How do you access elements in a Python array?
To access elements in a Python array, use indexing: my_array[2] accesses the third element, returning 3. Python uses zero-based indexing. 1) Use positive and negative indexing: my_list[0] for the first element, my_list[-1] for the last. 2) Use slicing for a range: my_list[1:5] extracts elements from index 1 to 4, my_list[::2] every second element, and my_list[::-1] reverses the list.
To access elements in a Python array, you use indexing. For example, if you have an array my_array = [1, 2, 3, 4, 5]
, you can access the third element with my_array[2]
, which returns 3
. Python uses zero-based indexing, so the first element is at index 0.
Now, let's dive deeper into the world of accessing elements in Python arrays, or more accurately, lists. I've been working with Python for years, and I've seen how mastering these basics can transform your coding efficiency and problem-solving skills.
When you're working with Python lists, understanding how to access elements is crucial. It's not just about getting the right value; it's about doing it efficiently and elegantly. Let's explore this in detail.
Accessing elements in Python lists is straightforward, but there's a lot more to it than meets the eye. For instance, you can use positive and negative indexing. Positive indexing starts from 0, while negative indexing starts from -1, which is the last element. Here's a quick example:
my_list = [10, 20, 30, 40, 50] print(my_list[0]) # Output: 10 print(my_list[-1]) # Output: 50
This flexibility is one of Python's strengths. It allows you to access elements from both ends of the list, which can be incredibly useful in certain scenarios, like when you need to process data from the end of a list.
But what if you want to access a range of elements? That's where slicing comes in. Slicing allows you to extract a subset of the list. The syntax is list[start:stop:step]
, where start
is the index of the first element you want to include, stop
is the index of the first element you want to exclude, and step
is the increment between elements. Here's how you can use it:
my_list = [10, 20, 30, 40, 50, 60, 70, 80, 90, 100] print(my_list[1:5]) # Output: [20, 30, 40, 50] print(my_list[::2]) # Output: [10, 30, 50, 70, 90] print(my_list[::-1]) # Output: [100, 90, 80, 70, 60, 50, 40, 30, 20, 10]
Slicing is powerful, but it's also easy to misuse. One common pitfall is forgetting that the stop
index is exclusive. This can lead to off-by-one errors, which can be frustrating to debug. Always double-check your indices when slicing.
Another aspect to consider is performance. Accessing elements by index is an O(1) operation, which means it's very fast. However, slicing can be more complex. If you're slicing a large list, it might be worth considering whether you really need all those elements at once, or if you can process them in smaller chunks.
In my experience, one of the best practices when working with lists is to keep them as simple as possible. If you find yourself constantly accessing elements in complex ways, it might be a sign that your data structure isn't the best fit for your problem. Sometimes, using a different data structure, like a dictionary or a set, can make your code more efficient and easier to understand.
To wrap up, accessing elements in Python lists is a fundamental skill, but it's also an area where you can showcase your mastery of the language. By understanding indexing, slicing, and the performance implications, you can write more efficient and elegant code. Remember, the key is to keep things simple and always consider whether your approach is the most efficient way to solve your problem.
The above is the detailed content of How do you access elements in a Python array?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










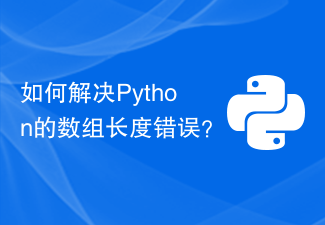
Python is a high-level programming language widely used in fields such as data analysis and machine learning. Among them, array is one of the commonly used data structures in Python, but during the development process, array length errors are often encountered. This article will detail how to solve Python's array length error. Length of Array First, we need to know the length of the array. In Python, the length of an array can vary, that is, we can modify the length of the array by adding or removing elements from the array. because
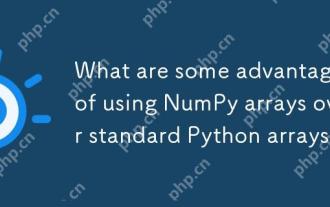
NumPyarrayshaveseveraladvantagesoverstandardPythonarrays:1)TheyaremuchfasterduetoC-basedimplementation,2)Theyaremorememory-efficient,especiallywithlargedatasets,and3)Theyofferoptimized,vectorizedfunctionsformathematicalandstatisticaloperations,making
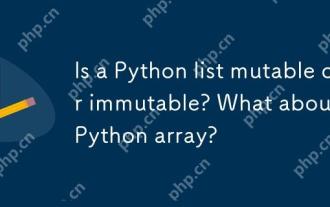
Pythonlistsandarraysarebothmutable.1)Listsareflexibleandsupportheterogeneousdatabutarelessmemory-efficient.2)Arraysaremorememory-efficientforhomogeneousdatabutlessversatile,requiringcorrecttypecodeusagetoavoiderrors.
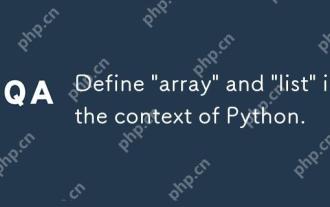
InPython,a"list"isaversatile,mutablesequencethatcanholdmixeddatatypes,whilean"array"isamorememory-efficient,homogeneoussequencerequiringelementsofthesametype.1)Listsareidealfordiversedatastorageandmanipulationduetotheirflexibility
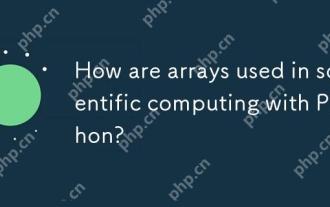
ArraysinPython,especiallyviaNumPy,arecrucialinscientificcomputingfortheirefficiencyandversatility.1)Theyareusedfornumericaloperations,dataanalysis,andmachinelearning.2)NumPy'simplementationinCensuresfasteroperationsthanPythonlists.3)Arraysenablequick
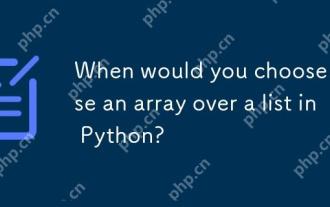
Useanarray.arrayoveralistinPythonwhendealingwithhomogeneousdata,performance-criticalcode,orinterfacingwithCcode.1)HomogeneousData:Arrayssavememorywithtypedelements.2)Performance-CriticalCode:Arraysofferbetterperformancefornumericaloperations.3)Interf

WhenyouattempttostoreavalueofthewrongdatatypeinaPythonarray,you'llencounteraTypeError.Thisisduetothearraymodule'sstricttypeenforcement,whichrequiresallelementstobeofthesametypeasspecifiedbythetypecode.Forperformancereasons,arraysaremoreefficientthanl
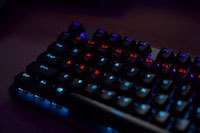
JavaIterator interface The Iterator interface is the standard interface in the Java collection framework for traversing collections. It defines a series of methods that allow client code to access elements in a collection in a sequential manner. The declaration of the Iterator interface is as follows: publicinterfaceIterator{booleanhasNext();Enext();voidremove();}hasNext() method is used to check whether there is a next element in the collection. The next() method is used to get the next element in the collection. The remove() method is used to remove the current element from the collection. Iterator interface is a generic interface
