How can you optimize PHP session performance?
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
introduction
In modern web development, PHP session management is an indispensable part, but it often becomes a performance bottleneck. Today we will discuss how to optimize PHP session performance to make your applications faster and more efficient. With this article, you will learn how to improve session processing efficiency from multiple perspectives, avoid common performance pitfalls, and master some practical best practices.
Review of basic knowledge
PHP session management is started through the session_start()
function, which creates a unique session ID and stores session data on the server side. Session data is usually stored in a file system, but can also be configured to use a database or other storage mechanism. Understanding how a session works is the first step in optimizing performance.
Core concept or function analysis
Definition and function of PHP session
A PHP session is a mechanism for maintaining user data between different page requests. Its main function is to keep users logged in or save temporary data while browsing the website. The advantage of a session is that it provides a convenient way to persist data, but if used improperly, it can lead to performance problems.
How it works
When session_start()
is called, PHP will check whether there is a valid session ID. If not, it generates a new ID and creates a corresponding session file on the server. Each time a request is requested, PHP reads this file, updates the session data, and writes the data back to the file at the end of the request. This kind of read and write operation may become a bottleneck in high concurrency environments.
Example of usage
Basic usage
// Start the session session_start(); // Set the session variable $_SESSION['username'] = 'example_user'; // Read the session variable echo $_SESSION['username'];
This code shows how to start a session, set and read session variables. Simple and straightforward, but if every request operates like this, performance will be affected.
Advanced Usage
// Start the session only if (!isset($_SESSION)) { session_start(); } // Use the database to store session data ini_set('session.save_handler', 'user'); session_set_save_handler( 'open', 'close', 'read', 'write', 'destroy', 'gc' ); // Custom session processing function function open($save_path, $session_name) { // Open the database connection and return true; } function close() { // Close the database connection and return true; } function read($id) { // Read session data from the database return ''; } function write($id, $data) { // Write session data to the database return true; } function destroy($id) { // Delete session data from the database return true; } function gc($maxlifetime) { // Clean out expired session return true; }
This code shows how to start a session when needed and how to use a database to store session data. This approach can significantly improve performance, especially in high concurrency environments.
Common Errors and Debugging Tips
- Frequently starting sessions : calling
session_start()
for each request will increase unnecessary overhead. The solution is to start the session only if needed. - Too large session data : storing too much data in a session will increase read and write time. It is recommended to store only the necessary data and consider using other storage mechanisms.
- Session file locking : In high concurrency environments, session file locking may cause request blockage. Using a database to store session data can avoid this problem.
Performance optimization and best practices
The key to optimizing PHP session performance is to reduce unnecessary read and write operations and choose the appropriate storage mechanism. Here are some specific optimization strategies:
- Delayed session start : Starting a session only when you need to access the session data, can reduce unnecessary overhead.
// Delay session start if (isset($_POST['login'])) { session_start(); // Handle login logic}
- Using database storage sessions : Databases can provide better concurrency performance and scalability than file systems.
// Configure the use of database storage session ini_set('session.save_handler', 'user'); session_set_save_handler( 'open', 'close', 'read', 'write', 'destroy', 'gc' );
- Session data compression : If the session data is large, consider using serialization and compression to reduce storage space.
// Compress session data $_SESSION['data'] = gzcompress(serialize($data));
- Session life cycle management : Set the life cycle of the session reasonably to avoid long-term use of resources.
// Set the session life cycle to 30 minutes ini_set('session.gc_maxlifetime', 1800);
- Load balancing and session sharing : In a distributed environment, using session sharing mechanisms can improve the scalability of the system.
// Use Redis to store session data ini_set('session.save_handler', 'redis'); ini_set('session.save_path', 'tcp://127.0.0.1:6379');
In practical applications, the effects of these optimization strategies will vary depending on the specific environment. It is recommended to perform performance testing before implementation to evaluate the effectiveness of different methods. At the same time, maintaining the readability and maintenance of the code is also part of optimization to avoid excessive optimization leading to increased code complexity.
Through these methods, you can significantly improve the performance of your PHP sessions and ensure that your application can still run efficiently in high concurrency environments.
The above is the detailed content of How can you optimize PHP session performance?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
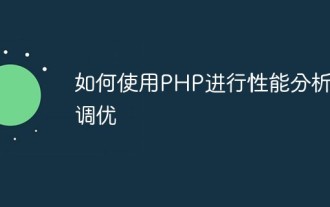
As a popular server-side language, PHP plays an important role in website development and operation. However, as the amount of PHP code continues to increase and the complexity of applications increases, performance bottlenecks become more and more likely to occur. In order to avoid this problem, we need to perform performance analysis and tuning. This article will briefly introduce how to use PHP for performance analysis and tuning to provide a more efficient running environment for your applications. 1. PHP performance analysis tool 1.XdebugXdebug is a widely used code analysis tool.
