Detailed steps for encapsulating React Context Composer (share)
This article is written by composer Tutorial column to introduce to you how to encapsulate a React Context Composer step by step. I hope it will be helpful to friends in need!
How do I encapsulate a React Context Composer step by step?
Motivation
There are many state management solutions for React, such as Redux, Mobx, Recoil, etc. So far, I have only experienced Redux, and I think it is still a bit cumbersome. . Because I usually write a lot of Hooks, I prefer to use Context Provider with the useContext hook, which makes it easy to split and combine states. Here, we will not discuss the pros and cons of each state management solution, but focus on a multi-layer nesting problem encountered when using Context.
The picture below is some code extracted from a taro react hooks ts project I was writing recently. I split some global state (the purpose of splitting is to reduce unnecessary re-rendering) and then nested them. This way of writing reminds me of the feeling of being dominated by callback hell, which is very uncomfortable. Therefore, I thought of sealing a high-order component myself and "flattening" the structure in terms of writing.
<LoadingContext.Provider value={{ loading, setLoading }}> <UserDataContext.Provider value={{ name: "ascodelife", age: 25 }}> <ThemeContext.Provider value={"light"}> {/* ....more Providers as long as you want */} </ThemeContext.Provider> </UserDataContext.Provider> </LoadingContext.Provider>
The easiest solution
Here, I quickly wrote the first solution, using reduceRight to complete the nesting of Provider.
The reason reduceRight is used here instead of reduce is that we are more accustomed to the writing order from the outer layer to the inner layer.
// ContextComposer.tsx import React from 'react'; type IContextComposerProps = { contexts: { context: React.Context<any>; value: any }[]; }; const ContextComposer: React.FC<IContextComposerProps> = ({ contexts, children }) => { return ( <> {contexts.reduceRight((child, parent) => { const { context, value } = parent; return <context.Provider value={value}>{child}</context.Provider>; }, children)} </> ); }; export default ContextComposer; // App.tsx <ContextComposer contexts={[ { context: ThemeContext, value: "light" }, { context: UserDataContext, value: { name: "ascodelife", age: 25 } }, { context: LoadingContext, value: { loading, setLoading } }, ]}> { children } </ContextComposer>
After actual experience, I found that although it can be used, the development experience is a little bit worse. The problem is that the value passed when the component enters the parameter is of type any, which means that the static type check of ts is abandoned. When passing parameters, since static type checking will not be done on the value, not only will there not be any code prompts when typing the code, but it may also cause some relatively low-level runtime errors. Bad review!
Transformation plan based on React.cloneElement()
In order to transform the above scheme, I turned to a relatively unpopular but easy-to-use function——React. cloneElement(). There are not many points worth noting about this function. Mainly take a look at its three input parameters. The first is the parent element, the second is the parent props, and the third is the remaining parameters...children, except for the first parameter. Except for this, all other values are optional.
For example:
<!-- 调用函数 --> React.cloneElement(<div/>,{},<span/>); <!-- 相当于创建了这样一个结构 --> <div> <span></span> </div>
Then let’s start the transformation. The reduceRight frame remains unchanged. Change the input parameter type and reduceRight callback.
// ContextComposer.tsx import React from 'react'; type IContextComposerProps = { contexts: React.ReactElement[]; }; const ContextComposer: React.FC<IContextComposerProps> = ({ contexts, children }) => { return ( <> {contexts.reduceRight((child, parent) => { return React.cloneElement(parent,{},child); }, children)} </> ); }; export default ContextComposer; // App.tsx <ContextComposer contexts={[ <ThemeContext.Provider value={"light"} />, <UserDataContext.Provider value={{ name: "ascodelife", age: 25 }} />, <LoadingContext.Provider value={{ loading, setLoading }} />, ]}> { children } </ContextComposer>
After the transformation, when we pass parameters, it seems that we are really creating a component (of course, the component is actually created, but the component itself is not rendered to the virtual Dom, and is actually rendered. is a cloned copy). At the same time, the static type checking problem of value that we just focused on has also been solved.
tips: React.cloneElement(parent,{},child) is equivalent to React.cloneElement(parent,{children:child}), do you know why?
Related resources
The source code has been synchronized to github (https://github.com/ascodelife/react-context-provider-composer).
It has also been packaged into the npm warehouse (https://www.npmjs.com/package/@ascodelife/react-context-provider-composer). Welcome to experience it.
The above is the detailed content of Detailed steps for encapsulating React Context Composer (share). For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics

Composer is a dependency management tool for PHP, used to declare, download and manage project dependencies. 1) Declare dependencies through composer.json file, 2) Install dependencies using composerinstall command, 3) parse the dependency tree and download it from Packagist, 4) generate the autoload.php file to simplify automatic loading, 5) optimize use includes using composerupdate--prefer-dist and adjusting the autoload configuration.

