Declarative Programming with React: Simplifying UI Logic
在React中,声明式编程通过描述UI的期望状态来简化UI逻辑。1) 通过定义UI状态,React会自动处理DOM更新。2) 这种方法使代码更清晰、易维护。3) 但需要注意状态管理复杂性和优化重渲染。
Declarative Programming with React: Simplifying UI Logic
When diving into the world of React, one quickly realizes the power of declarative programming. But what does it really mean to program declaratively, and how does it simplify UI logic in React? At its core, declarative programming is about describing what you want the program to do, rather than how to do it. In React, this translates to defining the desired state of your UI, letting React handle the "how" part.
I remember when I first started using React, the shift from imperative to declarative programming was mind-blowing. Instead of writing long sequences of DOM manipulation, I could simply describe what the UI should look like based on my application's state. This not only made my code cleaner but also more maintainable and easier to reason about.
Let's dive into how React leverages declarative programming to simplify UI logic, share some real-world examples, and discuss the pros and cons of this approach.
In React, you express your UI as a function of your application's state. For instance, if you want to display a list of items, you simply define a component that renders this list based on an array of items in your state. React then takes care of updating the DOM efficiently whenever the state changes. This abstraction from the "how" to the "what" is what makes React so powerful.
Here's a simple example to illustrate:
import React, { useState } from 'react'; function TodoList() { const [todos, setTodos] = useState([ { id: 1, text: 'Learn React' }, { id: 2, text: 'Build a project' }, ]); return ( <ul> {todos.map(todo => ( <li key={todo.id}>{todo.text}</li> ))} </ul> ); }
In this example, we're not telling the browser how to create a list or how to update it. We're simply saying, "Here's my state, and here's how I want it to look." React figures out the rest, which is incredibly liberating.
But it's not just about simplicity. Declarative programming in React also helps in managing complex UI states. Consider a more advanced scenario where you're building a dashboard with multiple interactive components. Each component can have its own state, and they might need to interact with each other. In an imperative approach, managing these interactions would be a nightmare. With React, you can define the state and the UI for each component declaratively, and React will handle the updates and interactions seamlessly.
Here's a more complex example to show how this works:
import React, { useState } from 'react'; function Dashboard() { const [selectedItem, setSelectedItem] = useState(null); const [items, setItems] = useState([ { id: 1, name: 'Item 1' }, { id: 2, name: 'Item 2' }, ]); const handleItemClick = (item) => { setSelectedItem(item); }; return ( <div> <ul> {items.map(item => ( <li key={item.id} onClick={() => handleItemClick(item)}> {item.name} </li> ))} </ul> {selectedItem && <ItemDetails item={selectedItem} />} </div> ); } function ItemDetails({ item }) { return ( <div> <h2>{item.name}</h2> <p>Details about {item.name}</p> </div> ); }
In this dashboard example, we're managing the state of selected items and the list of items declaratively. When an item is clicked, the state updates, and React re-renders the UI accordingly. The ItemDetails
component is only rendered when an item is selected, showcasing how React efficiently manages the UI based on the state.
However, while declarative programming in React is incredibly powerful, it's not without its challenges. One common pitfall is overcomplicating the state management. If you have too many nested states or if you're constantly lifting state up to parent components, it can lead to a complex and hard-to-maintain codebase. To mitigate this, consider using state management libraries like Redux or Context API for more complex applications.
Another challenge is understanding how React optimizes re-renders. While React is efficient, unnecessary re-renders can still happen if not managed properly. Using React.memo
or shouldComponentUpdate
can help optimize performance, but it requires a good understanding of React's reconciliation process.
In terms of best practices, always keep your components as pure as possible. This means that given the same props, a component should always render the same output. This not only makes your components easier to test but also helps React optimize performance.
To wrap up, declarative programming in React has revolutionized how we build user interfaces. It simplifies UI logic by abstracting away the "how" and focusing on the "what." While it comes with its own set of challenges and learning curves, the benefits in terms of code clarity, maintainability, and performance are undeniable. As you continue your journey with React, embrace the declarative mindset, and you'll find yourself building more efficient and elegant UIs.
The above is the detailed content of Declarative Programming with React: Simplifying UI Logic. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










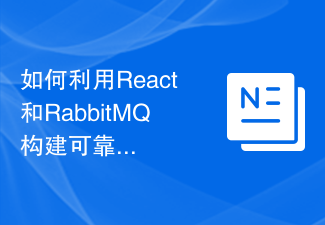
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
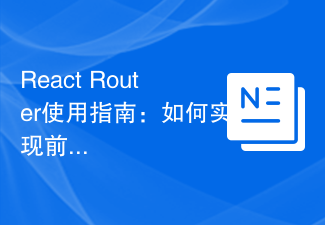
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
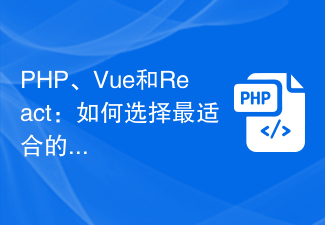
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
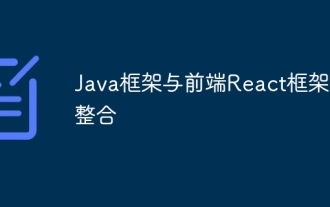
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
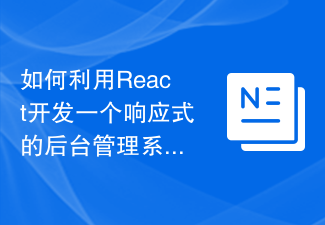
How to use React to develop a responsive backend management system. With the rapid development of the Internet, more and more companies and organizations need an efficient, flexible, and easy-to-manage backend management system to handle daily operations. As one of the most popular JavaScript libraries currently, React provides a concise, efficient and maintainable way to build user interfaces. This article will introduce how to use React to develop a responsive backend management system and give specific code examples. Create a React project first

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
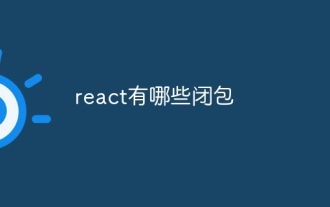
React has closures such as event handling functions, useEffect and useCallback, higher-order components, etc. Detailed introduction: 1. Event handling function closure: In React, when we define an event handling function in a component, the function will form a closure and can access the status and properties within the component scope. In this way, the state and properties of the component can be used in the event processing function to implement interactive logic; 2. Closures in useEffect and useCallback, etc.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
