Python vs. JavaScript: Performance and Efficiency Considerations
The differences in performance and efficiency between Python and JavaScript are mainly reflected in: 1) As an interpreted language, Python runs slowly but has high development efficiency and is suitable for rapid prototype development; 2) JavaScript is limited to single thread in the browser, but multi-threading and asynchronous I/O can be used to improve performance in Node.js, and both have advantages in actual projects.
introduction
In the programming world, Python and JavaScript are often compared as two mainstream languages. Today we delve into the differences in performance and efficiency between the two languages. This topic is not only about speed competition, but also about how to choose the most suitable tool in the actual project. Through this article, you will learn about the performance of Python and JavaScript, and how to optimize their use in development.
Review of basic knowledge
Python and JavaScript are both high-level programming languages, but they are different in their original design intentions and application scenarios. Python is known for its concise syntax and rich library ecosystem, and is commonly used in data science, machine learning, and backend development. JavaScript is known for its widespread use in browsers and has also shined in recent years in areas such as server side (Node.js) and mobile development (React Native).
Core concept or function analysis
Performance comparison between Python and JavaScript
In terms of performance, Python and JavaScript have their own advantages. As an interpreted language, Python runs relatively slowly, but its development efficiency is high and suitable for rapid prototype development. When JavaScript is run in the browser, it is limited to a single-threaded environment, but the emergence of Node.js makes its performance on the server side quite impressive.
Python performance
Python's performance bottleneck is mainly its interpreted characteristics, and the source code needs to be interpreted every execution, which increases the run time. Here is a simple Python code example showing its execution speed:
import time <p>start_time = time.time()</p><p> result = 0 for i in range(1000000): result = i</p><p> end_time = time.time() print(f"Execution time: {end_time - start_time} seconds")</p>
This loop can take several milliseconds to tens of milliseconds to run in Python, depending on your hardware environment.
Performance of JavaScript
JavaScript is limited to single threading when running in the browser, but the optimization of the V8 engine makes its performance good. In Node.js, JavaScript can further improve performance by leveraging multi-threading and asynchronous I/O. Here is a similar JavaScript code example:
const start_time = performance.now(); <p>let result = 0; for (let i = 0; i </p><p> const end_time = performance.now(); console.log( <code>Execution time: ${(end_time - start_time) / 1000} seconds</code> );</p>
In Node.js, the execution time of this loop is usually faster than that of Python, but the specific time will also be affected by the hardware and environment.
How it works
When the Python interpreter executes the code, it compiles the source code into bytecode and then executes it by the virtual machine. Although this method is flexible, it increases execution time. JavaScript is directly compiled into machine code by the engine (such as V8) in the browser, and has high execution efficiency. In Node.js, JavaScript can utilize event loops and asynchronous I/O to improve concurrent processing capabilities.
Example of usage
Basic usage of Python
Python's concise syntax makes development efficient. Here is a simple file reading example:
with open('example.txt', 'r') as file: content = file.read() print(content)
This method is simple and easy to understand, but if the file is large, it may cause excessive memory usage.
Basic usage of JavaScript
JavaScript is very convenient when handling DOM operations in the browser. Here is a simple example of DOM operations:
document.getElementById('myButton').addEventListener('click', function() { alert('Button clicked!'); });
This method directly operates the DOM, which has better performance, but you need to pay attention to avoid frequent DOM operations to avoid affecting the user experience.
Advanced Usage
Advanced usage of Python
Advanced usage of Python includes using generators and asynchronous programming, and here is an example using asynchronous I/O:
import asyncio <p>async def fetch_data(): await asyncio.sleep(1) # simulate I/O operation return "Data fetched!"</p><p> async def main(): tasks = [fetch <em>data() for</em> in range(10)] results = await asyncio.gather(*tasks) print(results)</p><p> asyncio.run(main())</p>
This approach can significantly improve the performance of I/O-intensive tasks, but attention needs to be paid to the complexity of asynchronous programming.
Advanced usage of JavaScript
Advanced usage of JavaScript includes using Promise and async/await. Here is an example of using Promise:
function fetchData() { return new Promise(resolve => { setTimeout(() => { resolve("Data fetched!"); }, 1000); }); } <p>async function main() { const results = await Promise.all(Array(10).fill().map(fetchData)); console.log(results); }</p><p> main();</p>
This method can effectively utilize the asynchronous features of JavaScript to improve concurrent processing capabilities.
Common Errors and Debugging Tips
Common performance issues in Python include improper use of global variables and excessive use of list comprehensions. Here is an example of a common error:
# Error example: Overuse of list comprehension numbers = [i for i in range(1000000)] squares = [i**2 for i in numbers]
This method will cause excessive memory usage, and the improvement is to use the generator:
# Improved example: Use generator numbers = (i for i in range(1000000)) squares = (i**2 for i in numbers)
Common performance issues in JavaScript include frequent DOM operations and improper use of closures. Here is an example of a common error:
// Error example: Frequent DOM operations for (let i = 0; i <p>This method can lead to performance degradation, and the improvement is to use document fragmentation:</p><pre class="brush:php;toolbar:false"> // Improved example: Use document fragmentation const fragment = document.createDocumentFragment(); for (let i = 0; i <h2 id="Performance-optimization-and-best-practices">Performance optimization and best practices</h2><h3 id="Performance-optimization-of-Python"> Performance optimization of Python</h3><p> Python performance optimization can be started from the following aspects:</p>
- Using Cython or PyPy: Cython can compile Python code into C language. PyPy is a JIT compiler that can significantly improve Python's running speed.
- Avoid global variables: Global variables will cause performance degradation, try to use local variables.
