Solution to date formatting in Java development
How to solve the date formatting problem in Java development
In Java development, date formatting is a common and important problem. Different date formatting requirements, such as converting dates to strings and converting strings to dates, are crucial to the functional implementation and user experience of the system. This article will introduce how to solve date formatting problems in Java development and provide some common tips and suggestions.
1. Use the SimpleDateFormat class
Java provides the SimpleDateFormat class for date formatting and parsing. This class is very flexible and can meet most date formatting needs. The following is a simple sample code:
// 日期转换成字符串 SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); String dateString = sdf.format(new Date()); // 字符串转换成日期 SimpleDateFormat sdf2 = new SimpleDateFormat("yyyy-MM-dd"); Date date = sdf2.parse("2022-01-01");
When using SimpleDateFormat, you need to pay attention to the following points:
- SimpleDateFormat is thread-unsafe. In order to avoid problems in multi-threaded environments , you can use ThreadLocal to ensure that each thread holds its own SimpleDateFormat object.
- The letters in the format string have special meanings, such as "yyyy" represents the year, "MM" represents the month, "dd" represents the number of days, etc. For the specific meaning of formatting characters, please refer to the official Java documentation.
- SimpleDateFormat can automatically parse date strings in different formats, but it must be ensured that the given date string has the same format as the formatted string, otherwise a ParseException will be thrown.
2. Use the DateTimeFormatter class
After Java 8, a new date and time API was introduced, including the DateTimeFormatter class. The DateTimeFormatter class is more powerful and safer than the SimpleDateFormat class and is recommended for use in new projects. The following is a sample code:
import java.time.LocalDate; import java.time.format.DateTimeFormatter; // 日期转换成字符串 DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd"); String dateString = LocalDate.now().format(formatter); // 字符串转换成日期 DateTimeFormatter formatter2 = DateTimeFormatter.ofPattern("yyyy-MM-dd"); LocalDate date = LocalDate.parse("2022-01-01", formatter2);
DateTimeFormatter is used similarly to SimpleDateFormat, but has the following advantages:
- DateTimeFormatter is thread-safe and can be used directly in a multi-threaded environment , no additional thread synchronization operations are required.
- DateTimeFormatter supports more date formatting options, such as it can parse date strings with time zones, and can easily perform date addition and subtraction operations.
3. Dealing with time zone issues
When formatting dates, time zone is a very important issue. If no time zone is explicitly specified, the system defaults to the local time zone. In order to avoid problems caused by time zones, you can explicitly specify the time zone before formatting or parsing the date, as shown below:
// 日期转换成字符串 SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd"); sdf.setTimeZone(TimeZone.getTimeZone("GMT+8")); String dateString = sdf.format(new Date()); // 字符串转换成日期 SimpleDateFormat sdf2 = new SimpleDateFormat("yyyy-MM-dd"); sdf2.setTimeZone(TimeZone.getTimeZone("GMT+8")); Date date = sdf2.parse("2022-01-01");
DateTimeFormatter also supports time zone settings, and you can use the withZone method to specify the time zone.
4. Avoid using outdated APIs
During the development process, try to avoid using outdated date and time APIs, such as Date, Calendar, etc. These APIs have some design problems and are not flexible and secure enough. It is recommended to use the new date and time API, such as the classes under the java.time package, to format and parse dates.
Summary
Date formatting is a common and important problem in Java development. By rationally choosing the appropriate date and time class and formatting tool, date formatting and parsing can be easily achieved. When formatting dates, pay attention to details such as thread safety, time zone issues, and avoiding using outdated APIs. I hope that the introduction in this article can help readers solve date formatting problems in Java development.
