


Give an example of a scenario where using a Python array would be more appropriate than using a list.
Using Python arrays is more suitable for processing large amounts of numerical data than lists. 1) Arrays save more memory, 2) Arrays are faster to operate by numerical values, 3) Arrays force type consistency, 4) Arrays are compatible with C arrays, but are not as flexible and convenient as lists.
When it comes to choosing between a Python list and an array, understanding the nuances can significantly impact the performance and efficiency of your code. Let's dive into a scenario where using a Python array from the array
module would be more appropriate than using a list.
Imagine you're working on a project that involves processing large amounts of numerical data, such as a financial application that needs to handle stock prices or a scientific computing task dealing with sensor data. In such cases, using a Python array can offer substantial benefits over a list.
Here's a detailed exploration of why and how to use arrays effectively in this context:
In the world of Python, lists are incredibly versatile and easy to use. They can store elements of different types, grow or shrink dynamically, and are generally the go-to choice for many programming tasks. But what if you're dealing with a specific kind of data, like numbers, and performance matters a lot?
Let's say you're developing a financial application that processes millions of stock prices. Each stock price is a floating-point number, and you need to perform calculations on these numbers quickly. Here's where the array
module comes into play.
The array
module provides an array
object that is more memory-efficient and faster for numerical operations compared to a list. Unlike lists, which can contain elements of any type, arrays are typed, meaning they can only store elements of a single type. This restriction allows for more efficient memory usage and faster access times.
Here's a simple example to illustrate the difference:
import array import sys # Using a list to store numbers numbers_list = [1.0, 2.0, 3.0, 4.0, 5.0] print(f"Size of list: {sys.getsizeof(numbers_list)} bytes") # Using an array to store numbers numbers_array = array.array('d', [1.0, 2.0, 3.0, 4.0, 5.0]) print(f"Size of array: {sys.getsizeof(numbers_array)} bytes")
When you run this code, you'll notice that the array takes up less memory than the list. This difference becomes even more significant as the size of the data increases.
Now, let's consider a more practical scenario in our financial application:
import array import time # Simulating a large dataset of stock prices stock_prices_list = [float(i) for i in range(1000000)] stock_prices_array = array.array('d', [float(i) for i in range(1000000)]) # Measuring time to sum up all prices using a list start_time = time.time() total_list = sum(stock_prices_list) list_time = time.time() - start_time # Measuring time to sum up all prices using an array start_time = time.time() total_array = sum(stock_prices_array) array_time = time.time() - start_time print(f"Sum using list: {total_list}, Time: {list_time:.6f} seconds") print(f"Sum using array: {total_array}, Time: {array_time:.6f} seconds")
In this example, you'll likely see that the array performs the summation faster than the list, especially as the size of the dataset grows. This is because arrays are more optimized for numerical operations.
But it's not just about performance. Here are some additional considerations:
- Memory Efficiency : Arrays use less memory than lists for storing numerical data, which is cruel when dealing with large datasets.
- Type Safety : Arrays enforce type consistency, which can prevent bugs that might occur if you accidentally mix data types in a list.
- Interoperability : Arrays can be easily converted to and from C arrays, making them useful when interfacing with C libraries or when you need to optimize certain parts of your code.
However, there are some potential pitfalls to watch out for:
- Limited Flexibility : Since arrays are typed, you can't mix different types of data within the same array. This might limit their use in more general-purpose scenarios.
- Less Convenient : Arrays don't support some of the convenient methods that lists do, like
append
orinsert
. You'll need to useextend
to add elements, which can be less intentional.
In practice, I've found that the choice between lists and arrays often come down to the specific needs of your project. For general-purpose programming, lists are usually the better choice due to their flexibility. But when you're dealing with large datasets of numerical data and performance is critical, arrays can be a game-changer.
To wrap up, if you're working on a project that involves processing millions of numbers quickly and efficiently, consider using a Python array from the array
module. It might just be the edge you need to optimize your code and make your application run faster and more smoothly.
