


The Boilerplate Code Required for React Projects: Reducing Setup Overhead
To reduce setup overhead in React projects, use tools like Create React App (CRA), Next.js, Gatsby, or starter kits, and maintain a modular structure. 1) CRA simplifies setup with a single command. 2) Next.js and Gatsby offer more features but a learning curve. 3) Starter kits provide comprehensive setups. 4) A modular structure enhances maintainability and scalability.
In the world of React development, one of the most frequent challenges developers face is managing the boilerplate code that comes with setting up a new project. This overhead can be a significant barrier, especially for newcomers or when working on quick prototypes. So, how can we reduce the setup overhead in React projects?
Let's dive into the world of React and explore various strategies and tools that can help us minimize the boilerplate code, making our development process smoother and more efficient.
When I first started with React, the sheer amount of setup required for even a simple project was overwhelming. I remember spending hours configuring webpack, setting up Babel, and ensuring all dependencies were correctly managed. It's a common pain point, but over time, I've discovered several approaches that drastically cut down on this setup time.
One of the most effective ways to reduce setup overhead is by using Create React App (CRA). CRA is a tool developed by the React team that sets up a new React project with a single command. It handles all the configuration for you, including webpack, Babel, and other essential tools. Here's how you can get started with CRA:
npx create-react-app my-app cd my-app npm start
This simplicity is a game-changer, especially for beginners. However, CRA comes with its own set of limitations. For instance, it's not as flexible as a custom setup, and ejecting from CRA to gain more control can be a complex process. If you need more customization, you might want to look into alternatives like Next.js or Gatsby, which offer more out-of-the-box features while still providing a streamlined setup process.
Next.js, for example, is particularly useful for server-side rendering and static site generation. It comes with built-in routing and a lot of optimizations out of the box. Here's a quick example of how to start a Next.js project:
npx create-next-app my-next-app cd my-next-app npm run dev
Gatsby, on the other hand, is excellent for content-driven sites and offers a rich plugin ecosystem. Starting a Gatsby project is just as straightforward:
npx gatsby new my-gatsby-app cd my-gatsby-app npm run develop
Both Next.js and Gatsby provide more features than CRA but might introduce a learning curve due to their additional capabilities. It's essential to weigh the trade-offs and choose the tool that best fits your project's needs.
Another approach to reducing boilerplate is to use a starter kit or a boilerplate project. There are numerous open-source projects on GitHub that you can clone and use as a starting point. For instance, the react-boilerplate
project offers a more comprehensive setup with Redux, React Router, and other commonly used libraries. Here's how you can use it:
git clone https://github.com/react-boilerplate/react-boilerplate.git my-react-app cd my-react-app npm install npm start
While starter kits can save time, they often come with more code than you might need, which can be overwhelming. It's crucial to understand the components and configurations included in these kits to avoid unnecessary complexity.
In my experience, one of the most overlooked aspects of reducing setup overhead is maintaining a clean and modular project structure. By organizing your code into clear, reusable components and using tools like ESLint and Prettier, you can ensure that your project remains maintainable and easy to navigate. Here's a simple example of a modular component structure:
// src/components/Button.js import React from 'react'; const Button = ({ onClick, children }) => ( <button onClick={onClick}> {children} </button> ); export default Button; // src/components/Header.js import React from 'react'; import Button from './Button'; const Header = ({ onLogin }) => ( <header> <h1 id="My-App">My App</h1> <Button onClick={onLogin}>Login</Button> </header> ); export default Header;
This approach not only reduces the initial setup time but also makes your project more scalable and easier to maintain in the long run.
When it comes to performance optimization, tools like react-fast-refresh
can significantly improve your development experience by providing faster feedback loops. Additionally, using modern React features like hooks can help reduce the amount of boilerplate code you need to write. For example, instead of using class components with lifecycle methods, you can use functional components with hooks:
// Before: Class Component import React, { Component } from 'react'; class Counter extends Component { constructor(props) { super(props); this.state = { count: 0 }; } increment = () => { this.setState({ count: this.state.count 1 }); }; render() { return ( <div> <p>Count: {this.state.count}</p> <button onClick={this.increment}>Increment</button> </div> ); } } // After: Functional Component with Hooks import React, { useState } from 'react'; const Counter = () => { const [count, setCount] = useState(0); const increment = () => { setCount(count 1); }; return ( <div> <p>Count: {count}</p> <button onClick={increment}>Increment</button> </div> ); };
In conclusion, reducing setup overhead in React projects is all about finding the right balance between simplicity and customization. Whether you choose to use tools like Create React App, Next.js, or Gatsby, or opt for a starter kit, the key is to understand your project's requirements and choose the approach that best suits your needs. By maintaining a clean, modular structure and leveraging modern React features, you can significantly streamline your development process and focus more on building great applications.
The above is the detailed content of The Boilerplate Code Required for React Projects: Reducing Setup Overhead. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










