


How to verify the domain name of an email address using regular expressions in golang
It is necessary to use regular expressions to verify the domain name of email addresses in golang, because email addresses are very common in practical applications, and the rules for email addresses are also relatively complex. In many cases, we need to verify whether the domain name of the email address entered by the user meets the specifications to ensure the validity and security of the data.
This article will introduce how to use regular expressions to verify the domain name of an email address in golang. First, we need to understand the basic rules of email addresses.
The email address consists of two parts: "user name" and "domain name", connected by the "@" symbol in the middle. Among them, the domain name part includes host name, first-level domain name, second-level domain name and other information, and is an important part of the email address.
When verifying the domain name of the email address, we need to pay attention to the following points:
- The domain name consists of multiple words, and the words are connected with "." symbols.
- Domain name must start and end with letters or numbers.
- Special characters cannot be included in the domain name, such as spaces, commas, semicolons, etc.
- The domain name can contain the hyphen "-", but it cannot start or end with a hyphen.
With the basis of these rules, we can use regular expressions to verify the domain name of the email address. In golang, we use "regexp" package to handle regular expressions. The following is a sample code that can verify whether a string is a legal email address domain name:
package main import ( "fmt" "regexp" ) func main() { str := "example.com" reg := regexp.MustCompile(`^[a-zA-Z0-9]([a-zA-Z0-9-]{0,61}[a-zA-Z0-9])?(.[a-zA-Z]{2,})+$`) if !reg.MatchString(str) { fmt.Println("Invalid domain name") } else { fmt.Println("Valid domain name") } }
In the above code, we use a regular expression to verify whether a string is a legal email address domain name . The meaning of this regular expression is as follows:
- ^ means matching the beginning of the string, $ means matching the end of the string.
- [a-zA-Z0-9] means matching letters or numbers, and must start and end with letters or numbers.
- [a-zA-Z0-9-]{0,61} means matching letters, numbers and hyphens, and the length cannot exceed 63 characters.
- (.[a-zA-Z]{2,}) means matching one or more consecutive domain names. Each domain name is connected with a "." symbol, indicating first-level domain name, second-level domain name, etc.
Using this regular expression can meet most email address domain name verification needs. If there are other special circumstances, you can also modify the regular expression to adapt to different needs.
In summary, using regular expressions to verify the domain name of an email address is a simple and efficient way to improve the code quality and security of our applications. It is also very convenient to use regular expressions in golang. You only need to import the "regexp" package.
The above is the detailed content of How to verify the domain name of an email address using regular expressions in golang. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
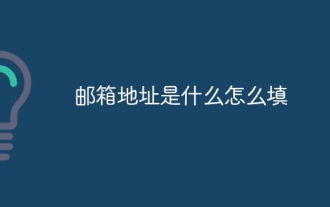
1. The format of the email address is [username@domain name], where the username is the recipient's account and the domain name is the name of the recipient's email server. 2. The example [123456@qq.com] is an email address, where [123456] is the user name and [qq.com] is the domain name. 3. Take another example [888888@163.com], where [888888] is the user name and [163.com] is the domain name.
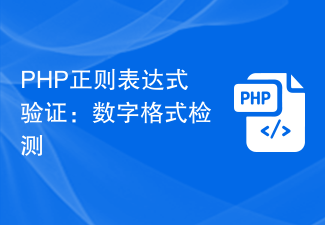
PHP regular expression verification: Number format detection When writing PHP programs, it is often necessary to verify the data entered by the user. One of the common verifications is to check whether the data conforms to the specified number format. In PHP, you can use regular expressions to achieve this kind of validation. This article will introduce how to use PHP regular expressions to verify number formats and provide specific code examples. First, let’s look at common number format validation requirements: Integers: only contain numbers 0-9, can start with a plus or minus sign, and do not contain decimal points. floating point
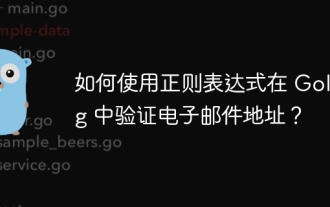
To validate email addresses in Golang using regular expressions, follow these steps: Use regexp.MustCompile to create a regular expression pattern that matches valid email address formats. Use the MatchString function to check whether a string matches a pattern. This pattern covers most valid email address formats, including: Local usernames can contain letters, numbers, and special characters: !.#$%&'*+/=?^_{|}~-`Domain names must contain at least One letter, followed by letters, numbers, or hyphens. The top-level domain (TLD) cannot be longer than 63 characters.
