Python: Efficient Ways to Merge Two Lists
There are many ways to merge Python lists: 1. Use operators, which are simple but not memory efficient for large lists; 2. Use extend method, which is efficient but will modify the original list; 3. Use itertools.chain, which is suitable for large data sets; 4. Use * operator, which is merged into small to medium-sized lists in one line of code; 5. Use numpy.concatenate, which is suitable for large data sets and scenarios with high performance requirements; 6. Use append method, which is suitable for small lists but is inefficient. When selecting a method, you need to consider the list size and application scenarios.
When it comes to merge two lists in Python, there's more than one way to skin this cat. Let me walk you through some efficient methods, sharing my insights and experiences along the way.
Let's dive in with a simple yet effective approach: using the
operator. This is straightforward and intuitive, but it's not always the most memory-efficient for large lists. Here's how you do it:
list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = list1 list2 print(merged_list) # Output: [1, 2, 3, 4, 5, 6]
This method creates a new list by concatenating list1
and list2
. It's simple, but if you're dealing with massive lists, you might want to consider alternatives that don't involve creating a new list in memory all at once.
Another approach is to use the extend
method, which modifies the original list in-place. This can be more memory-efficient because it doesn't create a new list:
list1 = [1, 2, 3] list2 = [4, 5, 6] list1.extend(list2) print(list1) # Output: [1, 2, 3, 4, 5, 6]
I've used extend
in situations where memory was a concern, and it's a handy tool to have in your belt. Just remember that it modifies the original list, so if you need to keep list1
unchanged, you'll need to make a copy first.
Now, let's talk about a method that's both efficient and flexible: list comprehension with itertools.chain
. This is a bit more advanced but incredibly powerful:
import itertools list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = list(itertools.chain(list1, list2)) print(merged_list) # Output: [1, 2, 3, 4, 5, 6]
Using itertools.chain
allows you to lazy iterate over multiple sequences without creating intermediate lists. It's especially useful when you're dealing with generators or other iterable objects. I once used this in a project where I had to process large datasets, and it saved me a ton of memory.
For those who love one-liners, here's another approach using the *
operator to unpack the lists:
list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = [*list1, *list2] print(merged_list) # Output: [1, 2, 3, 4, 5, 6]
This method is concise and works well for small to medium-sized lists. It's a bit of a hidden gem in Python, and I've found it to be quite handy when I need to merge lists quickly without thinking too much about it.
Now, let's talk about performance. If you're dealing with large lists, you might want to consider using numpy
arrays instead of Python lists. Here's how you can merge two numpy
arrays:
import numpy as np array1 = np.array([1, 2, 3]) array2 = np.array([4, 5, 6]) merged_array = np.concatenate((array1, array2)) print(merged_array) # Output: [1 2 3 4 5 6]
numpy
is designed for numerical operations and is much more efficient than Python lists for large datasets. I've used this approach in data science projects where performance was critical, and it made a noticeable difference.
Let's not forget about the append
method, which can be used in a loop to merge lists. While this isn't the most efficient for large lists, it's simple and works well for small ones:
list1 = [1, 2, 3] list2 = [4, 5, 6] merged_list = [] for item in list1 list2: merged_list.append(item) print(merged_list) # Output: [1, 2, 3, 4, 5, 6]
This method is straightforward but can be slow for large lists due to the overhead of the loop and the append
method. I've used this in situations where readability was more important than performance, but it's not my go-to for large datasets.
Finally, let's discuss some best practices and potential pitfalls. When merging lists, always consider the size of your lists and the context of your application. For small lists, the
operator or the *
operator might be fine, but for larger lists, you'll want to use more memory-efficient methods like extend
or itertools.chain
.
One pitfall to watch out for is the modification of the original list when using extend
. If you need to keep the original lists intact, make sure to create a copy before merge. Another thing to consider is the type of data you're working with. If you're dealing with numerical data, numpy
might be a better choice than Python lists.
In conclusion, merging lists in Python can be done in various ways, each with its own trade-offs. By understanding these methods and their implications, you can choose the most efficient approach for your specific use case. Whether you're dealing with small lists or massive datasets, there's a method out there that's just right for you.
The above is the detailed content of Python: Efficient Ways to Merge Two Lists. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










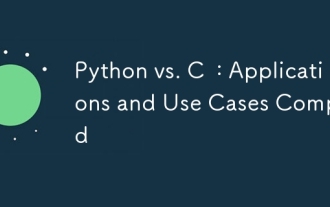
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
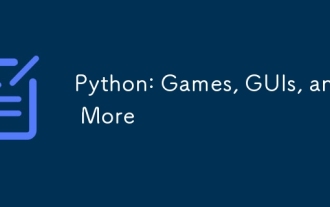
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
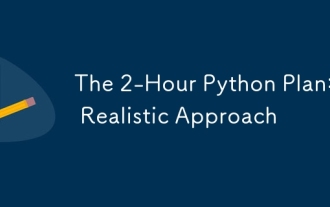
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
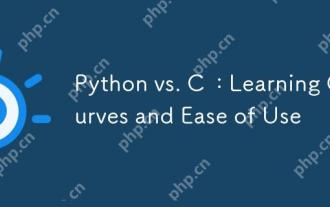
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
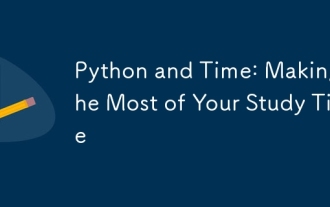
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
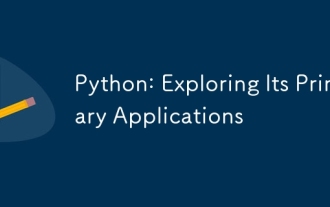
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
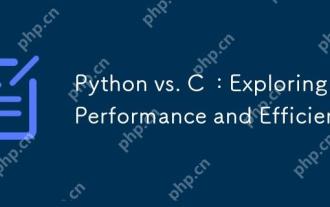
Python is better than C in development efficiency, but C is higher in execution performance. 1. Python's concise syntax and rich libraries improve development efficiency. 2.C's compilation-type characteristics and hardware control improve execution performance. When making a choice, you need to weigh the development speed and execution efficiency based on project needs.
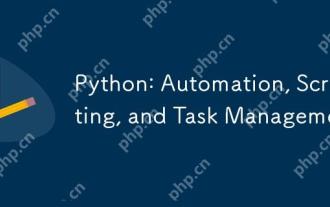
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
