React's View-Focused Nature: Managing Complex Application State
React's view focus manages complex application state by introducing additional tools and patterns. 1) React itself does not handle state management, and focuses on mapping states to views. 2) Complex applications need to use such as Redux, MobX, or Context APIs to decouple states, making management more structured and predictable.
React's view focus: Manage complex application states
When we talk about React, it's hard not to mention its focus on view layers. React greatly simplifies the UI construction process through its componentized and declarative features. But things can get a little tricky when we face complex application state management. So, how does React's view focus affect our management of complex application states?
First of all, React itself does not directly deal with state management, it focuses more on how to efficiently map state to views. This means that when building complex applications, we need to introduce additional tools and patterns to manage state, such as Redux, MobX, or Context APIs. These tools help us decouple states from components, making state management more structured and predictable.
Let's dive into how to manage complex application states in React, as well as some of the challenges and solutions you may encounter in the process.
In React, the basic concept of state management is to save data in the state of a component and update it through setState
method. For simple applications, this method is sufficient. However, when the application becomes complex and states begin to be shared among different components, relying solely on state management within the component becomes unscrupulous.
Let's look at a simple example, suppose we have a shopping cart app where users can add and delete items:
import React, { useState } from 'react'; function CartItem({ item, onRemove }) { Return ( <div> {item.name} - ${item.price} <button onClick={() => onRemove(item.id)}>Remove</button> </div> ); } function ShoppingCart() { const [items, setItems] = useState([]); const addItem = (item) => { setItems([...items, item]); }; const removeItem = (id) => { setItems(items.filter(item => item.id !== id)); }; Return ( <div> <h2 id="Shopping-Cart">Shopping Cart</h2> {items.map(item => ( <CartItem key={item.id} item={item} onRemove={removeItem} /> ))} <button onClick={() => addItem({ id: Date.now(), name: 'New Item', price: 10 })}>Add Item</button> </div> ); }
In this example, ShoppingCart
component manages the status of the entire shopping cart. However, things get complicated if we need to access or modify this state in other components.
To solve this problem, we can use React's Context API, which allows us to elevate state to a higher level of the component tree, allowing child components to access and modify it. Let's see how to use Context to manage the status of your shopping cart:
import React, { createContext, useContext, useState } from 'react'; const CartContext = createContext(); function CartProvider({ children }) { const [items, setItems] = useState([]); const addItem = (item) => { setItems([...items, item]); }; const removeItem = (id) => { setItems(items.filter(item => item.id !== id)); }; Return ( <CartContext.Provider value={{ items, addItem, removeItem }}> {children} </CartContext.Provider> ); } function useCart() { return useContext(CartContext); } function CartItem({ item }) { const { removeItem } = useCart(); Return ( <div> {item.name} - ${item.price} <button onClick={() => removeItem(item.id)}>Remove</button> </div> ); } function ShoppingCart() { const { items, addItem } = useCart(); Return ( <div> <h2 id="Shopping-Cart">Shopping Cart</h2> {items.map(item => ( <CartItem key={item.id} item={item} /> ))} <button onClick={() => addItem({ id: Date.now(), name: 'New Item', price: 10 })}>Add Item</button> </div> ); } function App() { Return ( <CartProvider> <ShoppingCart /> </CartProvider> ); }
By using Context, we can promote the state of the shopping cart to the CartProvider
component, so that any child component can access and modify the state of the shopping cart through useCart
hook.
However, the Context API may encounter some problems when dealing with complex states, such as performance issues of state updates and complexity of state management. To solve these problems, we can introduce more powerful state management libraries such as Redux or MobX.
