manage customer relationships. One of the important functions is report generation. Using reports can help enterprises better understand their business situations and make correct decisions. This article will introduce how to use PHP to extend the report generation function of SuiteCRM and provide relevant code examples. Before starting, you need to make sure that SuiteCRM is installed.
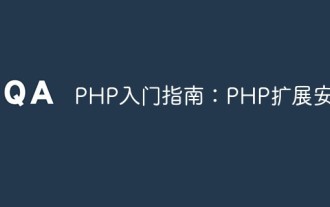
When developing with PHP, we may need to use some PHP extensions. These extensions can provide us with more functions and tools, making our development work more efficient and convenient. But before using these extensions, we need to install them first. This article will introduce you to how to install PHP extensions. 1. What is a PHP extension? PHP extensions refer to components that provide additional functionality and services to the PHP programming language. These components can be installed and used through PHP's extension mechanism. PHP extension can help us with
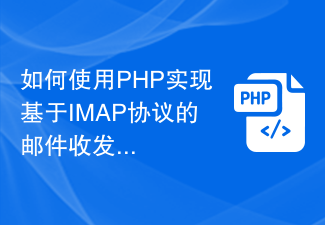
How to use PHP to implement email sending and receiving communication based on IMAP protocol [Introduction] In today's modern society, email has become one of the important communication tools for people. The IMAP (Internet Mail Access Protocol) protocol is widely used in the communication process of sending and receiving emails. This article will introduce how to use PHP language to implement email sending and receiving functions through IMAP protocol, and attach relevant code examples. [Basic knowledge] Before starting to write code, let’s first understand
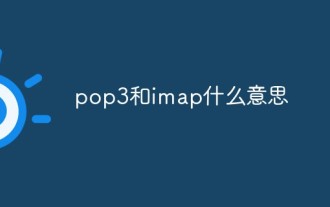
pop3 is the third version of the post office protocol, which stipulates the protocol for personal computers to connect to Internet mail servers and download e-mails; imap is the Internet mail access protocol, and mail clients can obtain mail information on the mail server through this protocol. Download and send emails, etc.
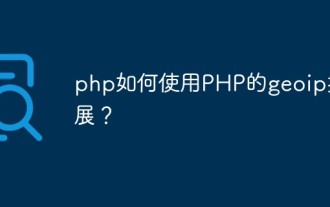
PHP is a popular server-side scripting language that can handle dynamic content on web pages. The geoip extension for PHP allows you to get information about the user's location in PHP. In this article, we’ll cover how to use PHP’s geoip extension. What is the GeoIP extension for PHP? The geoip extension for PHP is a free, open source extension that allows you to obtain data about IP addresses and location information. This extension can be used with the GeoIP database, a database developed by MaxMin
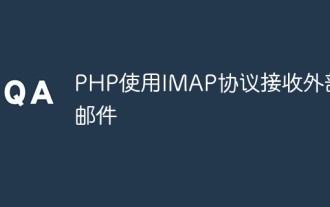
With the popularity of the Internet, email has become an indispensable communication tool in people's daily life and work. How to receive external emails on your own website has become a problem that website developers need to face. IMAP (InternetMailAccessProtocol) protocol is a standard protocol for receiving email. The function of receiving external emails can be easily implemented using the IMAP protocol in PHP. 1. Preparation work Before using PHP to receive emails, you need to make sure
