Best practice for saving remote images to the server in PHP
Best practice for PHP to save remote pictures to the server
In web development, we often encounter the need to save remote pictures to the server. For example, you may need to grab an image from another website, or the user may have uploaded a remote image link. This article will introduce how to use PHP to implement this best practice of saving remote images to the server.
First, we need a URL of a remote image. Suppose the URL of the image we want to save is: http://example.com/image.jpg.
Next, we need to use PHP's file operation function to save the remote image to the server. The following is a common approach:
<?php // 远程图片URL $remoteImageUrl = 'http://example.com/image.jpg'; // 指定保存路径和文件名 $savePath = 'path/to/save/'; $saveFilename = 'saved_image.jpg'; // 创建保存路径 if (!file_exists($savePath)) { mkdir($savePath, 0777, true); } // 保存图片 file_put_contents($savePath . $saveFilename, file_get_contents($remoteImageUrl)); // 输出保存结果 if (file_exists($savePath . $saveFilename)) { echo "远程图片保存成功!"; } else { echo "远程图片保存失败!"; } ?>
In the above code, we first specify the URL of the remote image, and then specify the save path and file name. Next, we created the save path and made sure the save path was writable. Finally, we use the file_put_contents function to save the contents of the remote image to the specified path. After the saving is completed, we judge the saving result by judging whether the saved file exists.
The above method can meet the basic needs, but may encounter some problems in practical application. For example, the saved picture may be a large picture, and using the above method is likely to cause memory overflow. To solve this problem, we can use the curl library to save in chunks. The following is a sample code for saving images using the curl library:
<?php // 远程图片URL $remoteImageUrl = 'http://example.com/image.jpg'; // 指定保存路径和文件名 $savePath = 'path/to/save/'; $saveFilename = 'saved_image.jpg'; // 创建保存路径 if (!file_exists($savePath)) { mkdir($savePath, 0777, true); } // 初始化curl $ch = curl_init($remoteImageUrl); // 设定保存文件 $fp = fopen($savePath . $saveFilename, 'wb'); // 设置curl选项 curl_setopt($ch, CURLOPT_FILE, $fp); curl_setopt($ch, CURLOPT_HEADER, 0); // 执行curl请求 curl_exec($ch); // 关闭curl和文件句柄 curl_close($ch); fclose($fp); // 输出保存结果 if (file_exists($savePath . $saveFilename)) { echo "远程图片保存成功!"; } else { echo "远程图片保存失败!"; } ?>
The above code first uses the curl_init function to initialize a curl request, taking the remote image URL as a parameter. We then use the fopen function to open a file handle and pass it to curl as a save file. Next, we set some options through the curl_setopt function, such as turning off request headers. Finally, use curl_exec to execute the curl request and save the remote image to the specified path. After the save is completed, we close the curl request and file handle, and judge the save result by judging whether the saved file exists.
By using the curl library, we can better control the process of saving images and avoid problems such as memory overflow.
To summarize, the best practice for saving remote images to the server is to use the curl library to save in chunks. This allows for better control over the saving process and avoids problems such as memory overflow. Whether you use the file_put_contents function or the curl library, you need to pay attention to setting the writable permissions of the save path. I hope this article can help you save remote images to the server in web development.