Composer is a dependency management tool for PHP. The core steps of using Composer include: 1) Declare dependencies in composer.json, such as "stripe/stripe-php":"^7.0"; 2) Run composerinstall to download and configure dependencies; 3) Manage versions and autoloads through composer.lock and autoload.php. Composer simplifies dependency management and improves project efficiency and maintainability.

Composer is used to manage dependencies on PHP projects, while Orchestrator is used to manage and coordinate microservices or containerized applications. 1.Composer declares and manages dependencies of PHP projects through composer.json file. 2. Orchestrator manages the deployment and extension of services through configuration files (such as Kubernetes' YAML files), ensuring high availability and load balancing.

To become proficient when using Composer, you need to master the following skills: 1. Proficient in using composer.json and composer.lock files, 2. Understand how Composer works, 3. Master Composer's command line tools, 4. Understand basic and advanced usage, 5. Familiar with common errors and debugging techniques, 6. Optimize usage and follow best practices.
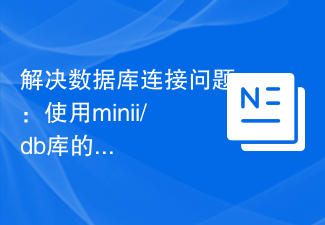
I encountered a tricky problem when developing a small application: the need to quickly integrate a lightweight database operation library. After trying multiple libraries, I found that they either have too much functionality or are not very compatible. Eventually, I found minii/db, a simplified version based on Yii2 that solved my problem perfectly.
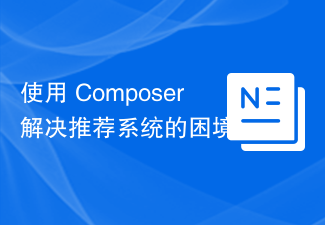
When developing an e-commerce website, I encountered a difficult problem: how to provide users with personalized product recommendations. Initially, I tried some simple recommendation algorithms, but the results were not ideal, and user satisfaction was also affected. In order to improve the accuracy and efficiency of the recommendation system, I decided to adopt a more professional solution. Finally, I installed andres-montanez/recommendations-bundle through Composer, which not only solved my problem, but also greatly improved the performance of the recommendation system. You can learn composer through the following address:
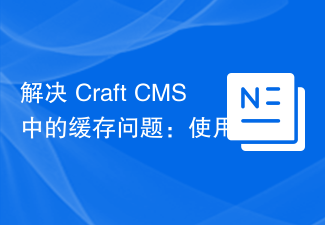
When developing websites using CraftCMS, you often encounter resource file caching problems, especially when you frequently update CSS and JavaScript files, old versions of files may still be cached by the browser, causing users to not see the latest changes in time. This problem not only affects the user experience, but also increases the difficulty of development and debugging. Recently, I encountered similar troubles in my project, and after some exploration, I found the plugin wiejeben/craft-laravel-mix, which perfectly solved my caching problem.
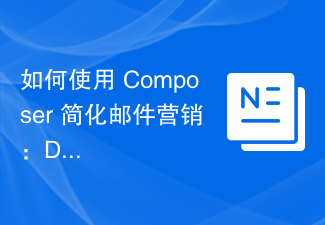
I'm having a tricky problem when doing a mail marketing campaign: how to efficiently create and send mail in HTML format. The traditional approach is to write code manually and send emails using an SMTP server, but this is not only time consuming, but also error-prone. After trying multiple solutions, I discovered DUWA.io, a simple and easy-to-use RESTAPI that helps me create and send HTML mail quickly. To further simplify the development process, I decided to use Composer to install and manage DUWA.io's PHP library - captaindoe/duwa.