The above is the detailed content of Solution to date formatting in Java development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
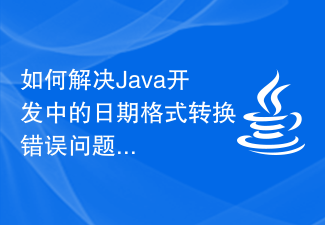
How to solve date format conversion errors in Java development Summary: In the Java development process, date format conversion issues are often involved. However, in different scenarios, you may encounter different date format conversion errors. This article will introduce some common date format conversion errors and provide solutions and sample code. Problem Description In Java development, date format conversion errors may occur in the following aspects: 1.1 Conversion of string to date object: When converting from string to date object, you may encounter

Java Development: How to Optimize Your Code Performance In daily software development, we often encounter situations where we need to optimize code performance. Optimizing code performance can not only improve program execution efficiency, but also reduce resource consumption and improve user experience. This article will introduce some common optimization techniques, combined with specific code examples, to help readers better understand and apply them. Use the right data structures Choosing the right data structures is key to improving the performance of your code. Different data structures have different advantages and disadvantages in different scenarios. For example, Arra
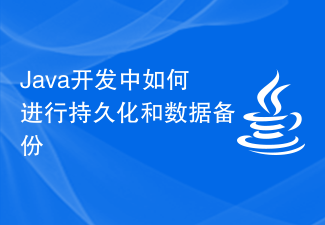
Java is a popular programming language used for the development of various applications. In Java development, data persistence and backup are very important, they can ensure the security and reliability of data. This article will introduce how to perform persistence and data backup in Java development, and provide some specific code examples. First, let us understand what persistence is. Persistence refers to saving data from memory to persistent storage (such as a hard disk or a database) so that the data can be reloaded and restored to its previous state after the program exits. exist
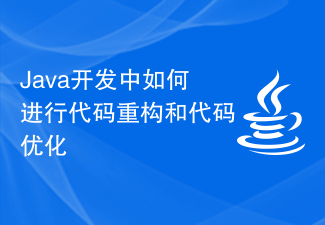
How to perform code refactoring and code optimization in Java development Introduction: In software development, code quality is one of the key factors affecting the success or failure of the project. Good code should have characteristics such as readability, maintainability, and scalability, and code refactoring and code optimization are important means to ensure code quality. This article will explore how to perform code refactoring and code optimization in Java development, and provide some specific code examples. 1. Code Refactoring Code refactoring refers to making the code easier to read by adjusting the code structure and organizational form without changing the code function.
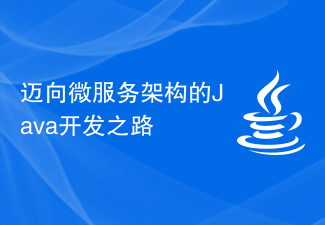
The road to Java development towards a microservices architecture requires concrete code examples In recent years, microservices architecture has become increasingly popular in the field of software development. Compared with the traditional monolithic application architecture, the microservice architecture splits the application into a set of small and independent services. Each service runs in its own process and is interconnected through a lightweight communication mechanism. This architecture enables development teams to develop, test, and deploy applications more flexibly and quickly, and to better adapt to changing business needs. In the field of Java development, the implementation of microservice architecture
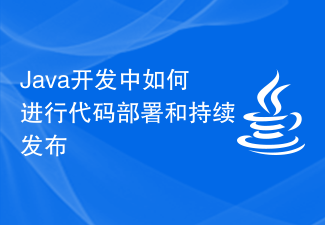
How to perform code deployment and continuous release in Java development Summary: In Java development, code deployment and release are very important links. This article will introduce some common methods and tools for code deployment and continuous release, and give specific code examples. 1. Code deployment Code deployment refers to deploying the developed code and related resource files to the server so that the application can run normally on the server. Here are some common code deployment methods: Manual deployment: This is the most basic deployment method. Developers manually compile
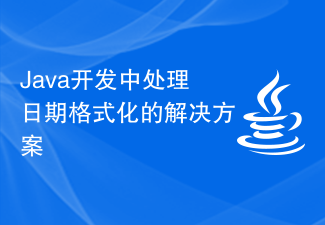
How to solve date formatting problems in Java development In Java development, date formatting is a common and important problem. Different date formatting requirements, such as converting dates to strings and converting strings to dates, are crucial to the functional implementation and user experience of the system. This article will introduce how to solve date formatting problems in Java development and provide some common tips and suggestions. 1. Use the SimpleDateFormat class Java provides SimpleDateForma
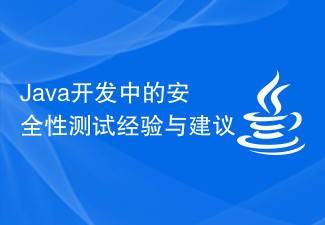
In today's Internet era, software development has become one of the core competitiveness of various industries. As a widely used programming language, Java's development and application scope are also expanding day by day. However, as software grows in size and complexity, software security issues become increasingly prominent. Therefore, security testing in Java development is particularly important. First, we need to understand what security testing is. Security testing is the process of detecting and evaluating security vulnerabilities and risks in software systems by simulating attacks. The purpose is to find
