


Detailed explanation of the concept of method overloading in JAVA - simple and easy to understand
1. What method is overloaded?
The overloading of the method refers to the method name is the same, but the parameter type is different
1.1, attack method overloading
There is a hero called the physical attack hero ADHero, which provides three methods for ADHero.
public void attack() public void attack(Hero h1) public void attack(Hero h1, Hero h2)
The method names are the same, but the parameter types are different
When calling the attack method, the corresponding method will be automatically called based on the type and quantity of parameters passed
public class ADHero extends Hero { public void attack() { System.out.println(name + " 进行了一次攻击 ,但是不确定打中谁了"); } public void attack(Hero h1) { System.out.println(name + "对" + h1.name + "进行了一次攻击 "); } public void attack(Hero h1, Hero h2) { System.out.println(name + "同时对" + h1.name + "和" + h2.name + "进行了攻击 "); } public static void main(String[] args) { ADHero bh = new ADHero(); bh.name = "赏金猎人"; Hero h1 = new Hero(); h1.name = "盖伦"; Hero h2 = new Hero(); h2.name = "提莫"; bh.attack(h1); bh.attack(h1, h2); } }
1.2. Variable number of parameters
If you want to attack more heroes, you need to design more methods, so that the class will appear It’s very cumbersome, like this:
public void attack(Hero h1) public void attack(Hero h1,Hero h2) public void attack(Hero h1,Hero h2,Hero 3)
At this time, you can use a variable number of parameters
You only need to design a method
public void attack(Hero...heros)
It can represent All the above methods
In the method, use the method of operating array to process the parameter heros
public class ADHero extends Hero { public void attack() { System.out.println(name + " 进行了一次攻击 ,但是不确定打中谁了"); } // 可变数量的参数 public void attack(Hero... heros) { for (int i = 0; i < heros.length; i++) { System.out.println(name + " 攻击了 " + heros[i].name); } } public static void main(String[] args) { ADHero bh = new ADHero(); bh.name = "赏金猎人"; Hero h1 = new Hero(); h1.name = "盖伦"; Hero h2 = new Hero(); h2.name = "提莫"; bh.attack(h1); bh.attack(h1, h2); } }
Exercise: Treatment
(Design a class Support (auxiliary hero) to inherit Hero and provide a heal (Treatment) method
Overload the heal method of Support
heal()
heal(Hero h) //Add blood to the specified hero
heal(Hero h, int hp) // Adds hp to the specified hero)
Answer
public class Support extends Hero { public void heal() { System.out.println("对自己进行治疗"); } public void heal(Hero hero) { System.out.println("给英雄 " + hero + " 加血"); } public void heal(Hero hero, int hp) { System.out.println("给英雄 " + hero + " 加了 " + hp + "点血"); } }
For more related content, please visit the PHP Chinese website: JAVA Video Tutorial
The above is the detailed content of Detailed explanation of the concept of method overloading in JAVA - simple and easy to understand. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










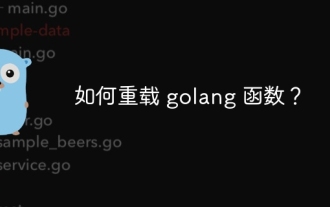
Traditional function overloading is not supported in Go, but it can be simulated by the following techniques: Multiple return values: Functions with the same method signature but different return types can be overloaded. Variadics: Use the ... syntax to create functions that receive a variable number of arguments, allowing method calls to be handled with different signatures.
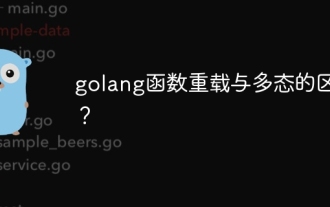
Function overloading is not supported in Go language because it adopts duck typing and determines the value type based on the actual type. Polymorphism is achieved through interface types and method calls, and objects of different categories can respond in the same way. Specifically, by defining interfaces and implementing these methods, Go language can make objects of different types have similar behaviors, thereby supporting polymorphism.

Differences: 1. MySQL is a relational database, while NoSQL is non-relational. 2. MySQL’s strict mode restrictions are not easy to expand, while NoSQL is easy to expand. 3. MySQL requires a detailed database model before creating a database, which is not required in NoSQL. 4. MySQL provides a large number of reporting tools, but nosql does not. 5. Compared with MySQL, NoSQL provides a more flexible design. 6. The standard language used in MySQL is SQL, while NoSQL lacks a standard query language.
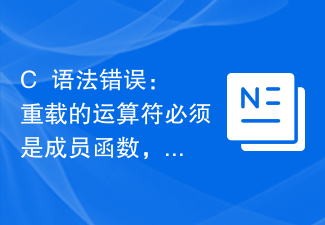
C++ is a popular programming language with powerful object-oriented programming capabilities. When programming in C++, you may sometimes encounter some syntax errors. This article will discuss a common error, "Overloaded operator must be a member function" and provide a solution to solve the problem. In C++, operators can be overloaded to perform various operations using objects of a custom class. For example, the "+" operator can be overloaded to implement addition between two custom class objects. Operator overloading can be done through member functions
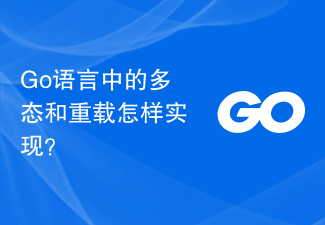
As a statically typed language, Go language does not seem to be able to implement polymorphism and overloading like dynamic languages. However, the Go language uses the characteristics of interfaces to achieve polymorphism, and the implementation of overloading is simpler and more accurate. Methods to implement polymorphism The interface in the Go language can implement polymorphism during the calling process. The interface can describe the behavior of an object. Any type that implements all methods of the interface can be called an instance of the interface type. In this way, polymorphism can be achieved by simply defining the interface type and implementing different concrete types. Below is a
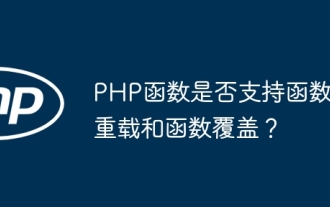
The PHP language does not support function overloading and function coverage because function overloading may cause ambiguity. Alternative: Use namespaces to isolate functions. Set parameter default values. Use variadic function arguments.

PHP is a very popular server-side scripting language used for developing web applications. However, for some beginners, understanding some concepts of PHP may cause some difficulties. This article will explore the concept of method overloading in PHP.
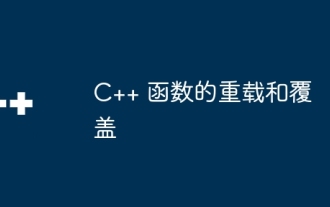
Overloading and overriding are different concepts in C++. Overloading allows the creation of a function with the same name, with a different parameter list, while overriding allows a derived class function to override a base class function with the same name. In overloading, the function name is the same but the parameter list is different. In overriding, the function name and parameter list must be the same and the derived class function must use the override keyword.
