How to get the last few characters of an array in php
When developing PHP, you often need to operate on arrays. Among them, getting the last few elements of an array is a common requirement. This article will introduce several ways to achieve this goal.
1. array_slice() function
array_slice() function can obtain elements within a specified range from an array.
Syntax:
array array_slice ( array $array , int $offset [, int $length = NULL [, bool $preserve_keys = false ]] )
Parameter description:
- array: source array
- offset: starting position. If offset is a negative number, counting starts from the end, for example -1 means the first element from the bottom
- length: the number of elements to be obtained. The default is null, which means all elements from offset to the end of the array
- preserve_keys: Whether to preserve key names. The default is false, that is, the new array uses consecutive integer key names
Code example:
// 定义数组 $arr = ['a', 'b', 'c', 'd', 'e', 'f', 'g']; // 获取倒数 3 个元素 $newArr = array_slice($arr, -3); // 输出结果 var_dump($newArr); // array(3) { [4]=> string(1) "e" [5]=> string(1) "f" [6]=> string(1) "g" }
2. array_splice() function
array_splice() function can be obtained from Removes an element from an array and replaces the removed element with a new element.
Syntax:
array array_splice ( array &$input , int $offset [, int $length = 0 [, mixed $replacement ]] )
Parameter description:
- input: source array, passed by reference
- offset: the start of the element to be removed Location. If offset is a negative number, counting starts from the end, for example -1 means the first element from the bottom
- length: the number of elements to be removed. The default is 0, which means all elements from offset to the end of the array
- replacement: the replacement element to be inserted
Code example:
// 定义数组 $arr = ['a', 'b', 'c', 'd', 'e', 'f', 'g']; // 移除前 4 个元素,插入新元素 array_splice($arr, 0, count($arr)-3, ['x', 'y', 'z']); // 输出结果 var_dump($arr); // array(6) { [0]=> string(1) "x" [1]=> string(1) "y" [2]=> string(1) "z" [3]=> string(1) "e" [4]=> string(1) "f" [5]=> string(1) "g" }
3. end() Function
end() function returns the last element of the array.
Syntax:
mixed end ( array &$array )
Parameter description:
- array: source array, passed by reference
Code example:
// 定义数组 $arr = ['a', 'b', 'c', 'd', 'e', 'f', 'g']; // 获取最后 3 个元素 $newArr = []; for ($i=0; $i<3; $i++) { $newArr[] = end($arr); prev($arr); } // 输出结果 var_dump($newArr); // array(3) { [0]=> string(1) "g" [1]=> string(1) "f" [2]=> string(1) "e" }
Among the above methods, the array_slice() function is the most intuitive and easy to understand method. The array_splice() function can implement more complex operations, but requires extra attention to the order of removed elements and new elements. The end() function provides a different idea and can be used in some special scenarios.
In short, in actual development, only by choosing different methods according to different needs can we better operate arrays.
The above is the detailed content of How to get the last few characters of an array in php. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
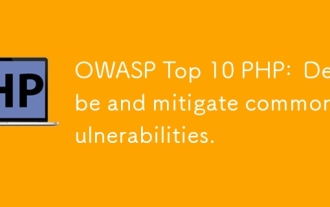
The article discusses OWASP Top 10 vulnerabilities in PHP and mitigation strategies. Key issues include injection, broken authentication, and XSS, with recommended tools for monitoring and securing PHP applications.
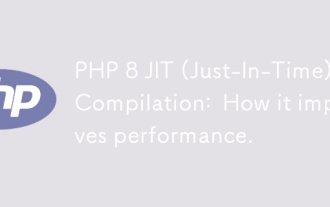
PHP 8's JIT compilation enhances performance by compiling frequently executed code into machine code, benefiting applications with heavy computations and reducing execution times.
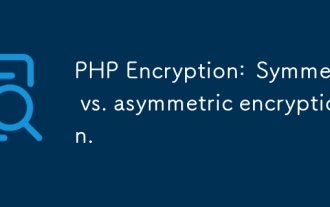
The article discusses symmetric and asymmetric encryption in PHP, comparing their suitability, performance, and security differences. Symmetric encryption is faster and suited for bulk data, while asymmetric is used for secure key exchange.
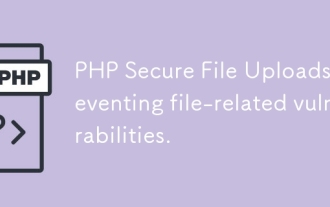
The article discusses securing PHP file uploads to prevent vulnerabilities like code injection. It focuses on file type validation, secure storage, and error handling to enhance application security.

The article discusses implementing robust authentication and authorization in PHP to prevent unauthorized access, detailing best practices and recommending security-enhancing tools.
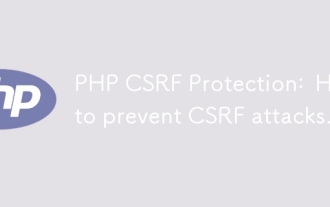
The article discusses strategies to prevent CSRF attacks in PHP, including using CSRF tokens, Same-Site cookies, and proper session management.

Article discusses best practices for PHP input validation to enhance security, focusing on techniques like using built-in functions, whitelist approach, and server-side validation.
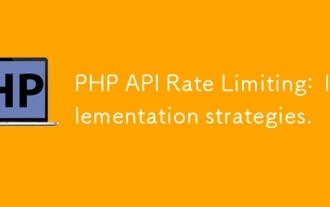
The article discusses strategies for implementing API rate limiting in PHP, including algorithms like Token Bucket and Leaky Bucket, and using libraries like symfony/rate-limiter. It also covers monitoring, dynamically adjusting rate limits, and hand
