Code debugging skills in PHP development
In PHP development, debugging is an inevitable step. Especially when faced with complex business logic and code architecture, problems and even bugs are common. Therefore, efficient code debugging skills are very necessary, which can help us quickly locate problems and fix bugs, and release reliable products as early as possible. This article will introduce several common code debugging techniques in PHP development.
1. Use var_dump() and print_r()
Using var_dump() and print_r() is one of the most commonly used debugging methods by PHP developers. These functions can help us print out the values of variables, arrays, objects, etc. so that we can check possible problems in the code.
The var_dump() function can output detailed information such as the type, length and value of the variable, so it is very suitable for debugging code:
$a = array('foo', 'bar', 'baz'); var_dump($a);
The output result is:
array(3) { [0]=> string(3) "foo" [1]=> string(3) "bar" [2]=> string(3) "baz" }
The print_r() function can print variables or arrays into an easy-to-read format, which is usually used to read the values of variables and arrays during debugging:
$a = array('foo', 'bar', 'baz'); print_r($a);
The output result is:
Array ( [0] => foo [1] => bar [2] => baz )
In addition, these two functions can also be nested to print out more information.
2. Use the xdebug plug-in
xdebug is a powerful PHP debugging plug-in that can provide good command line and code coverage functions. When you are debugging code, xdebug allows you to automatically capture the values of variables and functions in your code instead of manually calling the var_dump() or print_r() function, thereby helping you solve problems faster. .
To use the xdebug plug-in, you need to install it first and configure your IDE (such as PhpStorm, Visual Studio Code, etc.). Then put a breakpoint on the line of code you need to debug and start the xdebug plug-in. When the code reaches the breakpoint, xdebug will automatically stop running and wait for debugging and next step processing.
3. Use the error_reporting() function
The error_reporting() function can set the PHP error reporting level, which can help us quickly discover, locate and repair various errors in the PHP code.
During development and debugging, the reporting level can be set to E_ALL so that PHP catches all possible errors:
error_reporting(E_ALL);
When there are errors in the code, PHP will throw the relevant Error messages, which help us quickly find the problem.
4. Use try/catch block
In PHP, try/catch block is a common way to handle exceptions. Using try/catch in code can help us catch and handle errors that occur when the code is running.
For example:
try { // 可能会生成异常的代码 } catch (Exception $e) { // 处理异常的代码 }
In this example, if the code in the try block generates an exception, the code will jump to the catch block for processing. In this way, we can more easily diagnose and handle problems that occur when the code is running.
Conclusion
The above are several debugging techniques commonly used in PHP development. When we use these techniques during the development process, we can quickly locate and solve problems in the code and improve code development efficiency.
The above is the detailed content of Code debugging skills in PHP development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










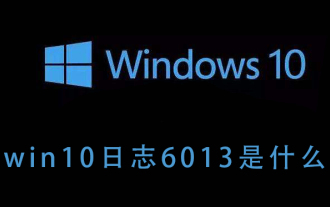
The logs of win10 can help users understand the system usage in detail. Many users must have encountered log 6013 when looking for their own management logs. So what does this code mean? Let’s introduce it below. What is win10 log 6013: 1. This is a normal log. The information in this log does not mean that your computer has been restarted, but it indicates how long the system has been running since the last startup. This log will appear once every day at 12 o'clock sharp. How to check how long the system has been running? You can enter systeminfo in cmd. There is one line in it.
