Implementing a simple Laravel Facade using PHP features traits
The following tutorial column from Laravel will introduce how to use PHP traits to implement a simple Facade. I hope it will be helpful to everyone!
##Brief Description
Facade can effectively help me realize the staticization of the method.
Laravel Most extension packages use
Facade. [Recommended:
laravel video tutorial]The following simple
Facade mainly uses PHP's features
trait, magic method
__callStatic, Reflection Class
ReflectionClass.
Usage scenarios
Most background systems will have operations similar to this:<?php $user = User::find($id);if (!$user) { throw new \Expection("资源不存在");}
$article = Article::find($id);if (!$article) { throw new \Expection("资源不存在");}$article->delete();
Code
1. First we should have a Service
<?phpnamespace App\Services;use App\Traits\ModeServiceTrait;class ModelService extends BaseService{ use ModeServiceTrait;}
2. Create a new Trait
trait exists for multiple inheritance. You can go to the PHP official website to read the documentation.<?php namespace App\Traits; use \ReflectionClass; use \Exception;use \ReflectionException; use Illuminate\Database\Eloquent\Model; use App\Exceptions\ResourceException; /** * @method static Model find(string $className, int $id, callable $callback = null) * * @see Model * @package App\Services */trait ModeServiceTrait{ /** * 回调方法 * * @param Model|null $model * @param string $method * @return Model * @throws ResourceException */ public static function callback(Model|null $model, string $method): Model { switch ($method) { case 'first': case 'find': if (!$model) { throw new ResourceException("资源不存在"); } break; default: break; } return $model; } /** * 调用不存在的方法时触发 * * @param $method * @param $args * @return false|mixed * @throws ReflectionException * @throws ResourceException * @throws Exception */ public static function __callStatic($method, $args) { $className = $args[0]; $arg = $args[1]; // 判断模型类是否存在 if (!class_exists($className)) { throw new Exception("The class {$className} could not be found. from:" . __CLASS__); } // 利用反射实例化其类 $reflection = new ReflectionClass($className); $instance = $reflection->newInstanceArgs(); // 调用该不存在的方法 $model = call_user_func_array([$instance, $method], [$arg]); // 如果存在复杂操作交给 callback return isset($args[2]) ? $args[2]($model) : self::callback($model, $method); }}
__callStatic magic method. This method is triggered when a non-existing static method is called. A magic method similar to his is
__call. This is to use
__callStatic to achieve the effect of
Facade.
__callStatic There are two callback parameters
$method is a
called method that does not exist ,
$args It is the parameter (array form) passed in the
$method method.
trait is completed.
Use
We create a newcommand
$ php artisan make:command TestCommand
<?php namespace App\Console\Commands; use Illuminate\Console\Command; use App\Services\ModelService; use App\Models\Article\Article; class TestCommand extends Command{ /** * The name and signature of the console command. * * @var string */ protected $signature = 'test:test'; /** * The console command description. * * @var string */ protected $description = 'a test'; /** * Create a new command instance. * * @return void */ public function __construct() { parent::__construct(); } /** * Execute the console command. */ public function handle() { $article = ModelService::find(Article::class, 1); $article = ModelService::find(Article::class, 1, function ($model) { return $model->load('author'); }); }}
Article model needs to be created by yourself.
Next you can see the effect:
$ php artisan test:test
Conclusion
In this way we abandon the use ofabstract Abstract class to achieve the same effect as
Facade. Code reuse is also achieved.
Using the program in this way will take a lot more steps, but compared to elegance, performance doesn't matter.