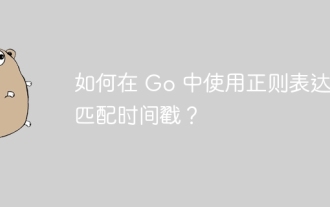
In Go, you can use regular expressions to match timestamps: compile a regular expression string, such as the one used to match ISO8601 timestamps: ^\d{4}-\d{2}-\d{2}T \d{2}:\d{2}:\d{2}(\.\d+)?(Z|[+-][0-9]{2}:[0-9]{2})$ . Use the regexp.MatchString function to check if a string matches a regular expression.
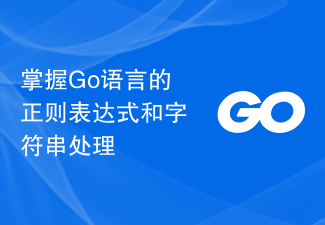
As a modern programming language, Go language provides powerful regular expressions and string processing functions, allowing developers to process string data more efficiently. It is very important for developers to master regular expressions and string processing in Go language. This article will introduce in detail the basic concepts and usage of regular expressions in Go language, and how to use Go language to process strings. 1. Regular expressions Regular expressions are a tool used to describe string patterns. They can easily implement operations such as string matching, search, and replacement.
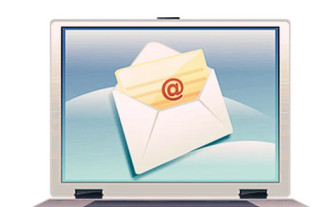
The format of filling in the email address is mainly username+@+website suffix. Analysis 1 The format of filling in the email address is mainly user name + @ + website suffix, such as zhangsan@qq.com. 2 An email address is a mail-receiving address in the online world. Currently, the most widely used email addresses in China include QQ email, Sina email, NetEase email, etc. 3. On the Internet, each user's email address is unique, which ensures the accuracy and uniqueness of sending and receiving emails. Supplement: What does e-mail mean? 1 E-mail refers to a communication method that uses intelligent electronic technology to exchange information. It is the most widely used service on the mobile Internet. Using the Internet e-mail system, users can quickly communicate with all users at a very cheap price. Anywhere in the world
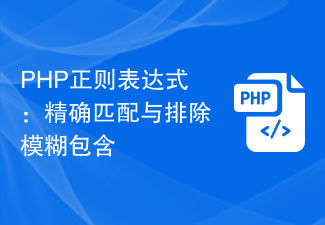
PHP Regular Expressions: Exact Matching and Exclusion Fuzzy inclusion regular expressions are a powerful text matching tool that can help programmers perform efficient search, replacement and filtering when processing text. In PHP, regular expressions are also widely used in string processing and data matching. This article will focus on how to perform exact matching and exclude fuzzy inclusion operations in PHP, and will illustrate it with specific code examples. Exact match Exact match means matching only strings that meet the exact condition, not any variations or extra words.
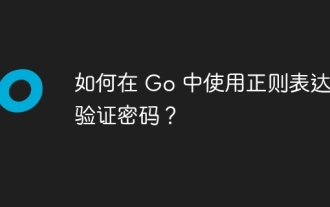
The method of using regular expressions to verify passwords in Go is as follows: Define a regular expression pattern that meets the minimum password requirements: at least 8 characters, including lowercase letters, uppercase letters, numbers, and special characters. Compile regular expression patterns using the MustCompile function from the regexp package. Use the MatchString method to test whether the input string matches a regular expression pattern.