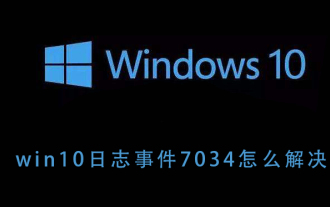
The logs of win10 can help users understand the system usage in detail. Many users must have seen a lot of error logs when looking for their own management logs. So how to solve them? Let’s take a look below. . How to solve win10 log event 7034: 1. Click "Start" to open "Control Panel" 2. Find "Administrative Tools" 3. Click "Services" 4. Find HDZBCommServiceForV2.0, right-click "Stop Service" and change it to "Manual Start "
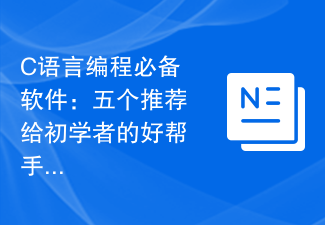
C language is a basic and important programming language. For beginners, it is very important to choose appropriate programming software. There are many different C programming software options on the market, but for beginners, it can be a bit confusing to choose which one is right for you. This article will recommend five C language programming software to beginners to help them get started quickly and improve their programming skills. Dev-C++Dev-C++ is a free and open source integrated development environment (IDE), especially suitable for beginners. It is simple and easy to use, integrating editor,
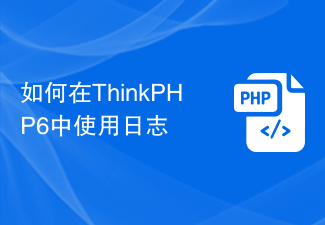
With the rapid development of the Internet and Web applications, log management is becoming more and more important. When developing web applications, how to find and locate problems is a very critical issue. A logging system is a very effective tool that can help us achieve these tasks. ThinkPHP6 provides a powerful logging system that can help application developers better manage and track events that occur in applications. This article will introduce how to use the logging system in ThinkPHP6 and how to utilize the logging system
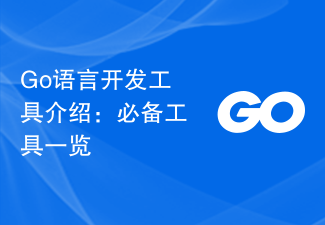
Title: Introduction to Go language development tools: List of essential tools In the development process of Go language, using appropriate development tools can improve development efficiency and code quality. This article will introduce several essential tools commonly used in Go language development, and attach specific code examples to allow readers to understand their usage and functions more intuitively. 1.VisualStudioCodeVisualStudioCode is a lightweight and powerful cross-platform development tool with rich plug-ins and functions.
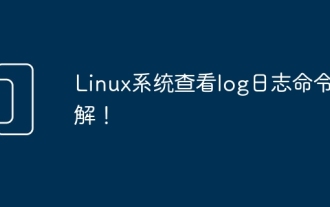
In Linux systems, you can use the following command to view the contents of the log file: tail command: The tail command is used to display the content at the end of the log file. It is a common command to view the latest log information. tail [option] [file name] Commonly used options include: -n: Specify the number of lines to be displayed, the default is 10 lines. -f: Monitor the file content in real time and automatically display the new content when the file is updated. Example: tail-n20logfile.txt#Display the last 20 lines of the logfile.txt file tail-flogfile.txt#Monitor the updated content of the logfile.txt file in real time head command: The head command is used to display the beginning of the log file
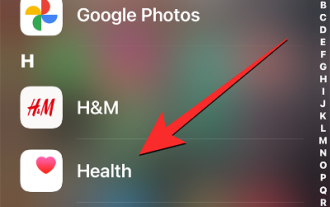
iPhone lets you add medications to the Health app to track and manage the medications, vitamins and supplements you take every day. You can then log medications you've taken or skipped when you receive a notification on your device. After you log your medications, you can see how often you took or skipped them to help you track your health. In this post, we will guide you to view the log history of selected medications in the Health app on iPhone. A short guide on how to view your medication log history in the Health App: Go to the Health App>Browse>Medications>Medications>Select a Medication>Options&a
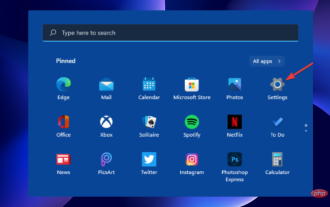
Since Windows 11 is still relatively new and is still expected to feature a lot of improvements, users are bound to deal with a bug or two. One such error is the Breaking Point Reached error message on Windows 11. This error can be due to a number of factors, some of which are known, while others are difficult to determine. Thankfully, these solutions are usually not far-fetched and in some cases only require a system update. Regardless of the cause and complexity, we've collected ways to fix errors in this complete guide. You just need to follow the instructions and you'll be fine. What does the error message "Breakpoint reached" mean? Breakpoint reached is a common error message that Windows 11 users may encounter. This error message usually occurs in
