A little knowledge of C# (1)
Little knowledge (1)
var:
Initialization must have a value assignment, var i; (wrong).
The type of an object of type var is not allowed to be changed during operation. (For example: var i=1; i="hello!" will cause an error)
var only declares local variables.
dynamic:
No value can be assigned during initialization.
This type can be given to dynamic type objects during operation. (For example: dynamic i=1; i="hello!" No error)
can be declared as a global variable.
VAR and DYNAMIC: Generally used when the variable type is uncertain.
decimal:
High-precision decimal, range: 1.0*10^-28 to 7.9*10^28, precision 28 digits. Generally used to express money. The precision of float/double is 7/15 digits. When using these two types of float/double to represent currency, it will "erase zeros" for you.
Decimal default value: 0.0M
float default value: 0.0F
double default value: 0.0D
char:
char character There are 3 types of assignment:
char c='d';
char c='\x0068'; hexadecimal
char c='\u0068'; Unicode means
char c=(char)68; Integer conversion to char.
Default value: '\x0000'
Octal and hexadecimal:
In C#, integers are only expressed in decimal, using the octal format specifier" o" or "O" and the hexadecimal format specifier is "x" or "X", a compilation error will occur (for example: int i = o73; error).
When you need to output octal or hexadecimal, just use i.ToString("x").
Display binary WriteLine(Convert.ToString(231,2));
- ## Display octal WriteLine(Convert.ToString( 231,8)) ;
- Display hexadecimal WriteLine(Convert.ToString(231,16));
- Display hexadecimal WriteLine(i.ToString("x")); displays lowercase.
- Display hexadecimal WriteLine(i.ToString("X")); display uppercase.
- Encoding.Default Gets the encoding of the system’s current ANSI code page
- Encoding.UTF7 Gets UTF7 Encoding format ## Encoding.UTF8 Gets the UTF8 encoding format
- Encoding.ASCII Gets the encoding of the 7-bit ASCII character set
- byte[] b=System.Text.Encoding.Unicode.GetBytes(s);
- enum enumeration:
User-defined data type. enum values start from 1, except custom ones. Separated by commas ",", integer values can be assigned.
String conversion specific enumeration:
object ← Enum.parse(type enumType,string value);
Such as:
color col=(color)Enum.parse(typeof(color),"white");
Note: If the string does not correspond to the enumeration, an error will occur.
enum e { }
struct structure:
User-defined data type, structure type. Structures can contain constructors, constants, fields, methods, properties, indexers, and operators.
The structure is a value type and the address is allocated on the stack
结构和类都可以继承接口。
结构不能被集成,类可以。
结构没有析构函数,类有。
struct s { }
以上就是C#拾遗之小知识(一)的内容,更多相关内容请关注PHP中文网(www.php.cn)!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
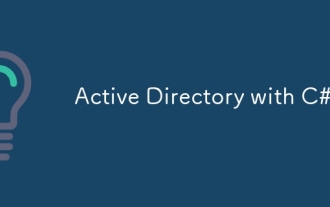
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
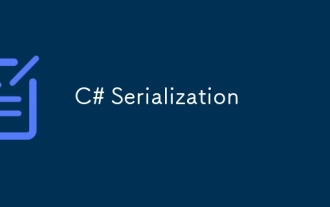
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
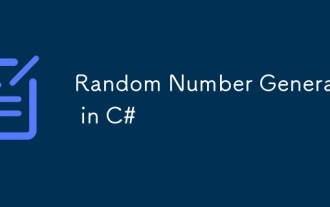
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
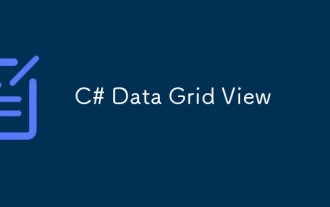
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
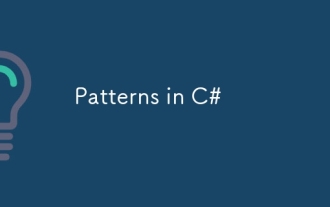
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
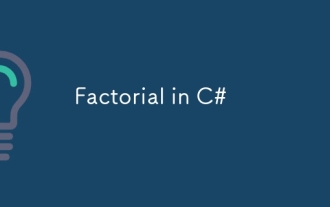
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
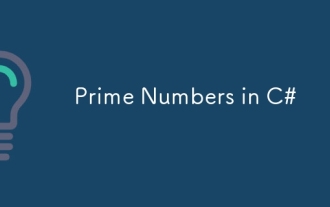
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
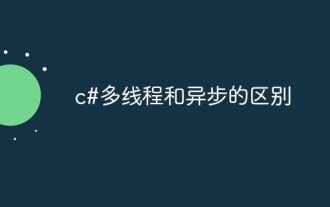
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
