Understanding Keys in React: Optimizing List Rendering
In React, keys are essential for optimizing list rendering performance by helping React track changes in list items. 1) Keys enable efficient DOM updates by identifying added, changed, or removed items. 2) Using unique identifiers like database IDs as keys, rather than indices, prevents issues with dynamic lists. 3) Proper key usage maintains component state integrity, avoiding unnecessary re-renders and state resets.
When it comes to React, understanding the role of keys in list rendering is crucial for optimizing your application's performance. Keys help React identify which items have changed, been added, or been removed, which in turn affects how efficiently the DOM is updated.
Diving into the world of React, I've come to appreciate how keys not only streamline the rendering process but also play a significant role in maintaining the integrity of component state. Let's explore this fascinating aspect of React development.
In my journey with React, I've often marveled at how a simple concept like keys can drastically improve the performance of list rendering. When you're working with lists in React, keys are your secret weapon for ensuring that the framework understands the structure of your data. Imagine you're building a todo list app; without keys, React would struggle to keep track of which todo item you're updating or deleting, leading to unpredictable behavior and performance hits.
Take this example: if you're rendering a list of items without keys, React has to resort to using the index of the item in the array as a fallback. This can lead to issues when the list is reordered or filtered, as React might confuse one item for another. Here's how you might implement a list without keys:
const TodoList = ({ todos }) => ( <ul> {todos.map((todo, index) => ( <li key={index}>{todo.text}</li> ))} </ul> );
While this works, it's not optimal. Using the index as a key can cause problems when the list is dynamic. If you insert an item at the beginning of the list, all subsequent items will shift down, but React will think they're new items because their indices have changed. This leads to unnecessary re-renders and can mess with component state.
Instead, you should use a unique identifier for each item as the key. If your todo items have an id
field, use that:
const TodoList = ({ todos }) => ( <ul> {todos.map(todo => ( <li key={todo.id}>{todo.text}</li> ))} </ul> );
This approach ensures that React can correctly identify each item, even when the list order changes. It's like giving each todo item a unique fingerprint that React can use to track its lifecycle.
One pitfall I've encountered is using keys that are not stable across re-renders. For instance, if you're generating keys based on some mutable data, you might end up with the same issues as using indices. Always opt for keys that are consistent and unique, like database IDs or UUIDs.
Another aspect to consider is the impact of keys on component state. If you're using keys incorrectly, you might lose component state when the list is updated. Imagine a todo item with an editable field; if the key changes, React might think it's a new item and reset the field to its initial state. This can be frustrating for users and is a common gotcha in React development.
In terms of performance optimization, using keys correctly can significantly reduce the number of DOM operations. React can reconcile the virtual DOM more efficiently when it knows exactly which items have changed. This is particularly important in large lists where even small optimizations can lead to noticeable improvements in user experience.
To further optimize list rendering, consider techniques like virtualization, where you only render the items currently visible in the viewport. Libraries like react-window
can help with this, but they still rely on proper key usage to function correctly.
In my experience, the best practice is to always use keys, even if you're rendering a static list. It's a good habit that ensures your code is future-proof and easier to maintain. Plus, it's a clear signal to other developers working on your codebase that you understand React's reconciliation process.
So, when you're next working with lists in React, remember the power of keys. They're not just a syntax requirement; they're a fundamental part of building efficient, stateful, and user-friendly applications. By mastering the use of keys, you'll unlock a deeper understanding of React's inner workings and be able to craft applications that perform beautifully, no matter how complex your data gets.
The above is the detailed content of Understanding Keys in React: Optimizing List Rendering. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










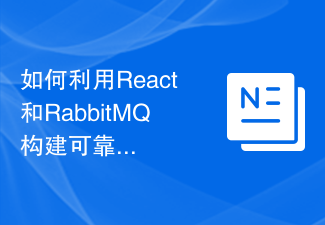
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
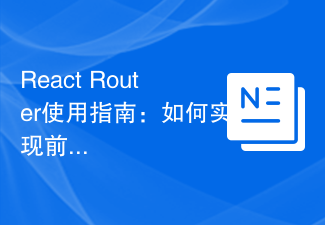
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
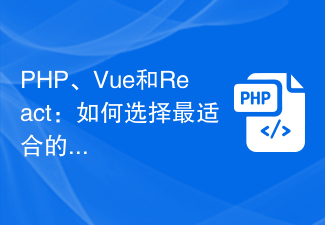
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
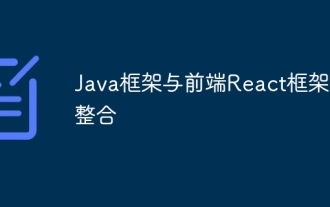
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
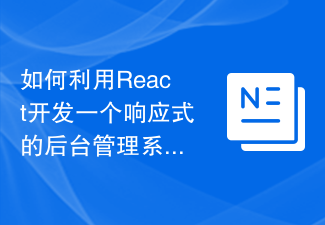
How to use React to develop a responsive backend management system. With the rapid development of the Internet, more and more companies and organizations need an efficient, flexible, and easy-to-manage backend management system to handle daily operations. As one of the most popular JavaScript libraries currently, React provides a concise, efficient and maintainable way to build user interfaces. This article will introduce how to use React to develop a responsive backend management system and give specific code examples. Create a React project first

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
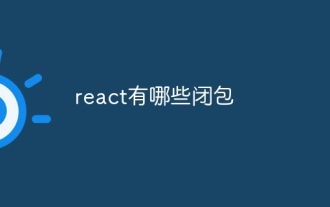
React has closures such as event handling functions, useEffect and useCallback, higher-order components, etc. Detailed introduction: 1. Event handling function closure: In React, when we define an event handling function in a component, the function will form a closure and can access the status and properties within the component scope. In this way, the state and properties of the component can be used in the event processing function to implement interactive logic; 2. Closures in useEffect and useCallback, etc.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
