


Second-hand recycling website developed in PHP provides inventory management functions
The second-hand recycling website developed by PHP provides inventory management functions
With the rise of the second-hand item trading market, second-hand recycling websites have become the preferred platform for many people to deal with idle items. In order to meet users' management needs for item inventory, we will develop a powerful second-hand recycling website through PHP and provide inventory management functions.
1. Design Overview
We first need to create a database to store item information and inventory. In the MySQL database, we create a table named "inventory", containing the following fields:
- id: unique identifier of the item
- name: name of the item
- description: Item description
- quantity: Item inventory quantity
2. Database connection
Before connecting to the database, we need to set the database connection in the PHP code parameter. For example, we can create a file named "config.php" to store database connection information, as shown below:
<?php $host = "localhost"; $username = "root"; $password = ""; $database = "inventory"; $conn = mysqli_connect($host, $username, $password, $database); // 检查数据库连接是否成功 if (!$conn) { die("数据库连接失败:" . mysqli_connect_error()); } ?>
3. Add items
In the website, we provide a form Let users add item information. After the user submits the form, we need to process the form data and insert the data into the database. For example, we can create a file named "add_item.php" to handle requests to add items as follows:
<?php include "config.php"; $name = $_POST['name']; $description = $_POST['description']; $quantity = $_POST['quantity']; // 插入物品信息到数据库 $sql = "INSERT INTO inventory (name, description, quantity) VALUES ('$name', '$description', $quantity)"; if (mysqli_query($conn, $sql)) { echo "物品添加成功!"; } else { echo "物品添加失败:" . mysqli_error($conn); } mysqli_close($conn); ?>
4. View inventory
In the website, we provide the user with Provides a function to view inventory, allowing users to browse item information currently in inventory. For example, we can create a file named "view_inventory.php" to display inventory information, as shown below:
<?php include "config.php"; // 查询库存信息 $sql = "SELECT * FROM inventory"; $result = mysqli_query($conn, $sql); if (mysqli_num_rows($result) > 0) { while ($row = mysqli_fetch_assoc($result)) { echo "物品ID: " . $row['id'] . "<br>"; echo "名称: " . $row['name'] . "<br>"; echo "描述: " . $row['description'] . "<br>"; echo "库存数量: " . $row['quantity'] . "<br><br>"; } } else { echo "库存为空!"; } mysqli_close($conn); ?>
5. Update inventory
In the website, we also need to provide a Function allows users to update inventory information. For example, we can create a file called "update_inventory.php" to handle requests to update inventory, as follows:
<?php include "config.php"; $id = $_GET['id']; $quantity = $_GET['quantity']; // 更新库存信息 $sql = "UPDATE inventory SET quantity = $quantity WHERE id = $id"; if (mysqli_query($conn, $sql)) { echo "库存更新成功!"; } else { echo "库存更新失败:" . mysqli_error($conn); } mysqli_close($conn); ?>
6. Delete items
Finally, we need to provide a function Let the user delete items from the inventory. For example, we can create a file named "delete_item.php" to handle requests to delete items as follows:
<?php include "config.php"; $id = $_GET['id']; // 删除物品 $sql = "DELETE FROM inventory WHERE id = $id"; if (mysqli_query($conn, $sql)) { echo "物品删除成功!"; } else { echo "物品删除失败:" . mysqli_error($conn); } mysqli_close($conn); ?>
The above is a brief example of the inventory management functionality provided by our second-hand recycling website developed in PHP . Through these functions, users can easily add, view, update and delete item information in the inventory, improving the functionality and user experience of second-hand recycling websites. Of course, in actual development, we can also optimize and expand the code according to actual needs to provide more rich functions.
The above is the detailed content of Second-hand recycling website developed in PHP provides inventory management functions. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
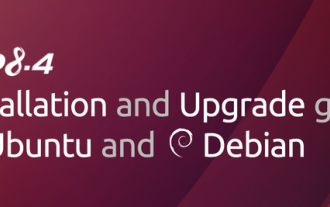
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
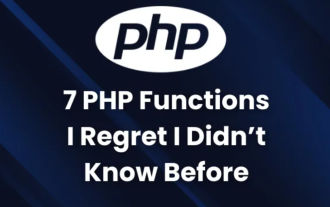
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
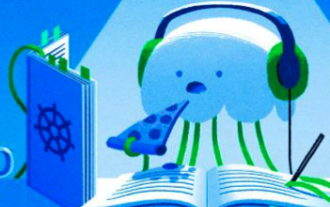
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
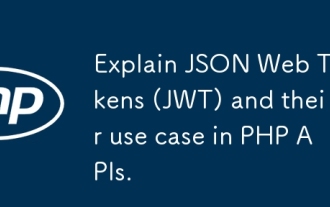
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
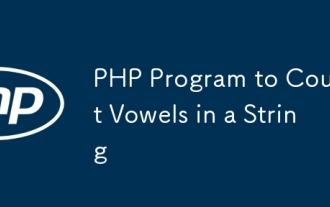
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
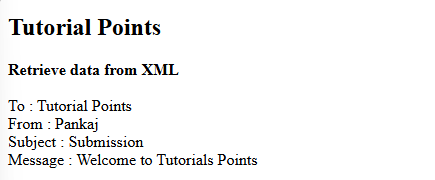
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
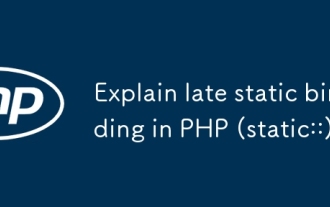
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
