How to implement the XML-RPC protocol using PHP and SimpleXML
XML-RPC is a remote procedure call protocol based on XML format that allows different applications to interact with each other on the Internet. PHP and SimpleXML are two very suitable tools for implementing the XML-RPC protocol. This article describes how to implement the XML-RPC protocol using PHP and SimpleXML.
Step 1: Understand the XML-RPC protocol
The XML-RPC protocol specifies the format and standards for data exchange between the client and the server. It is a remote procedure call-based protocol that uses HTTP as the transport protocol and XML as the data exchange format.
In the XML-RPC protocol, the client and server interact through XML documents. The client builds a request XML document, and the server receives the request and returns a response XML document. The XML-RPC protocol itself does not contain data type definitions, and all data types are implemented in XML.
Step 2: Install and configure PHP and SimpleXML
PHP is a powerful programming language, especially suitable for web development. Before using PHP to implement the XML-RPC protocol, we need to install and configure the PHP development environment.
SimpleXML is an extension library provided by PHP for parsing XML documents. We also need to install and configure the SimpleXML extension library in PHP.
Step 3: Create XML-RPC server
Creating an XML-RPC server in PHP is very simple. You only need to use PHP's built-in xmlrpc_server_register_method() function to register the method. Here is a simple XML-RPC server sample code:
<?php function add($params) { $sum = 0; foreach ($params as $param) { $sum += $param; } return $sum; } $server = xmlrpc_server_create(); xmlrpc_server_register_method($server, "add", "add"); $request_xml = file_get_contents("php://input"); $response_xml = xmlrpc_server_call_method($server, $request_xml, null); header("Content-Type: text/xml"); echo $response_xml; ?>
In the above code, we have created an add() method to handle XML-RPC requests. This method accepts an array parameter and returns the result after adding all parameters. We use the xmlrpc_server_create() function to create an XML-RPC server, and then use the xmlrpc_server_register_method() function to register the add() method to the XML-RPC server. Finally, we get the request XML document from the input stream and use the xmlrpc_server_call_method() method to process the XML-RPC request and return the response XML document.
Step 4: Call the XML-RPC server
Calling the XML-RPC server in PHP is also very simple. You only need to use the xmlrpc_encode_request() and xmlrpc_decode() functions provided by PHP. The following is a simple XML-RPC client sample code:
<?php $request = xmlrpc_encode_request("add", array(1, 2, 3, 4, 5)); $context = stream_context_create(array( "http" => array( "method" => "POST", "header" => "Content-Type: text/xml", "content" => $request ) )); $server_url = "http://example.com/xmlrpc-server.php"; $response_xml = file_get_contents($server_url, false, $context); $response = xmlrpc_decode($response_xml); echo $response; ?>
In the above code, we use the xmlrpc_encode_request() function to construct an XML-RPC request with the add() method and send it to the remote XML -RPC server. We send an HTTP POST request using PHP's built-in file_get_contents() function and insert the request XML document into the HTTP request body. Finally, we decode the return value from the XML-RPC response returned by the server and output it.
Conclusion
By studying this article, you can master how to use PHP and SimpleXML to implement the XML-RPC protocol. You can create an XML-RPC server that provides an interface to perform certain operations and send XML-RPC requests from clients and use the response data for your application. (targetEntity)
The above is the detailed content of How to implement the XML-RPC protocol using PHP and SimpleXML. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
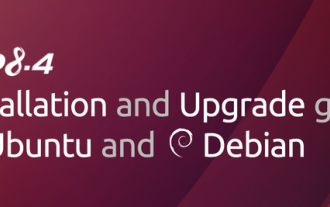
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
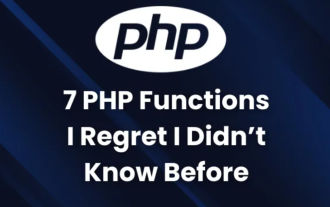
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
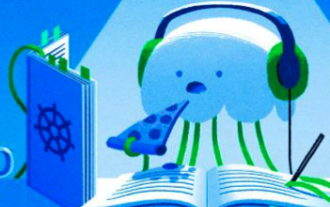
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
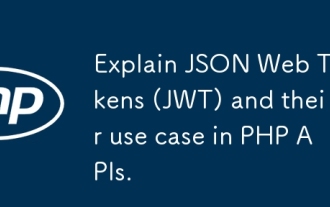
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
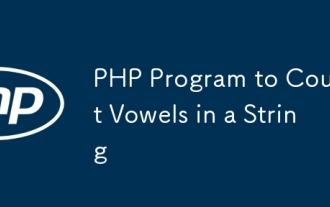
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
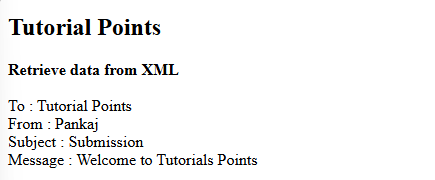
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
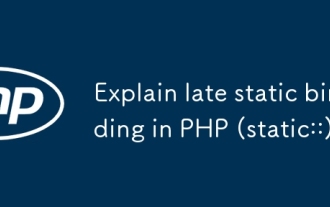
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