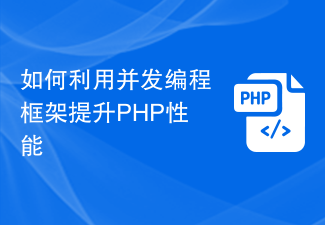
How to use concurrent programming framework to improve PHP performance As the complexity of web applications continues to increase, high concurrency processing has become a challenge faced by developers. The traditional PHP language has performance bottlenecks when handling concurrent requests, which forces developers to find more efficient solutions. Using concurrent programming frameworks, such as Swoole and ReactPHP, can significantly improve PHP's performance and concurrent processing capabilities. This article will introduce how to improve the performance of PHP applications by using Swoole and ReactPHP. we will
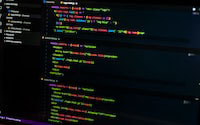
Introduction to PHPCI/CD CI/CD (Continuous Integration and Continuous Delivery) is a software development practice that helps development teams deliver high-quality software more frequently. The CI/CD process typically includes the following steps: Developers submit code to a version control system. The build system automatically builds code and runs unit tests. If the build and tests pass, the code is deployed to the test environment. Testers test code in a test environment. If the tests pass, the code is deployed to production. How does CI/CD improve the performance of PHP projects? CI/CD can improve the performance of PHP projects for the following reasons: Automated testing. CI/CD processes often include automated testing, which can help development teams find and fix bugs early. this
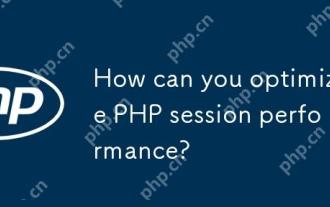
Methods to optimize PHP session performance include: 1. Delay session start, 2. Use database to store sessions, 3. Compress session data, 4. Manage session life cycle, and 5. Implement session sharing. These strategies can significantly improve the efficiency of applications in high concurrency environments.
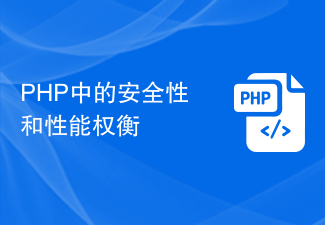
Summary of security and performance trade-offs in PHP: As a popular web programming language, PHP not only provides a flexible development environment and rich features, but also faces security and performance trade-offs. This article will explore security and performance issues in PHP and provide some code examples to illustrate how to strike a balance between the two. Introduction: In web application development, security and performance are two interrelated but independently important aspects. The server-side language PHP has good programming features and powerful functions. However, it is not suitable for
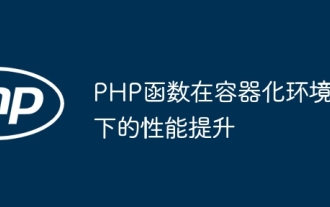
PHP function performance optimization strategies in containerized environments include: Upgrading the PHP version Optimizing PHP configuration (such as increasing memory limits, enabling OPcache, etc.) Using PHP extensions (such as APC, Xdebug, Swoole, etc.) Optimizing container configuration (such as setting memory and CPU limits) )
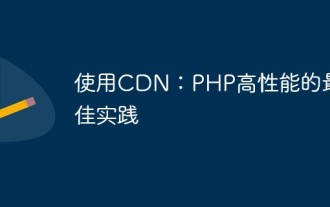
Nowadays, the Internet has become a daily necessity for more and more enterprises and individuals, and Web applications are receiving more and more attention. For web applications, performance has always been an important indicator, usually measured by access speed and response time. In PHP Web applications, using CDN is a common method to improve performance. Let's discuss the topic of "Using CDN: Best Practices for High Performance in PHP". First we need to understand what CDN is. CDN (ContentDelivery
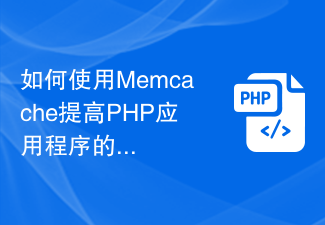
Memcache is an efficient caching solution that can greatly improve the performance of PHP applications. In this article, we'll cover how to use Memcache to optimize the performance of your PHP applications and provide practical PHP code examples. What is Memcache? Memcache is an open source distributed caching solution that stores data in memory to provide fast responses. Because the data is stored in memory, queries are very fast. Resolved with other databases
