Implementing RESTful API using ThinkPHP6
With the continuous development of mobile Internet, RESTful API has become an important part of Web development. It is a communication method based on HTTP protocol that can be used to access and operate web resources. In order to better develop RESTful API, we can use the PHP framework ThinkPHP6 to achieve it.
First, we need to establish a basic RESTful API structure. Using the command line tool of ThinkPHP6, you can easily generate a RESTful API application. Open the command line interface, switch to our project directory, and enter the following command:
php think build --name api
where api
is the name of the application we want to create. After executing this command, ThinkPHP6 will create a basic RESTful API application structure for us, including the following directories and files:
api/ ├─ app/ │ ├─ controller/ │ ├─ model/ │ ├─ service/ │ ├─ validate/ │ └─ route.php ├─ config/ │ ├─ app.php │ └─ database.php ├─ public/ │ ├─ index.php │ └─ .htaccess ├─ vendor/ ├─ .env ├─ composer.json └─ README.md
Among them, the app
directory stores our application-related files. The config
directory stores our application configuration files. The public
directory stores our entry files and static resource files. The vendor
directory stores our Composer dependency packages. .env
is our environment configuration file. composer.json
is our Composer configuration file. README.md
is our documentation.
Next, we need to define our API routing rules. In the route.php
file in the app
directory, we can add our API routing rules. For example:
Route::resource('article', 'ArticleController');
The above line of code defines a article
resource route, which means that we can access and operate the Article
resource through this route. This route will automatically generate 7 RESTful API actions, including index
, create
, store
, show
, edit
, update
and destroy
. We can implement these actions in ArticleController
.
<?php namespace appcontroller; use thinkRequest; use appmodelArticle as ArticleModel; class ArticleController { public function index() { $articles = ArticleModel::select(); return json($articles); } public function create() { return 'create'; } public function store(Request $request) { $data = $request->param(); $article = ArticleModel::create($data); return json($article); } public function show($id) { $article = ArticleModel::find($id); return json($article); } public function edit($id) { return 'edit'; } public function update(Request $request, $id) { $data = $request->param(); $article = ArticleModel::update($data, ['id' => $id]); return json($article); } public function destroy($id) { $article = ArticleModel::destroy($id); return json($article); } }
In the above code, we use ArticleModel
to handle data operations related to Article
resources. In the index
action, we get all the Article
data and return it. In the store
action, we save the data obtained through the Request
object into the database. The implementation of other actions is similar.
Finally, we need to set the configuration of our API application in the app.php
file in the config
directory. For example:
return [ 'app_status' => 'api', 'default_return_type' => 'json', 'http_exception_template' => [ 401 => function ($request) { return json(['code' => 401, 'msg' => 'Unauthorized']); }, 404 => function ($request) { return json(['code' => 404, 'msg' => 'Not Found']); }, 500 => function ($request, $exception) { return json(['code' => 500, 'msg' => 'Internal Server Error']); }, ], ];
In the above code, we specify that the response type of our application is JSON. Also defines some HTTP error handling methods.
At this point, we can use ThinkPHP6 to develop RESTful API. Using this framework can greatly speed up our efficiency in developing RESTful APIs. At the same time, it also provides better maintainability for our API applications.
The above is the detailed content of Implementing RESTful API using ThinkPHP6. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
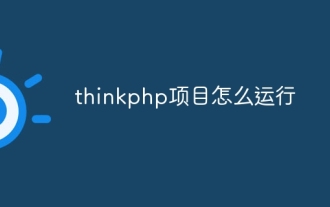
To run the ThinkPHP project, you need to: install Composer; use Composer to create the project; enter the project directory and execute php bin/console serve; visit http://localhost:8000 to view the welcome page.
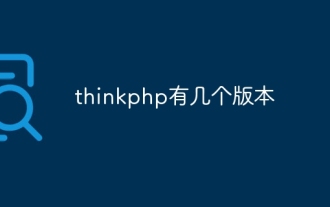
ThinkPHP has multiple versions designed for different PHP versions. Major versions include 3.2, 5.0, 5.1, and 6.0, while minor versions are used to fix bugs and provide new features. The latest stable version is ThinkPHP 6.0.16. When choosing a version, consider the PHP version, feature requirements, and community support. It is recommended to use the latest stable version for best performance and support.
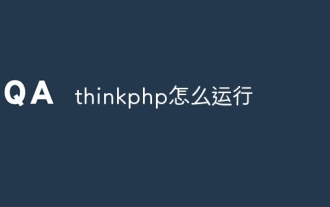
Steps to run ThinkPHP Framework locally: Download and unzip ThinkPHP Framework to a local directory. Create a virtual host (optional) pointing to the ThinkPHP root directory. Configure database connection parameters. Start the web server. Initialize the ThinkPHP application. Access the ThinkPHP application URL and run it.
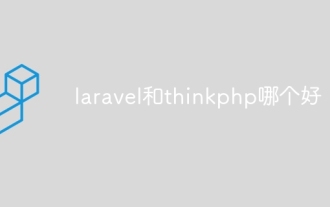
Performance comparison of Laravel and ThinkPHP frameworks: ThinkPHP generally performs better than Laravel, focusing on optimization and caching. Laravel performs well, but for complex applications, ThinkPHP may be a better fit.
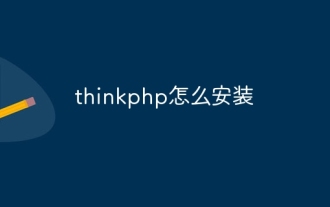
ThinkPHP installation steps: Prepare PHP, Composer, and MySQL environments. Create projects using Composer. Install the ThinkPHP framework and dependencies. Configure database connection. Generate application code. Launch the application and visit http://localhost:8000.

Oracle is a world-renowned database management system provider, and its API (Application Programming Interface) is a powerful tool that helps developers easily interact and integrate with Oracle databases. In this article, we will delve into the Oracle API usage guide, show readers how to utilize data interface technology during the development process, and provide specific code examples. 1.Oracle
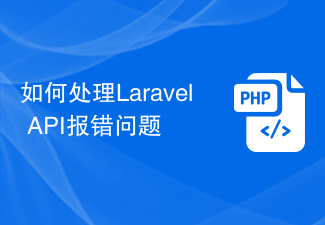
Title: How to deal with Laravel API error problems, specific code examples are needed. When developing Laravel, API errors are often encountered. These errors may come from various reasons such as program code logic errors, database query problems, or external API request failures. How to handle these error reports is a key issue. This article will use specific code examples to demonstrate how to effectively handle Laravel API error reports. 1. Error handling in Laravel
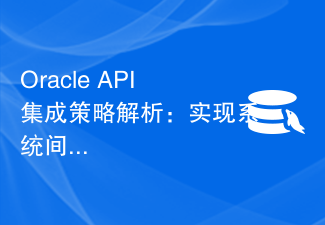
OracleAPI integration strategy analysis: To achieve seamless communication between systems, specific code examples are required. In today's digital era, internal enterprise systems need to communicate with each other and share data, and OracleAPI is one of the important tools to help achieve seamless communication between systems. This article will start with the basic concepts and principles of OracleAPI, explore API integration strategies, and finally give specific code examples to help readers better understand and apply OracleAPI. 1. Basic Oracle API
