Implementation of arrays in PHP kernel_PHP tutorial
Arrays are often used in PHP. PHP arrays are powerful and fast. Reading and writing can be completed in O(1) because the size of each element is the same. As long as you know the subscript, you can calculate it instantly. The location of its corresponding element in memory, so it can be directly taken out or written. So how are arrays implemented in the kernel?
Most functions of PHP are implemented through HashTable, including arrays.
HashTable has the advantages of a doubly linked list and has operational performance comparable to data.
-
The variables defined in PHP are stored in a symbol table, and this symbol table is actually a HashTable, and each element of it is a variable of type zval*. Not only that, containers that store user-defined functions, classes, resources, etc. are implemented in the kernel in the form of HashTable.
Let's look at how to define arrays in PHP and the kernel respectively.
Implementation of array definition
Define array in PHP:
<?php $array = array(); $array["key"] = "values"; ?>
Copy after login
Use macros in the kernel to achieve this:
zval* array; array_init(array); add_assoc_string(array, "key", "value", 1);
Copy after login
Expand the macro in the above code:
zval* array; ALLOC_INIT_ZVAL(array); Z_TYPE_P(array) = IS_ARRAY; HashTable *h; ALLOC_HASHTABLE(h); Z_ARRVAL_P(array)=h; zend_hash_init(h, 50, NULL,ZVAL_PTR_DTOR, 0); zval* barZval; MAKE_STD_ZVAL(barZval); ZVAL_STRING(barZval, "value", 0); zend_hash_add(h, "key", 4, &barZval, sizeof(zval*), NULL);
Copy after login
Convenient array macro operations
The kernel provides us with convenient macros to manage arrays.
//add_assoc_*系列函数: add_assoc_null(zval *aval, char *key); add_assoc_bool(zval *aval, char *key, zend_bool bval); add_assoc_long(zval *aval, char *key, long lval); add_assoc_double(zval *aval, char *key, double dval); add_assoc_string(zval *aval, char *key, char *strval, int dup); add_assoc_stringl(zval *aval, char *key,char *strval, uint strlen, int dup); add_assoc_zval(zval *aval, char *key, zval *value); //备注:其实这些函数都是宏,都是对add_assoc_*_ex函数的封装。 //add_index_*系列函数: ZEND_API int add_index_long (zval *arg, ulong idx, long n); ZEND_API int add_index_null (zval *arg, ulong idx ); ZEND_API int add_index_bool (zval *arg, ulong idx, int b ); ZEND_API int add_index_resource (zval *arg, ulong idx, int r ); ZEND_API int add_index_double (zval *arg, ulong idx, double d); ZEND_API int add_index_string (zval *arg, ulong idx, const char *str, int duplicate); ZEND_API int add_index_stringl (zval *arg, ulong idx, const char *str, uint length, int duplicate); ZEND_API int add_index_zval (zval *arg, ulong index, zval *value); //add_next_index_*函数: ZEND_API int add_next_index_long (zval *arg, long n ); ZEND_API int add_next_index_null (zval *arg ); ZEND_API int add_next_index_bool (zval *arg, int b ); ZEND_API int add_next_index_resource (zval *arg, int r ); ZEND_API int add_next_index_double (zval *arg, double d); ZEND_API int add_next_index_string (zval *arg, const char *str, int duplicate); ZEND_API int add_next_index_stringl (zval *arg, const char *str, uint length, int duplicate); ZEND_API int add_next_index_zval (zval *arg, zval *value);
Copy after loginBelow you can compare the macros corresponding to common array operations.
add_next_index_*()
PHP中 内核中 $arr[] = NULL; add_next_index_null(arr); $arr[] = 42; add_next_index_long(arr, 42); $arr[] = true; add_next_index_bool(arr, 1); $arr[] = 3.14; add_next_index_double(arr, 3.14); $arr[] = 'foo'; add_next_index_string(arr, "foo"); $arr[] = $var; add_next_index_zval(arr, zval);
Copy after login
add_index_*()PHP中 内核中 $arr[0] = NULL; add_index_null(arr, 0); $arr[1] = 42; add_index_long(arr, 1, 42); $arr[2] = true; add_index_bool(arr, 2, 1); $arr[3] = 3.14; add_index_double(arr, 3, 3.14); $arr[4] = 'foo'; add_index_string(arr, 4, "foo", 1); $arr[5] = $var; add_index_zval(arr, 5, zval);
Copy after login
add_assoc_*()PHP中 内核中 $arr["abc"] = NULL; add_assoc_null(arr, "abc"); $arr["def"] = 42; add_assoc_long(arr, "def", 42); $arr["ghi"] = true; add_assoc_bool(arr, "ghi", 1); $arr["jkl"] = 3.14 add_assoc_double(arr, "jkl", 3.14); $arr["mno"]="foo" add_assoc_string(arr, "mno", "foo", 1"); $arr["pqr"] = $var; add_assoc_zval(arr, "pqr", zval);
Copy after login
A complete example
Next define a function in PHP and use an array in it. Then let's see how to implement it in the kernel.