The above is the detailed content of Implementing a simple Laravel Facade using PHP features traits. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
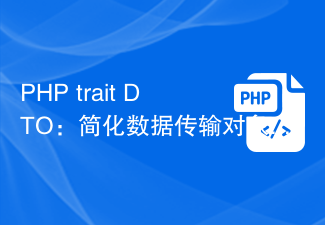
PHPtraitDTO: Simplifying the development of data transfer objects Introduction: In modern software development, data transfer objects (DataTransferObject, referred to as DTO) play an important role. DTO is a pure data container used to transfer data between layers. However, during the development process, developers need to write a large amount of similar code to define and operate DTOs. In order to simplify this process, the trait feature was introduced in PHP. We can use the trait feature to
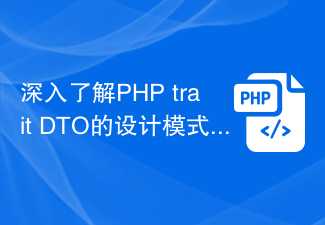
In-depth understanding of the design patterns and practices of PHPtraitDTO Introduction: In PHP development, design patterns are an essential part. Among them, DTO (DataTransferObject) is a commonly used design pattern used to encapsulate data transfer objects. In the process of implementing DTO, using traits can effectively improve the reusability and flexibility of the code. This article will delve into the design patterns and practices of traitDTO in PHP

PHPtraitDTO: A key tool for optimizing the data transmission process. Specific code examples are required. Introduction: During the development process, data transmission is a very common requirement, especially when data is transferred between different levels. In the process of transmitting this data, we often need to process, verify or convert the data to meet different business needs. In order to improve the readability and maintainability of the code, we can use PHPtraitDTO (DataTransferObject) to optimize
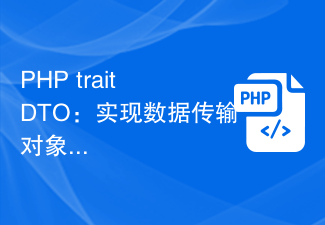
PHPtraitDTO: Implementing simplicity and flexibility of data transfer objects Introduction: In the PHP development process, data transmission and processing are often involved. The DataTransferObject (DTO for short) is a design pattern that is used to transfer data between different layers. During the transmission process, DTO simplifies data operations by encapsulating data and providing public access methods. This article will introduce how to use PHPtrait to implement DT
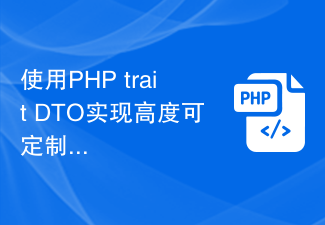
Implementing a highly customizable data transfer framework using PHPtraitDTO As websites and applications become more complex, data transfer becomes more and more important. In PHP, using DataTransferObject (DTO for short) to handle data transfer can greatly simplify the code and improve maintainability and scalability. This article will introduce how to use PHPtrait and DTO to implement a highly customizable data transfer framework and provide corresponding code examples.
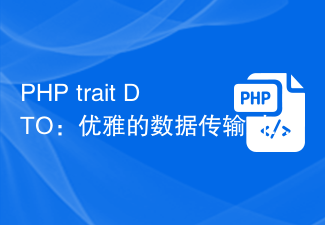
PHPtraitDTO: Elegant Data Transfer Object Pattern Overview: Data Transfer Object (DTO for short) is a design pattern used to transfer data between different layers. In applications, it is often necessary to obtain data from a database or external service and pass it between different layers of the application. The DTO mode can make data transmission more concise and clear, and also facilitates expansion and maintenance. In PHP, we can use traits to implement DTO
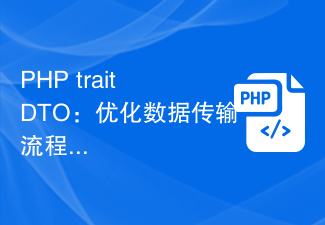
PHPtraitDTO: A key tool for optimizing the data transmission process. Specific code examples are required. In the development process, data transmission is a very critical link. How to transmit data efficiently has become one of the problems that developers need to solve. In PHP language, using traitDTO (DataTransferObject) can optimize the data transmission process and improve the efficiency of data transmission. This article will introduce what traitDTO is and how to use it to optimize the data transfer flow
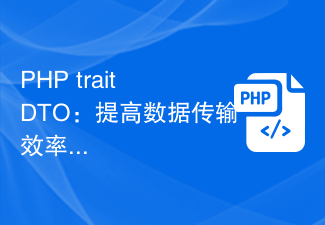
PHPtraitDTO: Key technology introduction to improving data transmission efficiency: In modern software development, data transmission is a very important link. In most applications, data needs to be transferred from one place to another, such as from the database to the front-end page, from the front-end form to the back-end processing logic, etc. The efficiency of data transmission directly affects the performance and user experience of the entire system. In order to improve the efficiency of data transfer, we can use PHP's traitDTO (DataTransferO
