What are triggers in MySQL?
A MySQL trigger is an automatically executed stored procedure associated with a table that is used to perform a series of operations when a specific data operation is performed. 1) Trigger definition and function: used for data verification, logging, etc. 2) Working principle: It is divided into BEFORE and AFTER, and supports row-level triggering. 3) Example of use: Can be used to record salary changes or update inventory. 4) Debugging skills: Use SHOW TRIGGERS and SHOW CREATE TRIGGER commands. 5) Performance optimization: Avoid complex operations, use indexes, and manage transactions.
introduction
In the world of MySQL, triggers are like "automation wizards" in databases, which are automatically executed when specific database operations occur. This article will take you into the mystery of MySQL triggers, revealing how they work and how to use them efficiently in real projects. After reading this article, you will master the definition of triggers, how to use them, and how to avoid common pitfalls to improve your database management skills.
Review of basic knowledge
Before explaining triggers, you might as well review some basic concepts in MySQL. A trigger is a special stored procedure associated with a table, which is automatically executed when specific data operations (such as INSERT, UPDATE, DELETE) occur. Understanding the concept of table structure, SQL statement execution process, and stored procedures is crucial to mastering triggers.
Core concept or function analysis
Definition and function of triggers
Triggers are a powerful tool in MySQL that allows you to automatically perform a series of operations when table data changes. They can be used in scenarios such as data verification, logging, and implementation of complex business logic. For example, you can set up a trigger that automatically logs the user's registration time to the log table every time a new user is inserted.
DELIMITER // CREATE TRIGGER after_insert_user AFTER INSERT ON users FOR EACH ROW BEGIN INSERT INTO user_logs (user_id, action, timestamp) VALUES (NEW.id, 'INSERT', NOW()); END // DELIMITER ;
This example shows a trigger that fires after inserting a new record in the users
table. It inserts the new user's ID, operation type, and current time into the user_logs
table.
How it works
When you perform a trigger-associated SQL operation, MySQL will automatically check whether there are related triggers. If so, MySQL executes them in the order defined by the triggers. Triggers can be divided into two categories: BEFORE and AFTER, which are executed before and after the operation. In addition, the trigger can be a FOR EACH ROW, which means that for multi-row operations, the trigger will be executed once for each row of data.
The execution order and transactionality of triggers need special attention. The trigger is executed in the same transaction, which means that if the operation in the trigger fails, the entire transaction will be rolled back. Therefore, when writing triggers, you must ensure the robustness and efficiency of their logic.
Example of usage
Basic usage
Let's look at a simple trigger example for recording salary change history when updating employee salary:
DELIMITER // CREATE TRIGGER before_update_salary BEFORE UPDATE ON employees FOR EACH ROW BEGIN IF NEW.salary != OLD.salary THEN INSERT INTO salary_history (employee_id, old_salary, new_salary, change_date) VALUES (OLD.id, OLD.salary, NEW.salary, NOW()); END IF; END // DELIMITER ;
This trigger will record the salary before and after the change in the salary of employees to the salary_history
table when the employee salary changes.
Advanced Usage
Triggers can also be used to implement complex business logic, for example, in an order system, automatically update inventory when the order status changes from "pending" to "completed":
DELIMITER // CREATE TRIGGER after_update_order AFTER UPDATE ON orders FOR EACH ROW BEGIN IF NEW.status = 'COMPLETED' AND OLD.status != 'COMPLETED' THEN UPDATE products SET stock = stock - NEW.quantity WHERE id = NEW.product_id; END IF; END // DELIMITER ;
This trigger will automatically reduce the inventory of the corresponding product when the order status becomes "Completed".
Common Errors and Debugging Tips
Common errors when using triggers include trigger logic errors, performance issues, and conflicts between triggers. When debugging triggers, you can use the SHOW TRIGGERS
command to view the trigger in the current database and view the definition of the trigger through the SHOW CREATE TRIGGER
command. In addition, MySQL's logging system can also help you track the execution of triggers.
Performance optimization and best practices
In practical applications, the performance optimization of triggers is crucial. The execution of triggers may affect the overall performance of the database and therefore requires careful design. Here are some optimization suggestions:
- Avoid complex operations : The operations in the trigger should be as simple as possible to avoid complex queries or large amounts of data operations.
- Using indexes : Establishing appropriate indexes on the tables involved in the trigger can improve the execution efficiency of the trigger.
- Transaction management : Ensure that operations in the trigger do not cause excessive transaction swelling and affect the concurrency performance of the database.
Additionally, the following best practices should be followed when writing triggers:
- Clear naming : The name of the trigger should clearly reflect its function for easy maintenance and understanding.
- Comments and Documents : Add detailed comments and documentation to triggers to facilitate team members to understand their logic.
- Testing and Verification : Perform adequate testing and verification before using triggers in a production environment to ensure their accuracy and stability.
Through these experiences and suggestions, you will be able to more effectively utilize MySQL triggers to improve the automation and business logic processing capabilities of your database.
The above is the detailed content of What are triggers in MySQL?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics










