Solution to Java date problem
How to solve the date processing problem in Java development
Date processing is an important problem often encountered in Java development. In the actual development process, we often need to perform date comparison, format conversion, calculation and other operations. There are also quite a lot of details and pitfalls involved in date processing. Here are some common date processing problems and their solutions.
1. Date formatting
In Java, date formatting mainly relies on the SimpleDateFormat class. However, when formatting dates, some problems often arise, such as thread safety, month format, timestamp, etc. In order to avoid these problems, we can use Joda-Time or the new date and time API provided by Java 8.
- Using Joda-Time
Joda-Time is a popular date and time processing library that provides easy-to-use and thread-safe date processing functions. Using Joda-Time, we can easily perform date formatting, comparison, calculation and other operations.
Sample code:
DateTimeFormatter formatter = DateTimeFormat.forPattern("yyyy-MM-dd HH:mm:ss"); DateTime dateTime = formatter.parseDateTime("2021-01-01 12:00:00"); String formattedDate = dateTime.toString("yyyy/MM/dd"); System.out.println(formattedDate);
- Using Java 8’s date and time API
Java 8 introduces a new date and time API, providing a new set of Date and time processing class. The new API provides thread-safe date and time classes, as well as simpler and easier-to-use methods.
Sample code:
DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"); LocalDateTime dateTime = LocalDateTime.parse("2021-01-01 12:00:00", formatter); String formattedDate = dateTime.format(DateTimeFormatter.ofPattern("yyyy/MM/dd")); System.out.println(formattedDate);
2. Date comparison and calculation
In Java, date comparison and calculation are relatively common operations. For example, judging the size of two dates, calculating the number of days and hours between two dates, etc. When comparing and calculating dates, we need to pay attention to the following points:
- Use the getTime method of the Date class
In Java, the Date class provides the getTime method, Dates can be converted to milliseconds to facilitate comparisons and calculations.
Sample code:
Date date1 = new SimpleDateFormat("yyyy-MM-dd").parse("2021-01-01"); Date date2 = new SimpleDateFormat("yyyy-MM-dd").parse("2022-01-01"); long difference = date2.getTime() - date1.getTime(); long days = difference / (1000 * 60 * 60 * 24); System.out.println(days);
- Using Joda-Time or Java 8’s Date Time API
Joda-Time and Java 8’s Date Time API are provided A series of methods are provided to facilitate date comparison and calculation. These operations can be accomplished using the Period, Duration, and ChronoUnit classes.
Sample code:
DateTime dateTime1 = new DateTime(2021, 1, 1, 0, 0, 0); DateTime dateTime2 = new DateTime(2022, 1, 1, 0, 0, 0); Period period = new Period(dateTime1, dateTime2); int years = period.getYears(); int days = period.getDays(); System.out.println(years + " years, " + days + " days"); // Java 8 LocalDate date1 = LocalDate.of(2021, 1, 1); LocalDate date2 = LocalDate.of(2022, 1, 1); Period period = Period.between(date1, date2); long days = ChronoUnit.DAYS.between(date1, date2); System.out.println(period.getYears() + " years, " + days + " days");
3. Dealing with time zone issues
When processing dates across time zones, we need to pay attention to time zone issues. Java provides the java.util.TimeZone class and the java.time.ZoneId class to handle time zone issues.
Sample code:
Date date = new Date(); SimpleDateFormat sdf = new SimpleDateFormat("yyyy-MM-dd HH:mm:ss"); sdf.setTimeZone(TimeZone.getTimeZone("GMT+8")); String formattedDate = sdf.format(date); System.out.println(formattedDate); // Java 8 LocalDateTime dateTime = LocalDateTime.now(); DateTimeFormatter formatter = DateTimeFormatter.ofPattern("yyyy-MM-dd HH:mm:ss"); ZonedDateTime zonedDateTime = dateTime.atZone(ZoneId.of("Asia/Shanghai")); String formattedDate = zonedDateTime.format(formatter); System.out.println(formattedDate);
The above are some common methods to solve date processing problems in Java development. By rationally using date processing tool classes and APIs, the development process can be simplified and efficiency improved. In actual development, we need to choose the appropriate method according to specific needs and fully understand the use of relevant APIs.
The above is the detailed content of Solution to Java date problem. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
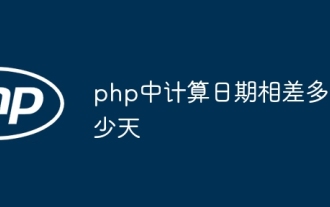
How to calculate the number of days between dates in PHP: Use the date_diff() function to obtain the DateInterval object. Extract the days property in the diff array from the DateInterval object. This property contains the number of days between two dates.