The above is the detailed content of Best practice for saving remote images to the server in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
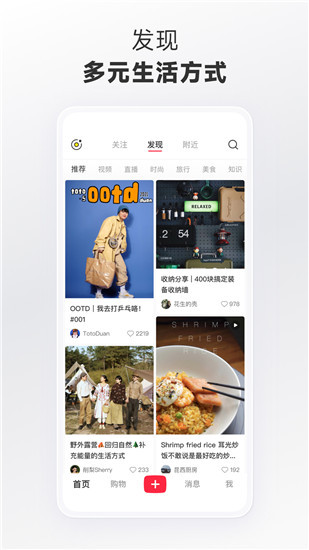
Xiaohongshu has rich content that everyone can view freely here, so that you can use this software to relieve boredom every day and help yourself. In the process of using this software, you will sometimes see various beautiful things. Many people want to save pictures, but the saved pictures have watermarks, which is very influential. Everyone wants to know how to save pictures without watermarks here. The editor provides you with a method for those in need. Everyone can understand and use it immediately! 1. Click the "..." in the upper right corner of the picture to copy the link 2. Open the WeChat applet 3. Search the sweet potato library in the WeChat applet 4. Enter the sweet potato library and confirm to get the link 5. Get the picture and save it to the mobile phone album
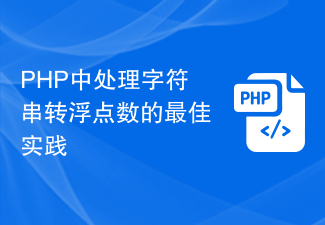
Converting strings to floating point numbers in PHP is a common requirement during the development process. For example, the amount field read from the database is of string type and needs to be converted into floating point numbers for numerical calculations. In this article, we will introduce the best practices for converting strings to floating point numbers in PHP and give specific code examples. First of all, we need to make it clear that there are two main ways to convert strings to floating point numbers in PHP: using (float) type conversion or using (floatval) function. Below we will introduce these two
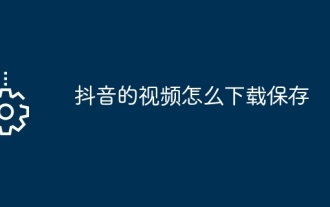
1. Open the Douyin app, find the video you want to download and save, and click the [Share] button in the lower right corner. 2. In the pop-up window that appears, slide the function buttons in the second row to the right, find and click [Save Local]. 3. A new pop-up window will appear at this time, and the user can see the download progress of the video and wait for the download to complete. 4. After the download is completed, there will be a prompt of [Saved, please go to the album to view], so that the video just downloaded will be successfully saved to the user's mobile phone album.
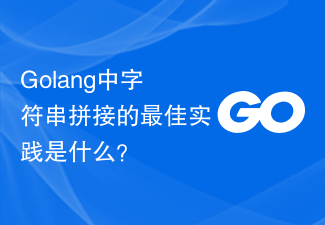
What are the best practices for string concatenation in Golang? In Golang, string concatenation is a common operation, but efficiency and performance must be taken into consideration. When dealing with a large number of string concatenations, choosing the appropriate method can significantly improve the performance of the program. The following will introduce several best practices for string concatenation in Golang, with specific code examples. Using the Join function of the strings package In Golang, using the Join function of the strings package is an efficient string splicing method.
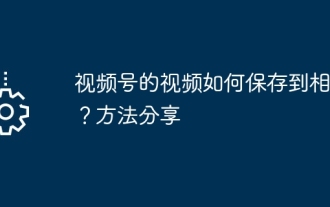
Video account is a popular short video application that allows users to shoot, edit and share their own videos. However, sometimes we may want to save these amazing videos to our photo album so that we can always look back at them when needed. So, next I will share some methods to teach you how to save the video of the video account to the album. Videos can be saved through the built-in function of the Video Number application. Open the app and find the video you want to save. Click the options icon in the lower right corner of the video, a menu will pop up, select "Save to Album". This will save the video to your phone's photo album. Method two is to save the video by taking a screenshot. This method is relatively straightforward, but the saved image will contain elements such as video control bars, which is not pure enough. you

In Go language, good indentation is the key to code readability. When writing code, a unified indentation style can make the code clearer and easier to understand. This article will explore the best practices for indentation in the Go language and provide specific code examples. Use spaces instead of tabs In Go, it is recommended to use spaces instead of tabs for indentation. This can avoid typesetting problems caused by inconsistent tab widths in different editors. The number of spaces for indentation. Go language officially recommends using 4 spaces as the number of spaces for indentation. This allows the code to be
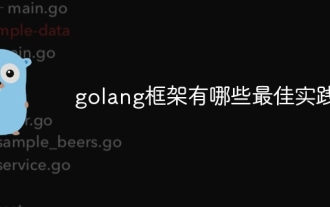
When using Go frameworks, best practices include: Choose a lightweight framework such as Gin or Echo. Follow RESTful principles and use standard HTTP verbs and formats. Leverage middleware to simplify tasks such as authentication and logging. Handle errors correctly, using error types and meaningful messages. Write unit and integration tests to ensure the application is functioning properly.
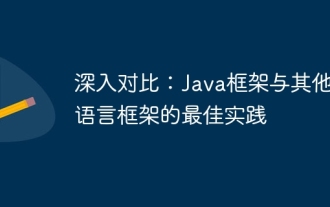
Java frameworks are suitable for projects where cross-platform, stability and scalability are crucial. For Java projects, Spring Framework is used for dependency injection and aspect-oriented programming, and best practices include using SpringBean and SpringBeanFactory. Hibernate is used for object-relational mapping, and best practice is to use HQL for complex queries. JakartaEE is used for enterprise application development, and the best practice is to use EJB for distributed business logic.