- Using appropriate data structures: For example, using
set
instead oflist
for member testing can significantly improve performance.
Here is an example of using Cython optimization:
# cython: language_level=3 <p>cdef int sum_range(int n): cdef int result = 0 for i in range(n): result = i return result</p><p> print(sum_range(1000000))</p>
This method can increase the execution speed of Python code to a level close to that of C, but it should be noted that Cython's learning curve is steep.
Performance optimization of JavaScript
JavaScript performance optimization can be started from the following aspects:
- Using Web Workers: Web Workers can move compute-intensive tasks out of the main thread to improve user experience.
- Reduce DOM operations: Minimize DOM operations, use document fragmentation or batch operations.
- Optimize event listening: Avoid too many global event listening, using event delegation can improve performance.
Here is an example of using Web Workers:
// main.js const worker = new Worker('worker.js'); worker.postMessage({cmd: 'start', data: 1000000}); <p>worker.onmessage = function(e) { console.log('Result:', e.data); };</p><p> // worker.js self.onmessage = function(e) { if (e.data.cmd === 'start') { let result = 0; for (let i = 0; i </p>
This method can move compute-intensive tasks out of the main thread to avoid blocking the user interface, but attention should be paid to the communication overhead of Web Workers.
Best Practices
Whether it is Python or JavaScript, the key to writing efficient code lies in understanding language features and application scenarios. Here are some best practices:
- Code readability: Whether it is Python or JavaScript, it is crucial to keep the code readable. Using meaningful variable names, appropriate comments and reasonable code structure can greatly improve the maintenance of the code.
- Performance testing: Before optimizing the code, performance testing is necessary. Using Python's
timeit
module or JavaScript'sconsole.time()
can help you find performance bottlenecks. - Code reuse: Try to reuse existing code and libraries to avoid duplicate wheels. Python's
functools
and JavaScript'slodash
are both very useful tool libraries.
In actual projects, choosing Python or JavaScript depends on your specific needs. If your project involves data processing, scientific computing, or backend development, Python may be a better choice. If your project involves front-end development, real-time applications, or cross-platform development, JavaScript may be more suitable.
In short, the performance and efficiency differences between Python and JavaScript need to be evaluated based on the specific application scenario. Through this article's discussion, I hope you can better understand the characteristics of these two languages and make smarter choices in actual development.
The above is the detailed content of Python vs. JavaScript: Performance and Efficiency Considerations. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
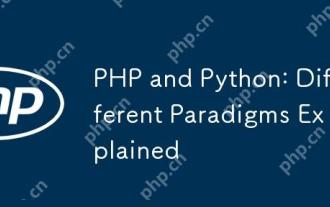
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
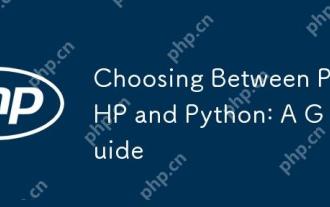
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
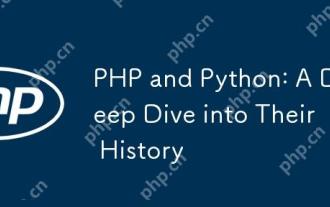
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
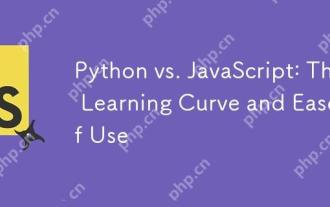
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
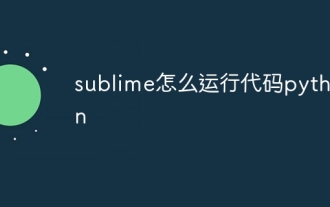
To run Python code in Sublime Text, you need to install the Python plug-in first, then create a .py file and write the code, and finally press Ctrl B to run the code, and the output will be displayed in the console.
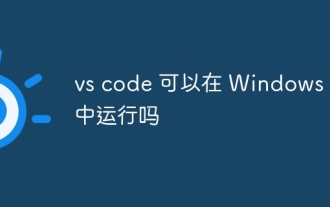
VS Code can run on Windows 8, but the experience may not be great. First make sure the system has been updated to the latest patch, then download the VS Code installation package that matches the system architecture and install it as prompted. After installation, be aware that some extensions may be incompatible with Windows 8 and need to look for alternative extensions or use newer Windows systems in a virtual machine. Install the necessary extensions to check whether they work properly. Although VS Code is feasible on Windows 8, it is recommended to upgrade to a newer Windows system for a better development experience and security.
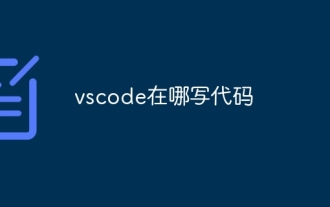
Writing code in Visual Studio Code (VSCode) is simple and easy to use. Just install VSCode, create a project, select a language, create a file, write code, save and run it. The advantages of VSCode include cross-platform, free and open source, powerful features, rich extensions, and lightweight and fast.
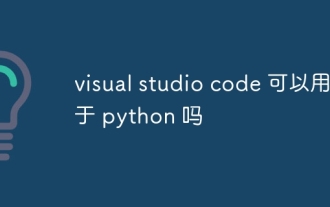
VS Code can be used to write Python and provides many features that make it an ideal tool for developing Python applications. It allows users to: install Python extensions to get functions such as code completion, syntax highlighting, and debugging. Use the debugger to track code step by step, find and fix errors. Integrate Git for version control. Use code formatting tools to maintain code consistency. Use the Linting tool to spot potential problems ahead of time.