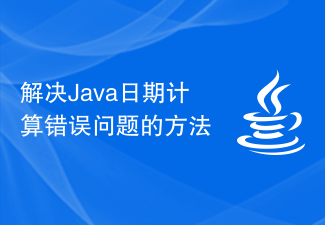
How to solve date calculation errors in Java development Date calculation is a common requirement in Java development, but some errors may occur when dealing with date calculations. This article will introduce some common date calculation errors and their solutions to help Java developers handle date calculations correctly. Problem 1: Incorrect calculation results occur when adding dates. In Java, you can use the Calendar or Date class to perform date addition and subtraction operations. But when using these classes for date addition, an error may occur
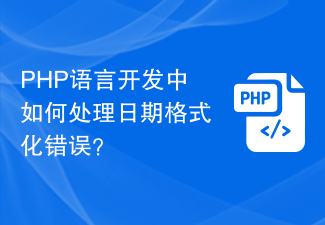
In PHP language development, date formatting errors are a common problem. The correct date format is very important to programmers because it determines the readability, maintainability and correctness of the code. This article will share some tips for dealing with date formatting errors. Understanding date formats Before dealing with date formatting errors, we must first understand date formats. A date format is a string of various letters and symbols used to represent a specific date and time format. In PHP, common date formats include: Y: four-digit year (such as 20
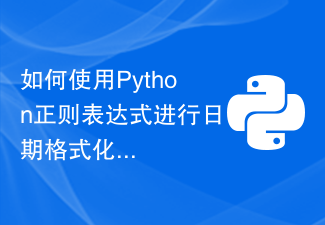
Python regular expression is a very powerful text processing tool that can perform operations such as matching, replacing, and extracting strings. In actual development, we often need to format dates, such as converting "2022/10/01" into the format of "October 01, 2022". This article will introduce how to use Python regular expressions for date formatting. 1. Overview of Python regular expressions Python regular expression is a special string pattern, which describes a series of characters that match a certain
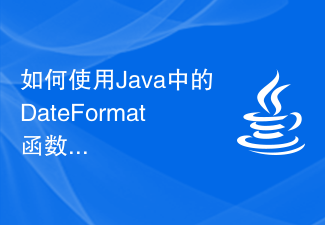
Java is a popular programming language that includes many powerful tools for date and time manipulation. One of the most commonly used tools is the DateFormat function, which can format date data into a specific string format. This article will introduce how to use the DateFormat function in Java for date formatting. Import the Date and DateFormat classes. Before starting to use the DateFormat function, we need to import the Date and DateFormat classes in Java.
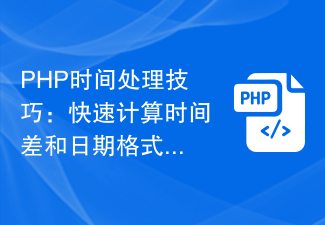
PHP Time Processing Tips: Quickly Calculate Time Difference and Date Formatting With the rapid development of the Internet, time processing has become one of the common tasks in web development. In PHP, time processing is a relatively common requirement, such as calculating time differences, formatting dates, and other operations. This article will introduce some PHP time processing techniques, including quickly calculating time differences and date formatting, and come with some specific code examples. Calculating the time difference In many application scenarios, we need to calculate the time difference between two time points, such as calculating the time difference between two time points.
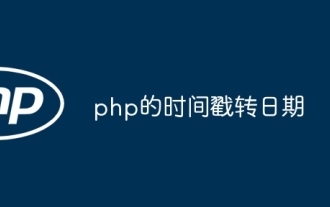
To convert a PHP timestamp to a date, you can use the date() function, the syntax is: date(format, timestamp). Common date format specifiers include: Y (year), m (month), d (day), H (hour), i (minute), and s (second). The code example to convert timestamp 1658096324 to date is: $timestamp=1658096324;$date=date('Y-m-dH:i:s',$timestamp); Output: 2022-07-1914:58:44.
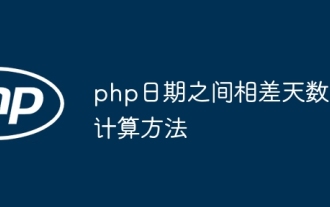
There are two ways to calculate date differences in PHP: 1. Using the DateInterval class, 2. Using the strtotime and date_diff functions. The specific implementation methods are: 1. Use the diff method to obtain the difference between two dates in the DateInterval object, and then obtain the difference in days; 2. Convert the date into a timestamp, use the date_diff function to obtain the date difference, and then convert the difference in days into an integer.
