


Give an example of a scenario where using a Python list would be more appropriate than using an array.
Python lists are better than arrays for managing diverse data types. 1) Lists can hold elements of different types, 2) they are dynamic, allowing easy additions and removals, 3) they offer intuitive operations like slicing, but 4) they are less memory-efficient and slower for large datasets.
When it comes to choosing between a Python list and an array, understanding the strengths and nuances of each can significantly impact the efficiency and readability of your code. In this exploration, we'll dive into a scenario where a Python list shines brighter than an array, and then we'll unpack the broader implications and best practices surrounding this choice.
Imagine you're working on a project where you need to manage a collection of diverse data types, perhaps for a social media platform. You're tasked with storing user profiles, which include a mix of data types such as strings for names, integers for age, lists for interests, and even dictionaries for more complex user data. In this case, a Python list is the perfect tool for the job.
Here's why: Python lists are incredibly versatile. They can hold elements of different types within the same list, which is not easily achievable with arrays, especially in languages like C or Java where arrays are strictly typed. Let's look at a simple example to illustrate this:
user_profile = ["John Doe", 30, ["reading", "coding"], {"location": "New York"}]
In this snippet, user_profile
is a list that contains a string, an integer, a list, and a dictionary. This flexibility allows you to manage complex data structures with ease, something that arrays are not designed to handle as smoothly.
Now, let's dive deeper into why Python lists are often preferred in scenarios like this, and explore some best practices and potential pitfalls.
Python lists are dynamic. You can add or remove elements at any time, which is incredibly useful when dealing with data that changes frequently. For instance, if a user updates their interests, you can easily modify the list without worrying about resizing an array.
# Adding a new interest user_profile[2].append("traveling") # Removing an old interest user_profile[2].remove("reading")
However, this flexibility comes with a cost. Lists are less memory-efficient than arrays because they need to store additional metadata to handle their dynamic nature. If you're dealing with large datasets and memory is a concern, you might want to consider using arrays or other more specialized data structures.
Another advantage of lists is their built-in methods and ease of use. Operations like slicing, concatenation, and searching are straightforward and often more intuitive than with arrays. Here's an example of slicing:
# Get the user's interests interests = user_profile[2] print(interests) # Output: ['coding', 'traveling']
But be cautious with large lists. Operations like searching can become slow because lists are not optimized for quick lookups. In such cases, consider using data structures like sets or dictionaries for better performance.
When it comes to performance optimization, it's crucial to understand the time complexity of operations on lists. For example, appending to a list is generally O(1), but if the list needs to be resized, it can become O(n). Here's a quick performance tip:
# Pre-allocating memory for better performance large_list = [None] * 10000 for i in range(10000): large_list[i] = i
This approach can be more efficient when you know the approximate size of your list in advance.
In terms of best practices, always consider the readability and maintainability of your code. Lists are great for readability because they're straightforward and easy to understand. But don't overuse them. If you find yourself nesting lists too deeply, it might be time to reconsider your data structure.
Finally, let's touch on some common pitfalls and how to avoid them. One common mistake is modifying a list while iterating over it, which can lead to unexpected behavior. Here's how to avoid it:
# Incorrect way to remove items while iterating for item in user_profile[2]: if item == "coding": user_profile[2].remove(item) # This can skip items # Correct way using a list comprehension user_profile[2] = [item for item in user_profile[2] if item != "coding"]
In conclusion, while arrays have their place, especially in performance-critical applications, Python lists offer unparalleled flexibility and ease of use for managing diverse and dynamic data structures. By understanding the trade-offs and applying best practices, you can leverage lists to write more efficient, readable, and maintainable code.
