How do you remove elements from a Python array?
Python lists can be manipulated using several methods to remove elements: 1) The remove() method removes the first occurrence of a specified value. 2) The pop() method removes and returns an element at a given index. 3) The del statement can remove an item or slice by index. 4) List comprehensions create a new list excluding elements meeting certain criteria. 5) The filter() function creates a new list based on a test condition, similar to list comprehensions but potentially more readable for complex conditions.
When it comes to removing elements from a Python array, we're actually talking about lists, as Python doesn't have a built-in array type like some other languages. Lists in Python are versatile and offer several methods to remove elements, each with its own quirks and use cases. Let's dive into the different ways to do this and explore some of the nuances.
Removing elements from a list can be as straightforward as using the remove()
method, or as nuanced as using list comprehensions or slices. I've been working with Python for years, and I've found that understanding these methods deeply can save you from common pitfalls and help you write more efficient code.
Let's look at the various ways to remove elements from a Python list:
Using the remove()
Method
The remove()
method is probably the most intuitive way to remove an element. It searches for the first occurrence of the specified value and removes it.
my_list = [1, 2, 3, 2, 4] my_list.remove(2) print(my_list) # Output: [1, 3, 2, 4]
This method is great for simple cases, but be aware that it only removes the first occurrence of the specified value. If you need to remove all occurrences, you'll need to use a loop or a different approach.
Using the pop()
Method
The pop()
method removes and returns the element at the specified index. If no index is provided, it removes and returns the last element.
my_list = [1, 2, 3, 4] removed_element = my_list.pop(1) print(removed_element) # Output: 2 print(my_list) # Output: [1, 3, 4]
pop()
is useful when you need to know what element you're removing, but it's less efficient for large lists because it involves shifting elements.
Using the del
Statement
The del
statement can remove an item at a specific index or a slice of the list.
my_list = [1, 2, 3, 4] del my_list[1] print(my_list) # Output: [1, 3, 4] my_list = [1, 2, 3, 4, 5] del my_list[1:3] print(my_list) # Output: [1, 4, 5]
del
is versatile and can be used to remove single elements or slices. It's particularly useful when you know the index of the element you want to remove.
Using List Comprehensions
List comprehensions offer a concise way to create a new list with elements that meet certain criteria, effectively filtering out unwanted elements.
my_list = [1, 2, 3, 4, 5] new_list = [x for x in my_list if x != 3] print(new_list) # Output: [1, 2, 4, 5]
This method is great for readability and can be more efficient for large lists, especially when you need to remove elements based on a condition.
Using the filter()
Function
The filter()
function can be used to create a new list with elements that pass a certain test.
my_list = [1, 2, 3, 4, 5] new_list = list(filter(lambda x: x != 3, my_list)) print(new_list) # Output: [1, 2, 4, 5]
filter()
is similar to list comprehensions but can be more readable for complex conditions.
Considerations and Best Practices
-
Performance: For large lists, using
remove()
orpop()
can be slow because they shift elements. List comprehensions orfilter()
are generally more efficient. -
Multiple Occurrences: If you need to remove all occurrences of a value, avoid using
remove()
alone. Instead, use a loop or list comprehension. -
Indexing: When using
pop()
ordel
, be careful with indices, especially if you're removing multiple elements in a loop. -
Readability: List comprehensions and
filter()
can make your code more readable and maintainable, especially for complex filtering logic.
In my experience, choosing the right method depends on the specific requirements of your task. For simple cases, remove()
or del
might suffice. For more complex scenarios or when performance is a concern, list comprehensions or filter()
are often better choices.
Remember, the key to mastering Python lists is understanding the trade-offs between different methods. Experiment with these techniques in your projects, and you'll develop a keen sense of when to use each one effectively.
The above is the detailed content of How do you remove elements from a Python array?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
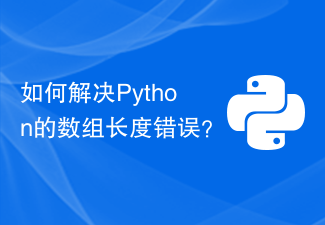
Python is a high-level programming language widely used in fields such as data analysis and machine learning. Among them, array is one of the commonly used data structures in Python, but during the development process, array length errors are often encountered. This article will detail how to solve Python's array length error. Length of Array First, we need to know the length of the array. In Python, the length of an array can vary, that is, we can modify the length of the array by adding or removing elements from the array. because
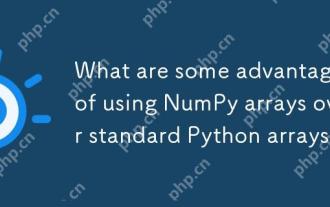
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making
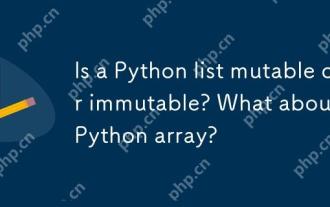
Pythonlistsandarraysarebothmutable.1)Listsareflexibleandsupportheterogeneousdatabutarelessmemory-efficient.2)Arraysaremorememory-efficientforhomogeneousdatabutlessversatile,requiringcorrecttypecodeusagetoavoiderrors.
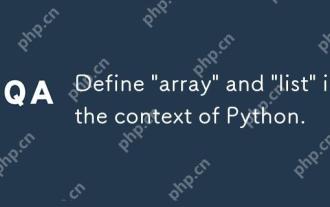
InPython,a"list"isaversatile,mutablesequencethatcanholdmixeddatatypes,whilean"array"isamorememory-efficient,homogeneoussequencerequiringelementsofthesametype.1)Listsareidealfordiversedatastorageandmanipulationduetotheirflexibility
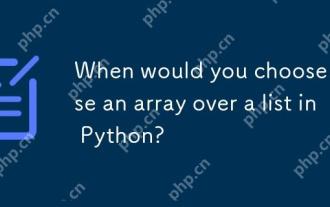
Useanarray.arrayoveralistinPythonwhendealingwithhomogeneousdata,performance-criticalcode,orinterfacingwithCcode.1)HomogeneousData:Arrayssavememorywithtypedelements.2)Performance-CriticalCode:Arraysofferbetterperformancefornumericaloperations.3)Interf

WhenyouattempttostoreavalueofthewrongdatatypeinaPythonarray,you'llencounteraTypeError.Thisisduetothearraymodule'sstricttypeenforcement,whichrequiresallelementstobeofthesametypeasspecifiedbythetypecode.Forperformancereasons,arraysaremoreefficientthanl
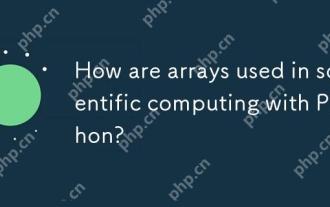
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
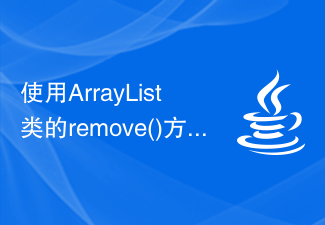
Remove elements from an array list in Java using the remove() method of the ArrayList class In Java, an ArrayList is a dynamic array that can automatically grow and shrink as needed. It is implemented using generics so it can store any type of object. The ArrayList class provides many methods to operate on array lists, including methods for removing elements. One of the commonly used methods is the remove() method, which can remove an array column based on the index or instance of the element.
