How to do parallel sorting using Arrays.parallelSort function in Java
With the development of computer hardware, we can now use multi-core CPUs to process data more efficiently. In Java, we can use the parallelSort function in the Arrays class to perform parallel sorting to speed up the data sorting process.
First, let's take a look at how to use the Arrays.sort function for single-threaded sorting. Here is a simple example that demonstrates how to sort an array of integers:
import java.util.Arrays; public class SingleThreadSortExample { public static void main(String[] args) { int[] numbers = { 5, 3, 6, 1, 9, 4 }; Arrays.sort(numbers); // 使用 Arrays.sort 函数进行排序 for (int num : numbers) { System.out.print(num + " "); } } }
The output is: 1 3 4 5 6 9
In this example, We used the Arrays.sort function to sort an array of integers. This is a single-threaded call that does all the sorting work in one thread.
However, using the parallelSort function, we can divide the sorting process into multiple threads for parallel execution. This will greatly improve sorting efficiency. The following is sample code for parallel sorting using the Arrays.parallelSort function:
import java.util.Arrays; public class ParallelSortExample { public static void main(String[] args) { int[] numbers = { 5, 3, 6, 1, 9, 4 }; Arrays.parallelSort(numbers); // 使用 Arrays.parallelSort 函数进行排序 for (int num : numbers) { System.out.print(num + " "); } } }
The output result is the same as single-threaded sorting: 1 3 4 5 6 9
. However, on devices with multi-core CPUs, the parallelSort function will be faster than single-threaded sorting. This example is just a simple demonstration. In fact, the larger the amount of data, the greater the advantage of using parallelSort for parallel sorting.
If you need to sort the object array, you can also use the parallelSort function, but you need to specify a custom Comparator for sorting. The following is an example of parallel sorting of an array of strings:
import java.util.Arrays; import java.util.Comparator; public class ParallelSortWithComparatorExample { public static void main(String[] args) { String[] words = { "banana", "apple", "pear", "orange" }; Arrays.parallelSort(words, new Comparator<String>() { public int compare(String s1, String s2) { return s1.compareTo(s2); } }); for (String word : words) { System.out.print(word + " "); } } }
The output is: apple banana orange pear
In this example, we use Arrays. The parallelSort method sorts an array of strings. Unlike single-threaded sorting, we need to pass a custom Comparator to the sorting function to specify the sorting rules. In this example, we use an anonymous inner class to create a custom Comparator that sorts elements alphabetically.
It can be seen that using the parallelSort function can help you sort the data faster. However, it is worth noting that single-threaded sorting may be faster with small data volumes. Therefore, when using the parallelSort function, you need to choose the sorting method that best suits you according to the actual situation.
The above is the detailed content of How to do parallel sorting using Arrays.parallelSort function in Java. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










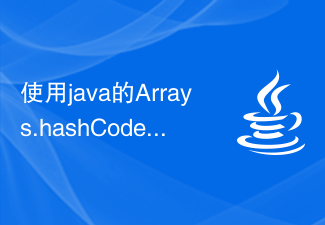
Use Java's Arrays.hashCode() function to calculate the hash code of an array. A hash code (HashCode) is an integer value that uniquely identifies an object. In Java, array is a common data structure. In order to facilitate comparison and indexing of arrays, we often need to calculate the hash code of the array. Java provides the hashCode() function of the Arrays class, which can quickly calculate the hash code of an array. The Arrays.hashCode() method is a static method that accepts
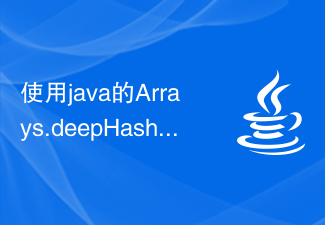
Use Java's Arrays.deepHashCode() function to calculate the hash code of a multi-dimensional array. In Java programming, we often need to compare whether two objects are equal. When multidimensional arrays are involved, comparing the contents of array objects can get a little trickier. In this case, we can use hashcode to compare the contents of the array. A hash code is a unique integer value that identifies an object. In Java we can use Arrays.deepHashCod

How does the Arrays.copyOf() method in Java copy an array into a new array? In Java, array is a very common data structure, and Java's Arrays class provides us with many convenient methods to deal with arrays. Among them, the Arrays.copyOf() method is a very useful method for copying an array to a new array. This article will introduce the use and implementation principle of this method, and provide specific code examples. UsageArrays
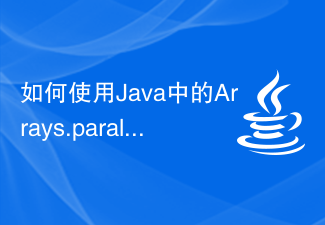
With the development of computer hardware, we can now use multi-core CPUs to process data more efficiently. In Java, we can use the parallelSort function in the Arrays class to perform parallel sorting to speed up the data sorting process. First, let's take a look at how to use the Arrays.sort function for single-threaded sorting. Here is a simple example showing how to sort an array of integers: importjava.util.Arrays;publi
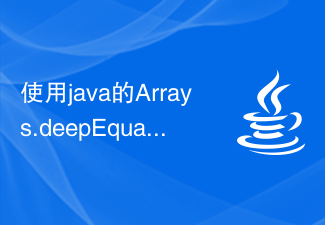
Use java's Arrays.deepEquals() function to compare whether multi-dimensional arrays are equal. In Java, if we need to compare whether two multi-dimensional arrays are equal, we can use the deepEquals() function in the java.util.Arrays class. This function compares each element in a multidimensional array to determine whether two arrays are equal. In this article, we will introduce the method of using the Arrays.deepEquals() function to compare multi-dimensional arrays for equality.
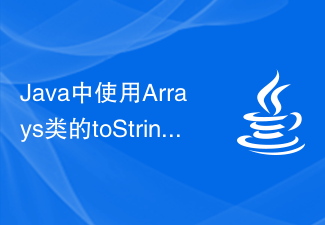
Use the toString() method of the Arrays class in Java to convert an array into a string. In Java programming, you often encounter situations where you need to convert an array into a string. Java provides the toString() method of the Arrays class, making this process very simple and convenient. This article will introduce how to use the toString() method of the Arrays class to convert an array into a string, and give corresponding code examples. First, we need to understand the toSt of the Arrays class
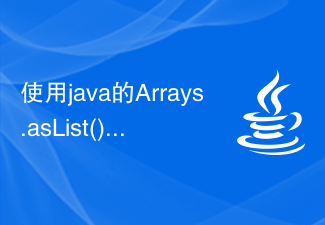
Use java's Arrays.asList() function to convert an array to a List. In Java programming, you often encounter the situation of converting an array to a List. To achieve this conversion, you can use the asList() function in the java.util.Arrays class. This function can convert an array into a List to facilitate the operation and processing of the array. The following is sample code to convert an array to a List using the Arrays.asList() function: import
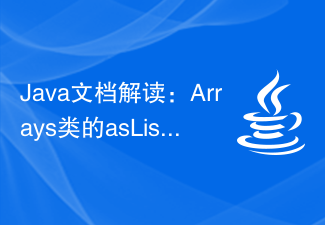
In Java applications, it is often necessary to operate and convert arrays. The Arrays class provides many convenient methods, one of which is the asList() method. This article will explain the meaning and usage of the asList() method in detail, and will also provide some code examples to help readers better understand this method. Overview of the asList() method The asList() method is a static method that returns a List object that contains all the elements in the specified array. The syntax of this method is as follows: p
