


Detailed explanation of how to implement overloading examples of functions and constructors in PHP
Since PHP is a weakly typed language, the input parameter type of the function cannot be determined (type hints can be used, but type hints cannot be used for integer types, strings and other scalar types), and for a function, for example, only 3 input parameters are defined, but PHP inputs 4 or more parameters when calling. Therefore, based on these two points, it is destined that functions cannot be overloaded in PHP (similar to Javascript languages), and there cannot be constructors of overloading.
Since implementing function overloading is very helpful to improve development efficiency, it would be great if it could be like C# or C++. In fact, PHP provides a magic method, mixed call (string name, array arguments). This method is also mentioned in the php manual. According to the official documentation, it is said that this method can achieve function overloading. When calling a method that does not exist in the object, if the call() method is defined, the method will be called. For example, the following code:
<?php class A { function call ( $name, $arguments ) { echo "call调用<br/>"; echo '$name为'.$name."<br/>"; print_r ($arguments); } } (new A)->test("test","argument"); ?>
The running result is:
call call
$name is test
Array ( [0] => test [ 1] => argument )
So you only need to use this magic method to achieve function overloading.
The code is as follows:
<?php class A { function call ($name, $args ) { if($name=='f') { $i=count($args); if (method_exists($this,$f='f'.$i)) { call_user_func_array(array($this,$f),$args); } } } function f1($a1) { echo "1个参数".$a1."<br/>"; } function f2($a1,$a2) { echo "2个参数".$a1.",".$a2."<br/>"; } function f3($a1,$a2,$a3) { echo "3个参数".$a1.",".$a2.",".$a3."<br/>"; } } (new A)->f('a'); (new A)->f('a','b'); (new A)->f('a','b','c'); 输出如下: 1个参数a 2个参数a,b 3个参数a,b,c
Similarly, in PHP, overloading of constructors can only be implemented in a flexible way. The key element of implementation is to obtain input parameters and determine which method to call based on the input parameters. The following is a sample code:
<?php class A { function construct() { echo "执行构造函数<br/>"; $a = func_get_args(); //获取构造函数中的参数 $i = count($a); if (method_exists($this,$f='construct'.$i)) { call_user_func_array(array($this,$f),$a); } } function construct1($a1) { echo "1个参数".$a1."<br/>"; } function construct2($a1,$a2) { echo "2个参数".$a1.",".$a2."<br/>"; } function construct3($a1,$a2,$a3) { echo "3个参数".$a1.",".$a2.",".$a3."<br/>"; } } $o = new A('a'); $o = new A('a','b'); $o = new A('a','b','c'); ?>
Execute the constructor
1 parameter a
Execute the constructor
2 parameters a,b
Execute the constructor
3 parameters a, b, c
By the way, just like C# and other object-oriented languages, in PHP, if the constructor is set to private or protected, the object cannot be instantiated.
The above is the detailed content of Detailed explanation of how to implement overloading examples of functions and constructors in PHP. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
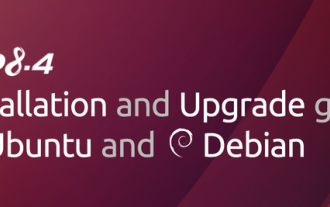
PHP 8.4 brings several new features, security improvements, and performance improvements with healthy amounts of feature deprecations and removals. This guide explains how to install PHP 8.4 or upgrade to PHP 8.4 on Ubuntu, Debian, or their derivati
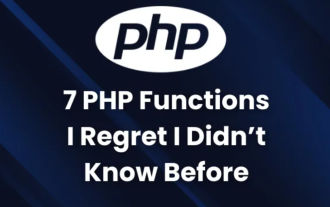
If you are an experienced PHP developer, you might have the feeling that you’ve been there and done that already.You have developed a significant number of applications, debugged millions of lines of code, and tweaked a bunch of scripts to achieve op
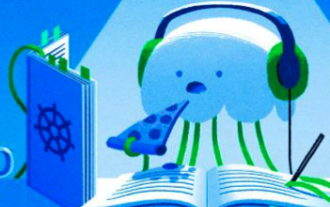
Visual Studio Code, also known as VS Code, is a free source code editor — or integrated development environment (IDE) — available for all major operating systems. With a large collection of extensions for many programming languages, VS Code can be c
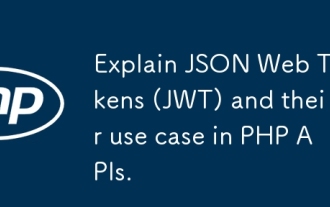
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
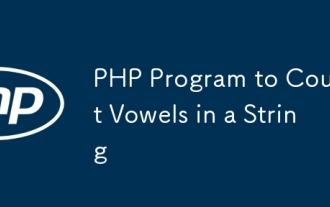
A string is a sequence of characters, including letters, numbers, and symbols. This tutorial will learn how to calculate the number of vowels in a given string in PHP using different methods. The vowels in English are a, e, i, o, u, and they can be uppercase or lowercase. What is a vowel? Vowels are alphabetic characters that represent a specific pronunciation. There are five vowels in English, including uppercase and lowercase: a, e, i, o, u Example 1 Input: String = "Tutorialspoint" Output: 6 explain The vowels in the string "Tutorialspoint" are u, o, i, a, o, i. There are 6 yuan in total
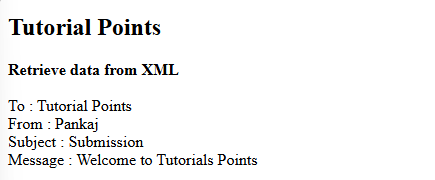
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an
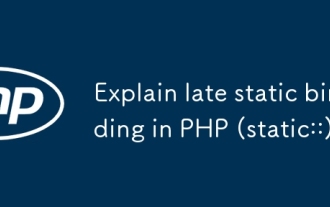
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.

What are the magic methods of PHP? PHP's magic methods include: 1.\_\_construct, used to initialize objects; 2.\_\_destruct, used to clean up resources; 3.\_\_call, handle non-existent method calls; 4.\_\_get, implement dynamic attribute access; 5.\_\_set, implement dynamic attribute settings. These methods are automatically called in certain situations, improving code flexibility and efficiency.
