


PHP multi-threaded programming example: creating concurrent tasks for anomaly detection
PHP multi-threaded programming example: creating concurrent tasks for anomaly detection
With the continuous development of web applications, PHP, as a popular server-side scripting language, has become more and more important. Developers need a way to improve PHP's performance and efficiency when dealing with large-scale and concurrent requests. One solution is to use multi-threaded programming techniques.
In this article, we will explore how to use PHP for multi-threaded programming and how to use multi-threading for exception detection of concurrent tasks. We will illustrate this process through an example.
Before we begin, we need to ensure that the PHP pthreads extension is installed on the server. After the installation is complete, we can start writing our code.
First, we will create a class named Task, which represents a concurrent task. Each task will contain a method that performs some action. Let's look at the specific code:
class Task extends Thread { public function __construct($id) { $this->id = $id; } public function run() { // 执行任务操作 $this->performTask(); } private function performTask() { // 这里写入具体任务的代码 // 例如,异常检测逻辑 try { // 执行某些操作 // .. } catch (Exception $e) { // 处理异常情况 // .. } } }
In the above code, we created a Task class, which inherits from the Thread class. In the class constructor, we pass in a unique task ID. In the run method, we will call the performTask method to perform the task operation.
In the performTask method, we can perform any operation, such as anomaly detection. Suppose we have a database connection and we need to execute some queries concurrently to check for anomalies in the database. We can perform corresponding query operations in the performTask method and handle exceptions based on the results.
Next, we need to create a class called ThreadManager, which is responsible for managing the creation and execution of multiple concurrent tasks:
class ThreadManager { public function __construct($numThreads) { $this->numThreads = $numThreads; } public function startThreads() { $threads = []; for ($i = 1; $i <= $this->numThreads; $i++) { $thread = new Task($i); $thread->start(); $threads[] = $thread; } // 等待所有线程完成执行 foreach ($threads as $thread) { $thread->join(); } } }
In the above code, we create a ThreadManager Class, this class accepts a parameter numThreads, indicating the number of concurrent tasks to be created.
In the startThreads method, we use a loop to create a specified number of Task objects (i.e. concurrent tasks) and add them to an array. Then, we call the start method of each task to start the execution of the task.
Next, we use a foreach loop to wait for all threads to complete execution by calling the join method of each task. In this way, we ensure that all tasks are completed.
Now, we can instantiate the ThreadManager class in the main program and call the startThreads method to start all concurrent tasks:
$numThreads = 5; // 并发任务数量 $manager = new ThreadManager($numThreads); $manager->startThreads();
Through the above steps, we have successfully created concurrent tasks to handle exceptions detection.
Summary:
This article introduces how to use PHP for multi-threaded programming and how to use multi-threads to detect exceptions in concurrent tasks. We created a class named Task, which represents a concurrent task, and wrote code in it to specifically perform task operations. Then, we create a ThreadManager class that is responsible for managing the creation and execution of multiple concurrent tasks. By instantiating the ThreadManager class and calling the startThreads method, we can create concurrent tasks and start their execution. This multi-threaded programming technology can effectively improve the performance and efficiency of PHP, especially when handling large-scale and concurrent requests.
The above is the detailed content of PHP multi-threaded programming example: creating concurrent tasks for anomaly detection. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
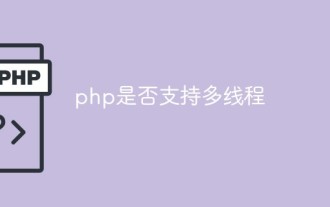
PHP does not support multi-threading. The reason is: PHP does not support multi-threading by default. To use multi-threading, you need to install the pthread extension. To install the pthread extension, you must use the --enable-maintainer-zts parameter to recompile PHP.