<?php function array_test(){ $mystr = "Forty Five"; $return_value = array(); $return_value[42] = 123; $return_value[] = "test"; $return_value[] = $mystr; $return_value["double"] = 3.14; $mysubarray; $mysubarray = array(); $mysubarray[] = "nowamagic"; $return_value["subarray"] = $mysubarray; return $return_value; } ?>
Copy after login
Implemented in the kernel:PHP_FUNCTION(array_test){ char* mystr; zval* mysubarray; array_init(return_value); add_index_long(return_value, 42, 123); add_next_index_string(return_value, "test", 1); add_next_index_stringl(return_value, "test_stringl", 10, 1); mystr = estrdup("Forty Five"); add_next_index_string(return_value, mystr, 0); add_assoc_double(return_value, "double", 3.14); ALLOC_INIT_ZVAL(mysubarray); array_init(mysubarray); add_next_index_string(mysubarray, "hello", 1); add_assoc_zval(return_value, "subarray", mysubarray); }
Copy after login
You may wonder where the variable return_value in the above code is defined. Expand PHP_FUNCTION below and you will understand.zif_array_test(int ht, zval *return_value, zval **return_value_ptr, zval *this_ptr, int return_value_used TSRMLS_DC);
Copy after login
That's right, in fact every function has a default return value return_value. When using RETVAL_*(), RETURN_*() as the function return value, only the return_value is modified.

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
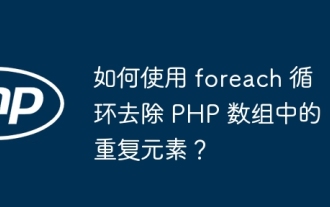
The method of using a foreach loop to remove duplicate elements from a PHP array is as follows: traverse the array, and if the element already exists and the current position is not the first occurrence, delete it. For example, if there are duplicate records in the database query results, you can use this method to remove them and obtain results without duplicate records.
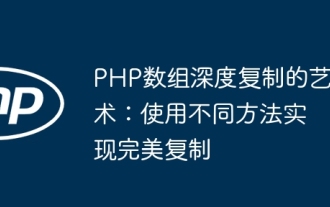
Methods for deep copying arrays in PHP include: JSON encoding and decoding using json_decode and json_encode. Use array_map and clone to make deep copies of keys and values. Use serialize and unserialize for serialization and deserialization.

The performance comparison of PHP array key value flipping methods shows that the array_flip() function performs better than the for loop in large arrays (more than 1 million elements) and takes less time. The for loop method of manually flipping key values takes a relatively long time.
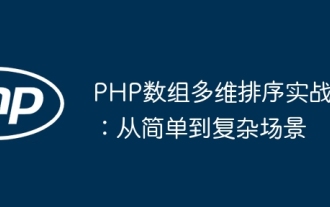
Multidimensional array sorting can be divided into single column sorting and nested sorting. Single column sorting can use the array_multisort() function to sort by columns; nested sorting requires a recursive function to traverse the array and sort it. Practical cases include sorting by product name and compound sorting by sales volume and price.
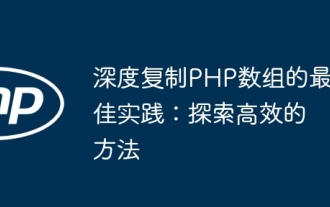
The best practice for performing an array deep copy in PHP is to use json_decode(json_encode($arr)) to convert the array to a JSON string and then convert it back to an array. Use unserialize(serialize($arr)) to serialize the array to a string and then deserialize it to a new array. Use the RecursiveIteratorIterator to recursively traverse multidimensional arrays.
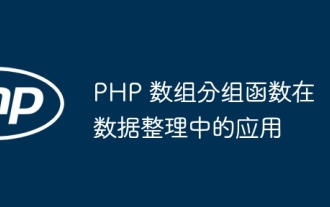
PHP's array_group_by function can group elements in an array based on keys or closure functions, returning an associative array where the key is the group name and the value is an array of elements belonging to the group.
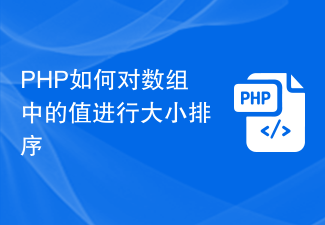
PHP is a commonly used server-side scripting language widely used in website development and data processing fields. In PHP, it is a very common requirement to sort the values in an array by size. By using the built-in sort function, you can easily sort arrays. The following will introduce how to use PHP to sort the values in an array by size, with specific code examples: 1. Sort the values in the array in ascending order:
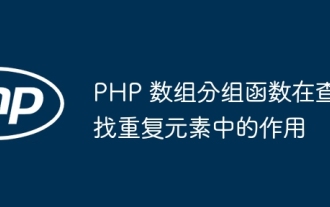
PHP's array_group() function can be used to group an array by a specified key to find duplicate elements. This function works through the following steps: Use key_callback to specify the grouping key. Optionally use value_callback to determine grouping values. Count grouped elements and identify duplicates. Therefore, the array_group() function is very useful for finding and processing duplicate elements.