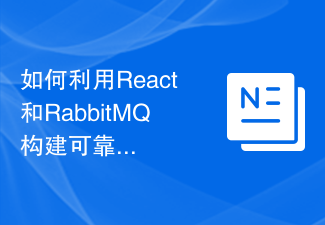
How to build a reliable messaging application with React and RabbitMQ Introduction: Modern applications need to support reliable messaging to achieve features such as real-time updates and data synchronization. React is a popular JavaScript library for building user interfaces, while RabbitMQ is a reliable messaging middleware. This article will introduce how to combine React and RabbitMQ to build a reliable messaging application, and provide specific code examples. RabbitMQ overview:
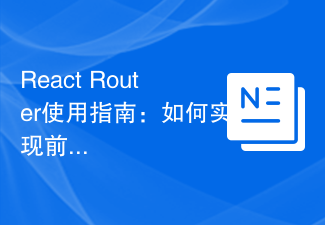
ReactRouter User Guide: How to Implement Front-End Routing Control With the popularity of single-page applications, front-end routing has become an important part that cannot be ignored. As the most popular routing library in the React ecosystem, ReactRouter provides rich functions and easy-to-use APIs, making the implementation of front-end routing very simple and flexible. This article will introduce how to use ReactRouter and provide some specific code examples. To install ReactRouter first, we need
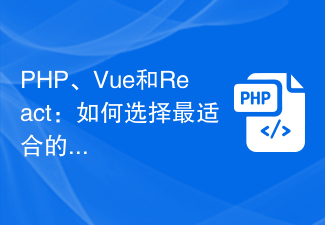
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
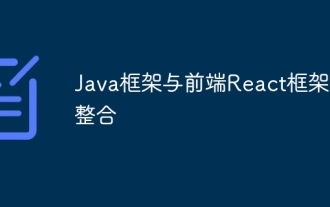
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
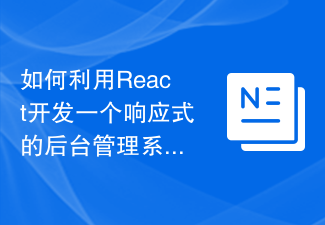
How to use React to develop a responsive backend management system. With the rapid development of the Internet, more and more companies and organizations need an efficient, flexible, and easy-to-manage backend management system to handle daily operations. As one of the most popular JavaScript libraries currently, React provides a concise, efficient and maintainable way to build user interfaces. This article will introduce how to use React to develop a responsive backend management system and give specific code examples. Create a React project first

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.
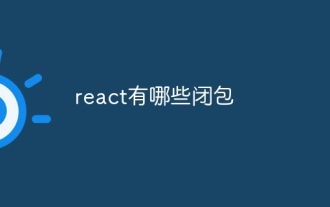
React has closures such as event handling functions, useEffect and useCallback, higher-order components, etc. Detailed introduction: 1. Event handling function closure: In React, when we define an event handling function in a component, the function will form a closure and can access the status and properties within the component scope. In this way, the state and properties of the component can be used in the event processing function to implement interactive logic; 2. Closures in useEffect and useCallback, etc.
