What is an interface in javascript
In JavaScript, an interface refers to a reference type that defines a contract. The interface actually tells us what methods a class implements, thereby helping it use this class; the interface can make the code more stable.
The operating environment of this tutorial: windows7 system, javascript version 1.8.5, Dell G3 computer.
There is no built-in way to create or implement interfaces in JavaScript. It also has no built-in method for determining whether one object implements the same set of methods as another object. This makes it difficult to use objects interchangeably. But JavaScript is very flexible, and we can implement it in other ways.
What is an interface
The interface actually defines what methods should be in the object. He does not need to consider how these methods are implemented, but defines that the object has these methods.
Interface refers to the reference type that defines the agreement. Other types implement interfaces to ensure that they support certain operations. An interface specifies the members that must be provided by a class or other interface that implements it. Similar to classes, interfaces can contain methods, properties, indexers, and events as members.
Benefits of interfaces
Interfaces actually tell us which methods a class implements. This helps them use this class. Interfaces can make our code more stable if we add a method to the interface. If a class that implements it does not add this method accordingly, it will definitely throw an error.
javascript imitation interface
There are three methods for JavaScript imitation interface:
Commentation method
Attribute checking method
Duck transformation method
Use comments to describe the interface
Using comments to describe the interface is the simplest method, but the effect is the worst.
/* interface Composite { function add(child); function remove(child); function getChild(index); } interface FormItem { function save(); } */ class CompositeForm { add(child) { //... } remove(child) { } getChild(index) { //... } save() { //... } }
This imitation method is not very good. It does not check whether CompositeForm implements the method correctly. It is entirely through programmers consciously implementing the interface in the annotation. However, this implementation is very simple, but it does not help in testing and debugging.
Attribute checking method imitates interface
This method is more cautious, but the interface is also written in the form of comments, but you can know a certain class by checking an attribute What interface does it claim to implement?
class CompositeForm { constructor() { this.implementsInterface = ['Composite', 'FormItem']; } } function addForm(formInstance) { if (!implements(formInstance, 'Composite', 'FormItem')) { throw new Error('对象没有实现接口方法'); } } function implements(obj) { // 这个方法查询接口 for (let i = 1; i < arguments.length; i++) { let interfaceName = arguments[i]; let interfaceFound = false; for (let j = 1; j < obj.implementsInterface.length; j++) { if (obj.implementsInterface[j] == interfaceName) { interfaceFound = true; break; } } if (!interfaceFound) { return false; } return true; } } addForm(new CompositeForm());
The advantage of this method is that it provides documentation for the interface implemented by the class. If the required interface is not among the interfaces that my class declares to support (that is, it is not in my this.implementsInterface) ), you will see an error message.
The shortcomings are also obvious. If the interface declared in my this.implementsInterface is different from the interface defined in my comments but the check can still pass, it means that calling the addForm method will not cause an error.
Using duck transformation to simulate interfaces
In fact, it doesn’t matter whether a class declares which interfaces it supports, as long as it has the methods in these interfaces. Duck transformation uses the method set of an object as the only criterion for judging whether it is an instance. The implementation principle is also very simple: if an object has a method with the same name as the method defined by the interface, then it can be considered that it implements the interface.
// interface class Interface { constructor(name, method) { if (arguments.length != 2) { throw new Error('两个参数:name method'); } this.name = name; this.method = []; for (let i in method) { if (typeof method[i] != 'string') { throw new Error('method 必须是字符串'); } this.method.push(method[i]); } } //检查接口方法 static ensureImplements(obj) { if (arguments.length < 2) { throw new Error('参数小于两个'); } for (let i = 1; i < arguments.length; i++) { var instanceInterface = arguments[i]; if (instanceInterface.constructor !== Interface) { throw new Error('你要检查的参数不属于Interface接口') } for (let j in instanceInterface.method) { let methodName = instanceInterface.method[j]; if (!obj[methodName] || typeof obj[methodName] !== 'function') { throw new Error(`请实现接口的${methodName}方法`) } } } } } // 实例化接口对象 var Composite = new Interface('Composite', ['add', 'remove', 'getChild']); var FormItem = new Interface('FormItem', ['save']); // CompositeForm 类 class CompositeForm { //... add() {} remove() {} getChild() {} } let c1 = new CompositeForm(); Interface.ensureImplements(c1, Composite, FormItem); function addForm(formInterface) { ensureImplements(formInterface, Composite, FormItem); }
I did not implement the save method in the CompositeForm class in the above code. Running this code will result in an error.
But the duck transformation method also has shortcomings, and all inspection aspects are mandatory.
This rookie’s implementation method > I call myself the inheritance method
I used class inheritance to simulate the interface. Please see the code for the specific implementation.
