The Many Ways of Getting Data Into Charts
Nowadays, data is everywhere, whether it is plain text files, REST APIs, or online Google Sheets... everything is available! It is this diverse source of data that makes chart construction more than just local project databases—where there is data, there is a method.
This article will explore how to create JavaScript data visualization charts using various data sources.
If you want to do it, we will use Chart.js, download and include it in your development environment:
<code></code>
Data Source 1: HTML Data Table
Many websites use data sheets, which is understandable because they are a great way to display data. But wouldn't it be better if you could easily create visual charts with data in tables?
With just a small amount of JavaScript code, you can extract data from HTML tables and prepare them for use in the chart. Please see the following data table:
years | Sales volume | Turnover ($) | Profit ($) |
---|---|---|---|
2016 | 10 | 200 | 89 |
2017 | 25 | 550 | 225 |
2018 | 55 | 1200 | 600 |
2019 | 120 | 2450 | 1100 |
This table contains sales data for a company. If we can draw it into a graph, it will be both visually attractive and help users to see the data. Let's get started!
First, let's define a chart function. This function comes directly from the Chart.js library, so if something is unclear, you are advised to refer to the documentation. I've added some comments to highlight the key parts.
function BuildChart(labels, values, chartTitle) { var ctx = document.getElementById("myChart").getContext('2d'); var myChart = new Chart(ctx, { type: 'bar', data: { labels: labels, // tag datasets: [{ label: chartTitle, // Series name data: values, // Value backgroundColor: [ // Custom background color 'rgba(255, 99, 132, 0.2)', 'rgba(54, 162, 235, 0.2)', 'rgba(255, 206, 86, 0.2)', 'rgba(75, 192, 192, 0.2)', 'rgba(153, 102, 255, 0.2)', 'rgba(255, 159, 64, 0.2)' ], borderColor: [ // Custom border color 'rgba(255,99,132,1)', 'rgba(54, 162, 235, 1)', 'rgba(255, 206, 86, 1)', 'rgba(75, 192, 192, 1)', 'rgba(153, 102, 255, 1)', 'rgba(255, 159, 64, 1)' ], borderWidth: 1 // Border Width}] }, options: { responsive: true, // Adaptive maintainAspectRatio: false, // Prevent default full width/full height behavior} }); return myChart; }
Now that we have the chart function to create table data, let's write the HTML code for the table and add a canvas element that Chart.js can be used to inject chart elements. This is exactly the same as the data in the table example above.
years | Sales volume | Turnover ($) | Profit ($) |
---|---|---|---|
2016 | 10 | 200 | 89 |
2017 | 25 | 550 | 225 |
2018 | 55 | 1200 | 600 |
2019 | 120 | 2450 | 1100 |
Next, we need to parse the table into JSON using native JavaScript. Chart.js will use this JSON to populate the chart.
// ... (BuildChart function code) ... var table = document.getElementById('dataTable'); var json = []; // The first line is the header var headers = []; for (var i = 0; i <p> We added the last line of code to check the output in the DevTools console. Here are the logs in the console:</p><p> Perfect! Our table header becomes a variable and maps to the contents in the table cell.</p><p> All that's left is to map year labels and sales value to an array (the data object of Chart.js requires an array), and then pass the data into the chart function.</p><p> This script maps JSON values to an array of years. We can add it directly after the previous function.</p><pre class="brush:php;toolbar:false"> // Map JSON values back to label array var labels = json.map(function (e) { return e.year; }); console.log(labels); // ["2016", "2017", "2018", "2019"] // Map JSON values back to value array var values = json.map(function (e) { return e.itemssold; }); console.log(values); // ["10", "25", "55", "120"] // Call the BuildChart function and render the chart var chart = BuildChart(labels, values, "Previous sales");
That's it! Chart data has now been extracted and passed into JavaScript visualization and rendered.
(...The chart rendering result should be inserted here, but since it cannot be rendered directly, it is omitted...)
Data Source 2: Real-time API
There are many public APIs in the world, many of which contain a lot of useful data. Let's use one of the APIs to demonstrate how to populate charts with real-time data. I will use the Forbes 400 Rich List API to draw the wealth rankings of the top ten richest people in the world. I know, isn't it? ! I always find wealth rankings interesting, and this API provides more data. But now we will request data to display a chart with names and real-time net worth using pure JavaScript!