Let's take a look at how to use Redux to manage the status of your shopping cart:
import React from 'react'; import { createStore } from 'redux'; import { Provider, useSelector, useDispatch } from 'react-redux'; // Define state and operation const initialState = { Items: [] }; const ADD_ITEM = 'ADD_ITEM'; const REMOVE_ITEM = 'REMOVE_ITEM'; function cartReducer(state = initialState, action) { switch (action.type) { case ADD_ITEM: return { ...state, items: [...state.items, action.payload] }; case REMOVE_ITEM: return { ...state, items: state.items.filter(item => item.id !== action.payload) }; default: return state; } } const store = createStore(cartReducer); function CartItem({ item }) { const dispatch = useDispatch(); Return ( <div> {item.name} - ${item.price} <button onClick={() => dispatch({ type: REMOVE_ITEM, payload: item.id })}>Remove</button> </div> ); } function ShoppingCart() { const items = useSelector(state => state.items); const dispatch = useDispatch(); Return ( <div> <h2 id="Shopping-Cart">Shopping Cart</h2> {items.map(item => ( <CartItem key={item.id} item={item} /> ))} <button onClick={() => dispatch({ type: ADD_ITEM, payload: { id: Date.now(), name: 'New Item', price: 10 } })}>Add Item</button> </div> ); } function App() { Return ( <Provider store={store}> <ShoppingCart /> </Provider> ); }
Using Redux, we can completely decouple state management from the components and manage the state of the application through a centralized store. This approach makes state management more predictable and maintainable, but also increases the complexity and learning curve of the application.
In practical applications, which state management solution to choose depends on the complexity of the application and the team's technology stack. Whether using the Context API or Redux, they need to be weighed.
The advantage of the Context API is that it is built-in from React, has low learning costs, and is suitable for small and medium-sized applications. But its disadvantages are performance issues and the complexity of state management can become difficult to deal with in complex applications.
The advantage of Redux is that it provides a powerful state management mechanism suitable for large applications and complex state management needs. But its disadvantage is that the learning curve is steep and may increase the complexity of the application.
There are also some common pitfalls and best practices to be aware of when using these state management scenarios. For example, when using the Context API, avoid overuse of Context, as this may lead to unnecessary re-rendering. When using Redux, be careful to avoid overuse of actions and reducers, as this may make state management too complicated.
Overall, React's view focus makes it necessary to introduce additional tools and patterns when dealing with complex application states. By rationally selecting and using these tools, we can effectively manage the state of complex applications and build efficient and maintainable applications.
The above is the detailed content of React's View-Focused Nature: Managing Complex Application State. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
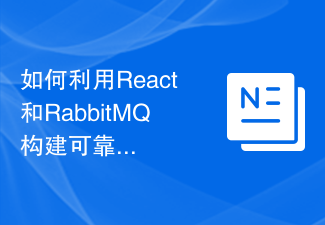
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
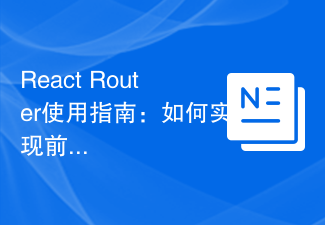
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
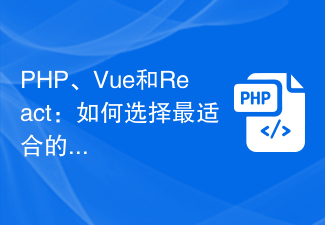
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
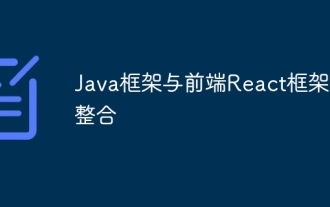
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
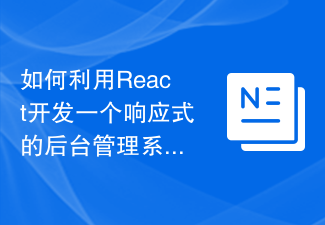
How to use React to develop a responsive backend management system. With the rapid development of the Internet, more and more companies and organizations need an efficient, flexible, and easy-to-manage backend management system to handle daily operations. As one of the most popular JavaScript libraries currently, React provides a concise, efficient and maintainable way to build user interfaces. This article will introduce how to use React to develop a responsive backend management system and give specific code examples. Create a React project first

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
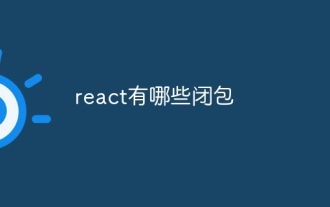
React has closures such as event handling functions, useEffect and useCallback, higher-order components, etc. Detailed introduction: 1. Event handling function closure: In React, when we define an event handling function in a component, the function will form a closure and can access the status and properties within the component scope. In this way, the state and properties of the component can be used in the event processing function to implement interactive logic; 2. Closures in useEffect and useCallback, etc.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