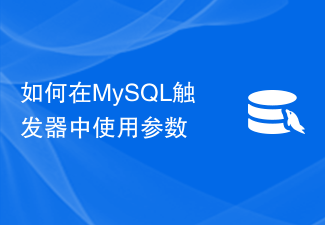
How to use parameters in MySQL triggers requires specific code examples. MySQL is a popular relational database management system that supports triggers to monitor changes in data in tables and perform corresponding operations. Triggers can be triggered when an INSERT, UPDATE or DELETE operation occurs. It is a powerful database function that can be used to implement data constraints, logging, data synchronization and other requirements. In MySQL, triggers can use parameters to pass data, and the parameters can be used to flexibly customize the trigger.
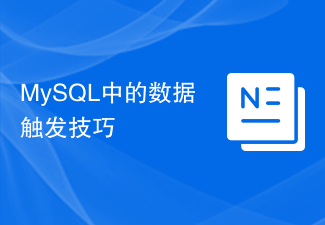
MySQL is a widely used relational database management system that supports many different operations and functions. One of them is the data triggering technique, which can monitor and process data changes by defining triggers in the database. This article will introduce the basic principles, usage and examples of data triggering techniques in MySQL. 1. Basic principles of data triggers Data triggers in MySQL are a special type of stored procedures that can be defined and executed in the database. It is closely associated with the table. When a specified event (such as insert, update

In recent years, with the continuous development of Internet technology, the development of various websites and applications has attracted more and more attention. In these applications, the database is an important component. In databases, triggers are a commonly used feature that automatically performs a series of operations when certain operations occur. PHP can be used to write database triggers. This article will introduce how to use PHP to write database triggers. 1. What is a database trigger? In a relational database, a trigger is a special stored procedure.
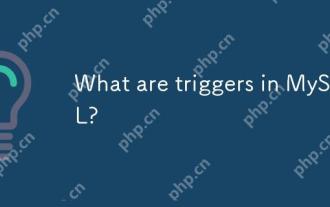
A MySQL trigger is an automatically executed stored procedure associated with a table that is used to perform a series of operations when a specific data operation is performed. 1) Trigger definition and function: used for data verification, logging, etc. 2) Working principle: It is divided into BEFORE and AFTER, and supports row-level triggering. 3) Example of use: Can be used to record salary changes or update inventory. 4) Debugging skills: Use SHOWTRIGGERS and SHOWCREATETRIGGER commands. 5) Performance optimization: Avoid complex operations, use indexes, and manage transactions.
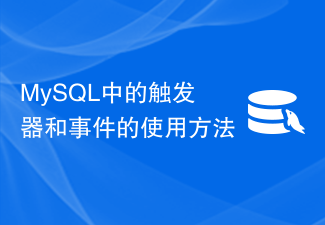
MySQL is a commonly used relational database management system. Its powerful functions and flexible usage make it the first choice of many developers and enterprises. In MySQL, triggers and events are two important concepts that can implement functions such as data monitoring, data integrity protection, and data synchronization. This article will introduce the use of triggers and events in MySQL. 1. Trigger overview Trigger is a special stored procedure in MySQL. It is associated with the table and automatically

Title: Using MySQL triggers to automate database operations. In database management, triggers are a powerful tool that can help us automate database operations. As a widely used open source database management system, MySQL also provides trigger functions. We can use MySQL triggers to automate database operations. This article will introduce the basic concepts and specific implementation methods of MySQL triggers, and provide some code examples to help readers better understand how to utilize MySQL triggers.
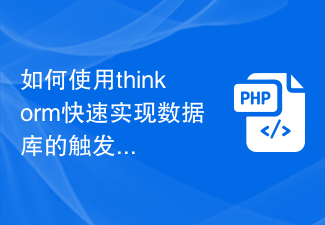
How to use ThinkORM to quickly implement database triggers and stored procedures Introduction: Triggers and stored procedures are very important tools when developing and maintaining a database. They can make our database operations more flexible and efficient. This article will introduce how to use ThinkORM to quickly implement database triggers and stored procedures, and explain in detail through code examples. 1. Implementation of triggers A trigger is an action associated with a table. When the data on the table changes, the trigger will automatically perform the corresponding operation. The following is passed
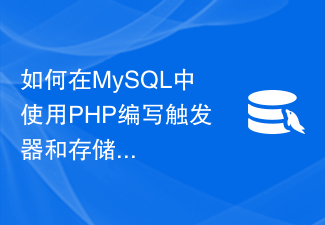
How to use PHP to write triggers and stored procedures in MySQL MySQL is a commonly used relational database management system, and PHP is a commonly used server-side scripting language. The two are often used in combination to complete complex data processing tasks. In MySQL, triggers and stored procedures are two powerful functions that can help developers simplify database operation processes and improve efficiency. This article will introduce in detail how to use PHP to write MySQL triggers and stored procedures, and provide specific code examples. 1. Touch