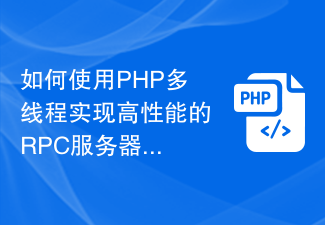
How to use PHP multi-threading to implement a high-performance RPC server. With the continuous development of the Internet, there are more and more demands for distributed systems. Remote Procedure Call (RPC) is one of the communication mechanisms often used in these distributed systems. It allows programs on different machines to call remote functions just like calling local functions, thereby realizing data transmission and function calls between systems. In actual development, in order to improve the performance and concurrent processing capabilities of the system, multi-threading technology is used to
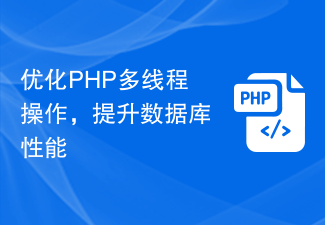
How to improve database read and write performance through PHP multi-threading. With the rapid development of the Internet, database read and write performance has become a key issue. When our application needs to frequently read and write to the database, using a single-threaded approach often leads to performance bottlenecks. The use of multi-threading can improve the efficiency of database reading and writing, thereby improving overall performance. As a commonly used server-side scripting language, PHP has flexible syntax and powerful database operation capabilities. This article will introduce how to use PHP multi-threading technology to improve
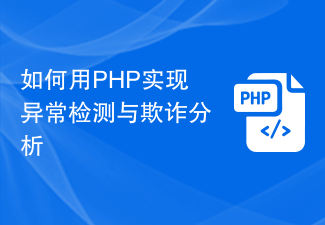
How to use PHP to implement anomaly detection and fraud analysis Abstract: With the development of e-commerce, fraud has become a problem that cannot be ignored. This article introduces how to use PHP to implement anomaly detection and fraud analysis. By collecting user transaction data and behavioral data, combined with machine learning algorithms, user behavior is monitored and analyzed in real time in the system, potential fraud is identified, and corresponding measures are taken to deal with it. Keywords: PHP, anomaly detection, fraud analysis, machine learning 1. Introduction With the rapid development of e-commerce, the number of transactions people conduct on the Internet
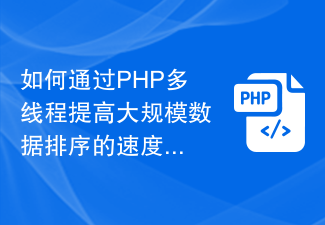
How to improve the speed of large-scale data sorting through PHP multi-threading. With the rapid development of the Internet and the popularity of big data, the demand for processing massive data is also increasing. Among them, for the common problem of data sorting, how to improve the processing speed has become an urgent problem to be solved. In the field of PHP, multi-threading technology is considered an effective solution. This article will introduce how to improve the speed of large-scale data sorting through PHP multi-threading. 1. The principle of multi-threading Multi-threading refers to the existence of multiple threads at the same time. Multiple threads can execute different tasks at the same time.
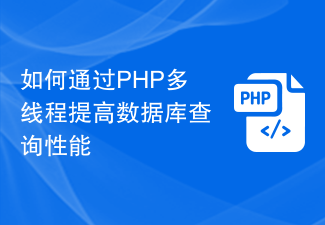
How to improve database query performance through PHP multi-threading Introduction: With the rapid development of the Internet, database query performance has become one of the important challenges faced by developers. As a widely used server-side scripting language, PHP also plays an important role in database queries. This article will explore how to improve database query performance through PHP multi-threading technology to meet the needs of high concurrent requests. 1. What is multi-threading? Before discussing how to use multi-threading to improve database query performance, we first need to understand what multi-threading is. popular
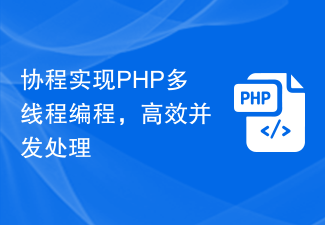
PHP multi-threaded programming practice: using coroutines to implement concurrent task processing. With the development of Internet applications, the requirements for server performance and concurrent processing capabilities are becoming higher and higher. Traditional multi-threaded programming is not easy to implement in PHP, so in order to improve PHP's concurrent processing capabilities, you can try to use coroutines to implement multi-threaded programming. Coroutine is a lightweight concurrency processing model that can implement concurrent execution of multiple tasks in a single thread. Compared with traditional multi-threading, coroutine switching costs are lower
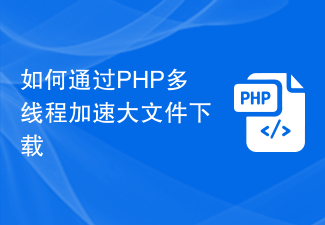
How to accelerate large file downloads through PHP multi-threading In today's Internet era, file transfer has become more and more common and important. However, for larger files, the download time increases significantly, causing inconvenience to users. In order to improve the download speed of large files, we can achieve acceleration through PHP multi-threading. This article will introduce how to speed up large file downloads through PHP multi-threading. First, in order to implement PHP multi-threaded downloading, we need to do some preparations. Make sure the latest version of PHP is installed on the server and enabled