The above is the detailed content of Give an example of a scenario where using a Python array would be more appropriate than using a list.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










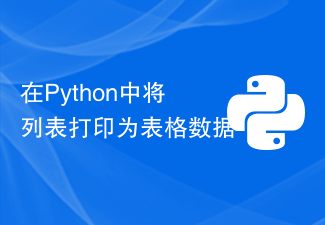
Data manipulation and analysis are key aspects of programming, especially when working with large data sets. A challenge programmers often face is how to present data in a clear and organized format that facilitates understanding and analysis. Being a versatile language, Python provides various techniques and libraries to print lists as tabular data, thus enabling visually appealing representation of information. Printing a list as tabular data involves arranging the data in rows and columns, similar to a tabular structure. This format makes it easier to compare and understand the relationships between different data points. Whether you are working on a data analysis project, generating reports, or presenting information to stakeholders, being able to print a list as a table in Python is a valuable skill. In this article, we will explore Pytho
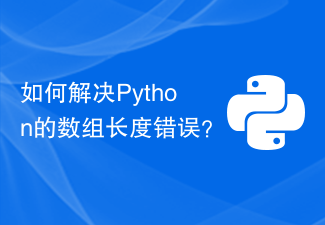
Python is a high-level programming language widely used in fields such as data analysis and machine learning. Among them, array is one of the commonly used data structures in Python, but during the development process, array length errors are often encountered. This article will detail how to solve Python's array length error. Length of Array First, we need to know the length of the array. In Python, the length of an array can vary, that is, we can modify the length of the array by adding or removing elements from the array. because
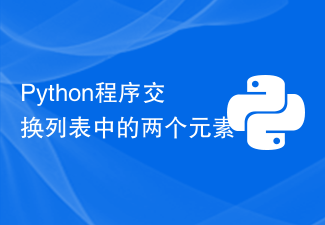
In Python programming, a list is a common and commonly used data structure. They allow us to store and manipulate collections of elements efficiently. Sometimes, we may need to swap the positions of two elements in a list, either to reorganize the list or to perform a specific operation. This blog post explores a Python program that swaps two elements in a list. We will discuss the problem, outline an approach to solving it, and provide a step-by-step algorithm. By understanding and implementing this program, you will be able to manipulate lists and change the arrangement of elements according to your requirements. Understanding the Problem Before we dive into solving the problem, let us clearly define what it means to swap two elements in a list. Swapping two elements in a list means swapping their positions. In other words, I
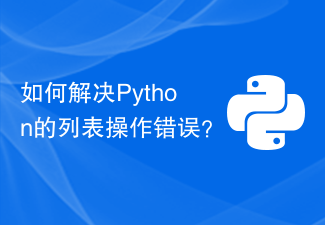
As a high-level programming language, Python provides many convenient data structures and operation methods. Among them, list is a very commonly used data structure in Python. It can store data of the same type or different types, and can perform various operations. However, when using Python lists, errors sometimes occur. This article will introduce how to solve Python list operation errors. IndexError (IndexError) In Python, the index of a list starts counting from 0,
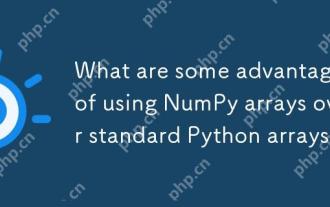
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making
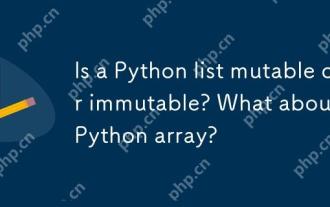
Pythonlistsandarraysarebothmutable.1)Listsareflexibleandsupportheterogeneousdatabutarelessmemory-efficient.2)Arraysaremorememory-efficientforhomogeneousdatabutlessversatile,requiringcorrecttypecodeusagetoavoiderrors.
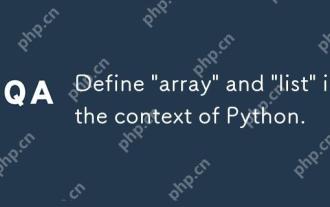
InPython,a"list"isaversatile,mutablesequencethatcanholdmixeddatatypes,whilean"array"isamorememory-efficient,homogeneoussequencerequiringelementsofthesametype.1)Listsareidealfordiversedatastorageandmanipulationduetotheirflexibility
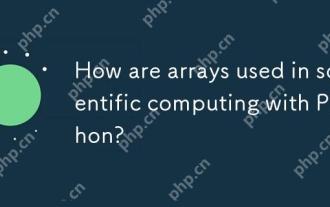
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
