


Detailed explanation of PHP (7) object-oriented programming_PHP tutorial
Elaborate on php(7) object-oriented programming
1. Class declaration and object initialization
1.1 When declaring member attributes in a class: There must be a modifier in front of it. When you don’t know which one to use, use var. If you know which modifier keyword to use, don’t use var
var $color;
var $name = "zhangsan"
1.2 A file only saves one class, and the file name contains the class name, such as: class name.class.php
person.class.php
1.3 Use the new keyword to create an object. Once an object is created, a space is allocated in the memory. $Object reference = new class name;
$person = new Person
<?php class Person { var $name; // Java: private String name; var $age; var $sex; function say() { echo $this->name; } } $p1 = new Person; // Java: Person person = new Person; $p1->name = "lisi"; // Java: person.name = "lisi"; $p1->say(); // Java: person.say(); ?>
a. Stack memory: stores local variables
b. Heap memory: storage object
c. Shared area: store static variables
d. Code segment: storage methods, etc.
2. Constructor and destructor
2.1 Constructor:
a. The constructor method is the first method that is automatically called after the object is created
b. In PHP4, the method with the same name as the class is the constructor
c. In PHP5, the constructor chooses to use the magic method __construct(). This name is used to declare constructors in all classes
Advantages: The constructor method does not need to be changed when changing the class name
d. The role of the constructor: initialize member properties
<?php class Person { var $name; var $age; var $sex; function __construct($name="", $age=0, $sex="男"){ $this->name=$name; $this->age=$age; $this->sex=$sex; } function say(){ echo "我的名子:{$this->name},我的年龄:{$this->age},我的性别:{$this->sex}。<br>"; } } $p1=new Person("zhangsan", 20, "女"); $p2=new Person("lisi", 25); $p3=new Person("wangwu"); $p1->say(); $p2->say(); $p3->say(); ?>
2.2 Destructor:
a. The destructor refers to the last method that is automatically called before the object is released
b. Like Java, PHP also uses a garbage collector to release resources, but PHP does so immediately after the call, while Java does not.
c. The role of the destructor: close some resources, do some cleanup work, use the magic method __destruct()
<?php class Person { var $name; var $age; var $sex; function __construct($name="", $age=0, $sex="男"){ $this->name=$name; $this->age=$age; $this->sex=$sex; } function say(){ echo "我的名子:{$this->name},我的年龄:{$this->age},我的性别:{$this->sex}。<br>"; } function __destruct(){ echo $this->name."再见!<br>"; } } $p1=new Person("zhangsan", 20, "女"); $p1->say(); $p1 = null; // 我的名子:zhangsan,我的年龄:20,我的性别:女。 // zhangsan再见! ?>
Magic methods are methods provided by the system that are automatically called at different times to complete a certain function. Different magic methods have different calling times
Magic methods start with __
__construct(); // Constructor
__destruct(); // Destructor
__set();
__get();
__isset();
__unset();
__clone();
__call();
__sleep();
__weakup();
__toString()
__autoload();

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
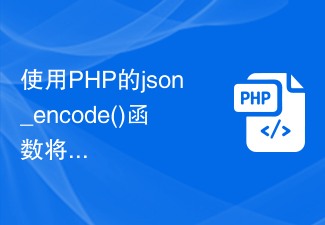
JSON (JavaScriptObjectNotation) is a lightweight data exchange format that has become a common format for data exchange between web applications. PHP's json_encode() function can convert an array or object into a JSON string. This article will introduce how to use PHP's json_encode() function, including syntax, parameters, return values, and specific examples. Syntax The syntax of the json_encode() function is as follows: st
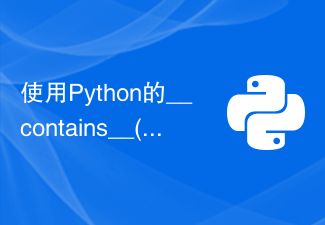
Use Python's __contains__() function to define the containment operation of an object. Python is a concise and powerful programming language that provides many powerful features to handle various types of data. One of them is to implement the containment operation of objects by defining the __contains__() function. This article will introduce how to use the __contains__() function to define the containment operation of an object, and give some sample code. The __contains__() function is Pytho
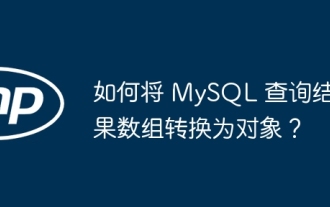
Here's how to convert a MySQL query result array into an object: Create an empty object array. Loop through the resulting array and create a new object for each row. Use a foreach loop to assign the key-value pairs of each row to the corresponding properties of the new object. Adds a new object to the object array. Close the database connection.

Wedge We know that objects are created in two main ways, one is through Python/CAPI, and the other is by calling a type object. For instance objects of built-in types, both methods are supported. For example, lists can be created through [] or list(). The former is Python/CAPI and the latter is a calling type object. But for instance objects of custom classes, we can only create them by calling type objects. If an object can be called, then the object is callable, otherwise it is not callable. Determining whether an object is callable depends on whether a method is defined in its corresponding type object. like
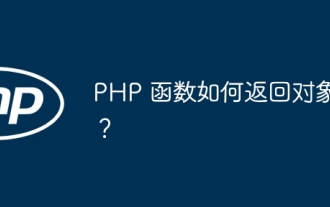
PHP functions can encapsulate data into a custom structure by returning an object using a return statement followed by an object instance. Syntax: functionget_object():object{}. This allows creating objects with custom properties and methods and processing data in the form of objects.
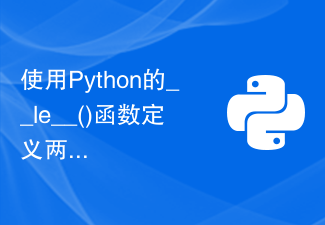
Title: Using Python's __le__() function to define a less than or equal comparison of two objects In Python, we can define comparison operations between objects by using special methods. One of them is the __le__() function, which is used to define less than or equal comparisons. The __le__() function is a magic method in Python and is a special function used to implement the "less than or equal" operation. When we compare two objects using the less than or equal operator (<=), Python
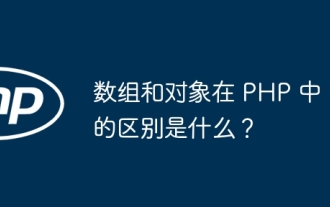
In PHP, an array is an ordered sequence, and elements are accessed by index; an object is an entity with properties and methods, created through the new keyword. Array access is via index, object access is via properties/methods. Array values are passed and object references are passed.
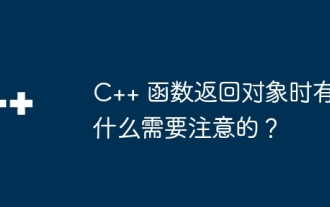
In C++, there are three points to note when a function returns an object: The life cycle of the object is managed by the caller to prevent memory leaks. Avoid dangling pointers and ensure the object remains valid after the function returns by dynamically allocating memory or returning the object itself. The compiler may optimize copy generation of the returned object to improve performance, but if the object is passed by value semantics, no copy generation is required.