The above is the detailed content of Give an example of a scenario where using a Python list would be more appropriate than using an array.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










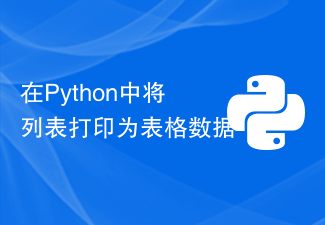
Data manipulation and analysis are key aspects of programming, especially when working with large data sets. A challenge programmers often face is how to present data in a clear and organized format that facilitates understanding and analysis. Being a versatile language, Python provides various techniques and libraries to print lists as tabular data, thus enabling visually appealing representation of information. Printing a list as tabular data involves arranging the data in rows and columns, similar to a tabular structure. This format makes it easier to compare and understand the relationships between different data points. Whether you are working on a data analysis project, generating reports, or presenting information to stakeholders, being able to print a list as a table in Python is a valuable skill. In this article, we will explore Pytho
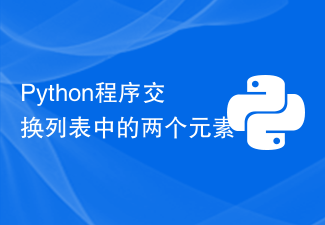
In Python programming, a list is a common and commonly used data structure. They allow us to store and manipulate collections of elements efficiently. Sometimes, we may need to swap the positions of two elements in a list, either to reorganize the list or to perform a specific operation. This blog post explores a Python program that swaps two elements in a list. We will discuss the problem, outline an approach to solving it, and provide a step-by-step algorithm. By understanding and implementing this program, you will be able to manipulate lists and change the arrangement of elements according to your requirements. Understanding the Problem Before we dive into solving the problem, let us clearly define what it means to swap two elements in a list. Swapping two elements in a list means swapping their positions. In other words, I
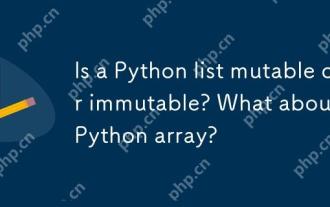
Pythonlistsandarraysarebothmutable.1)Listsareflexibleandsupportheterogeneousdatabutarelessmemory-efficient.2)Arraysaremorememory-efficientforhomogeneousdatabutlessversatile,requiringcorrecttypecodeusagetoavoiderrors.
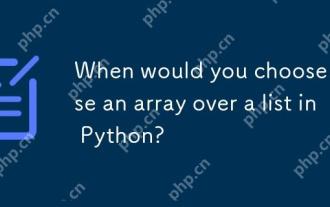
Useanarray.arrayoveralistinPythonwhendealingwithhomogeneousdata,performance-criticalcode,orinterfacingwithCcode.1)HomogeneousData:Arrayssavememorywithtypedelements.2)Performance-CriticalCode:Arraysofferbetterperformancefornumericaloperations.3)Interf
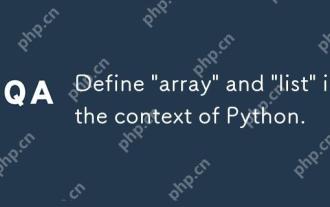
InPython,a"list"isaversatile,mutablesequencethatcanholdmixeddatatypes,whilean"array"isamorememory-efficient,homogeneoussequencerequiringelementsofthesametype.1)Listsareidealfordiversedatastorageandmanipulationduetotheirflexibility
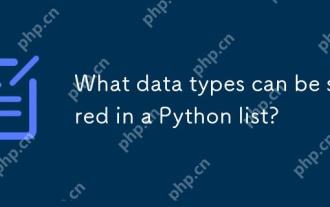
Pythonlistscanstoreanydatatype,includingintegers,strings,floats,booleans,otherlists,anddictionaries.Thisversatilityallowsformixed-typelists,whichcanbemanagedeffectivelyusingtypechecks,typehints,andspecializedlibrarieslikenumpyforperformance.Documenti
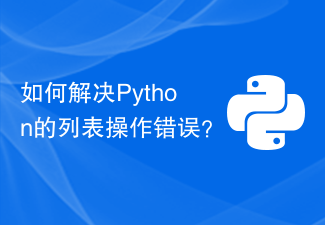
As a high-level programming language, Python provides many convenient data structures and operation methods. Among them, list is a very commonly used data structure in Python. It can store data of the same type or different types, and can perform various operations. However, when using Python lists, errors sometimes occur. This article will introduce how to solve Python list operation errors. IndexError (IndexError) In Python, the index of a list starts counting from 0,
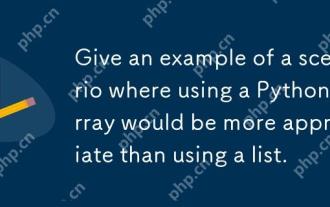
Using Python arrays is more suitable for processing large amounts of numerical data than lists. 1) Arrays save more memory, 2) Arrays are faster to operate by numerical values, 3) Arrays force type consistency, 4) Arrays are compatible with C arrays, but are not as flexible and convenient as lists.