First we define a class used as an interface. The attribute method represents the method set of the interface.
class Interface { constructor() { this.mehods = ['add', 'save', 'remove', 'save']; } static ensureImplements(obj) { //... } }
Define a CompositeForm class to inherit this interface, and call the ensureImplements method of the parent class in the class to detect Interface
class CompositeForm extends Interface{ constructor() { super().ensureImplements(this); } }
Improve the ensureImplements method
class Interface { constructor() { this.mehods = ['add', 'save', 'remove', 'save']; } static ensureImplements(obj) { for (let i in this.mehods) { let methodName = this.mehods[i] if (!obj[methodName] || typeof obj[methodName] !== 'function') { let err = '请实现接口' + methodName + '的方法'; throw new Error(err); } } } }
[Recommended learning: javascript advanced tutorial】
The above is the detailed content of What is an interface in javascript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
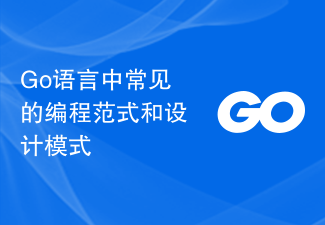
As a modern and efficient programming language, Go language has rich programming paradigms and design patterns that can help developers write high-quality, maintainable code. This article will introduce common programming paradigms and design patterns in the Go language and provide specific code examples. 1. Object-oriented programming In the Go language, you can use structures and methods to implement object-oriented programming. By defining a structure and binding methods to the structure, the object-oriented features of data encapsulation and behavior binding can be achieved. packagemaini
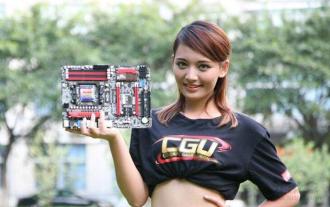
When we assemble the computer, although the installation process is simple, we often encounter problems in the wiring. Often, users mistakenly plug the power supply line of the CPU radiator into the SYS_FAN. Although the fan can rotate, it may not work when the computer is turned on. There will be an F1 error "CPUFanError", which also causes the CPU cooler to be unable to adjust the speed intelligently. Let's share the common knowledge about the CPU_FAN, SYS_FAN, CHA_FAN, and CPU_OPT interfaces on the computer motherboard. Popular science on the CPU_FAN, SYS_FAN, CHA_FAN, and CPU_OPT interfaces on the computer motherboard 1. CPU_FANCPU_FAN is a dedicated interface for the CPU radiator and works at 12V
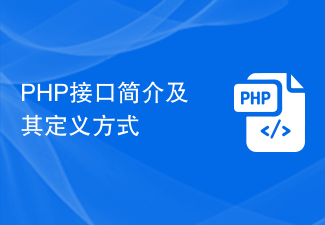
Introduction to PHP interface and how it is defined. PHP is an open source scripting language widely used in Web development. It is flexible, simple, and powerful. In PHP, an interface is a tool that defines common methods between multiple classes, achieving polymorphism and making code more flexible and reusable. This article will introduce the concept of PHP interfaces and how to define them, and provide specific code examples to demonstrate their usage. 1. PHP interface concept Interface plays an important role in object-oriented programming, defining the class application
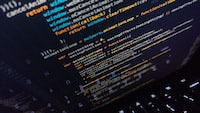
The reason for the error is in python. The reason why NotImplementedError() is thrown in Tornado may be because an abstract method or interface is not implemented. These methods or interfaces are declared in the parent class but not implemented in the child class. Subclasses need to implement these methods or interfaces to work properly. How to solve this problem is to implement the abstract method or interface declared by the parent class in the child class. If you are using a class to inherit from another class and you see this error, you should implement all the abstract methods declared in the parent class in the child class. If you are using an interface and you see this error, you should implement all methods declared in the interface in the class that implements the interface. If you are not sure which
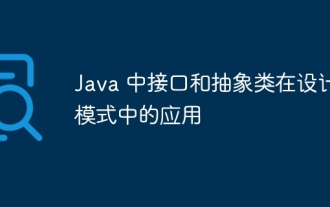
Interfaces and abstract classes are used in design patterns for decoupling and extensibility. Interfaces define method signatures, abstract classes provide partial implementation, and subclasses must implement unimplemented methods. In the strategy pattern, the interface is used to define the algorithm, and the abstract class or concrete class provides the implementation, allowing dynamic switching of algorithms. In the observer pattern, interfaces are used to define observer behavior, and abstract or concrete classes are used to subscribe and publish notifications. In the adapter pattern, interfaces are used to adapt existing classes. Abstract classes or concrete classes can implement compatible interfaces, allowing interaction with original code.
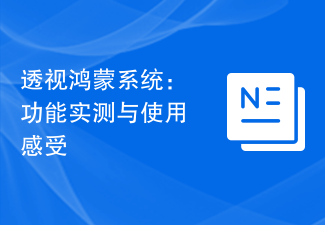
As a new operating system launched by Huawei, Hongmeng system has caused quite a stir in the industry. As a new attempt by Huawei after the US ban, Hongmeng system has high hopes and expectations. Recently, I was fortunate enough to get a Huawei mobile phone equipped with Hongmeng system. After a period of use and actual testing, I will share some functional testing and usage experience of Hongmeng system. First, let’s take a look at the interface and functions of Hongmeng system. The Hongmeng system adopts Huawei's own design style as a whole, which is simple, clear and smooth in operation. On the desktop, various
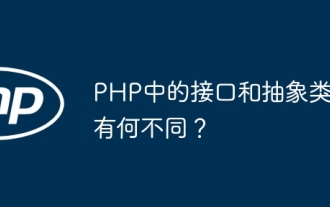
Interfaces and abstract classes are used to create extensible PHP code, and there is the following key difference between them: Interfaces enforce through implementation, while abstract classes enforce through inheritance. Interfaces cannot contain concrete methods, while abstract classes can. A class can implement multiple interfaces, but can only inherit from one abstract class. Interfaces cannot be instantiated, but abstract classes can.

Java allows inner classes to be defined within interfaces and abstract classes, providing flexibility for code reuse and modularization. Inner classes in interfaces can implement specific functions, while inner classes in abstract classes can define general functions, and subclasses provide concrete implementations.