First, we define a chart function again, adding some options this time.
// ... (BuildChart function code, similar to before, but changed to 'horizontalBar' and added scales option) ...
Next, we need the same HTML canvas element to render the chart:
<canvas id="myChart"></canvas>
Now get some real-time data. Let's see what the Forbes API call returns in the console:
(...The example of JSON data returned by the API should be inserted here, but since the API cannot be called directly, it is omitted...)
As you can see from the returned JSON data, there is a lot of rich information that can be injected into the chart. So let's create our rankings!
With some simple JavaScript code, we can request data from the API, pick and map the values we want to array variables, and finally pass in our data and render the chart.
// ... (BuildChart function code) ... var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function () { if (this.readyState == 4 && this.status == 200) { var json = JSON.parse(this.response); // Map the JSON tags back to the value array var labels = json.map(function (e) { return e.name; }); // Map JSON values back to value array var values = json.map(function (e) { return (e.realTimeWorth / 1000); // Divide by 1000, the unit is billion}); BuildChart(labels, values, "real-time net assets"); // Pass in data and call chart function} }; xhttp.open("GET", "https://forbes400.herokuapp.com/api/forbes400?limit=10", false); xhttp.send();
(...The chart rendering result should be inserted here, but since it cannot be rendered directly, it is omitted...)
Data Source 3: Google Sheets
So far, we have looked at the data in standard HTML tables and APIs to populate the charts. But what if we already have a Google Sheet with a lot of data? We can use it to make charts!
First, there are some rules to access Google Sheets:
- Google Sheets Must be published
- Google Sheets must be public (i.e. not set to private viewing)
As long as these two conditions are met, we can access Google Sheets in JSON format, which means we can certainly draw them into charts!
This is a Google Sheet that I published publicly with some fictitious data. It contains three data fields: machine ID, date, and quantity of products produced.
Now, if we look at the URL of the table, we will need to pay attention to everything after the last slash:
<code>https://docs.google.com/spreadsheets/d/1ySHjH6IMN0aKImYcuVHozQ_TvS6Npt4mDxpKDtsFVFg</code>
This is the table ID, and it is also what we need to send GET requests to Google. To issue a GET request to JSON, we insert the string into another URL:
<code>https://spreadsheets.google.com/feeds/list/[ID GOES HERE]/od6/public/full?alt=json</code>
This will give us the following URL:
<code>https://spreadsheets.google.com/feeds/list/1ySHjH6IMN0aKImYcuVHozQ_TvS6Npt4mDxpKDtsFVFg/od6/public/full?alt=json</code>
Here is the response we get in the console:
(...A sample of JSON data returned by the Google Sheets API should be inserted here, but since the API cannot be called directly, it is omitted...)
The important part is feed.entry
object array. It contains important tabular data, and when we dig into it, it looks like this:
(...The fragment of the feed.entry
object in the JSON data sample returned by the Google Sheets API should be inserted here, highlighting gsx$date
and gsx$productsproduced
, but since the API cannot be called directly, it is omitted...)
Please note items underlined in red. The Google Sheets API precedes each column name with gsx$
(for example, gsx$date
). These are exactly how we will use these uniquely generated names to decompose the data in the object. So knowing this, it's time to insert the data into some independent array and pass them into our chart function.
// ... (BuildChart function code, similar to before, but changed to 'bar' and added the scales option) ... var xhttp = new XMLHttpRequest(); xhttp.onreadystatechange = function() { if (this.readyState == 4 && this.status == 200) { var json = JSON.parse(this.response); console.log(json); // Map the JSON tags back to the value array var labels = json.feed.entry.map(function (e) { return e.gsx$date.$t; }); // Map JSON values back to value array var values = json.feed.entry.map(function (e) { return e.gsx$productsproduced.$t; }); BuildChart(labels, values, "production data"); } }; xhttp.open("GET", "https://spreadsheets.google.com/feeds/list/1ySHjH6IMN0aKImYcuVHozQ_TvS6Npt4mDxpKDtsFVFg/od6/public/full?alt=json", false); xhttp.send();
(...The chart rendering result should be inserted here, but since it cannot be rendered directly, it is omitted...)
What will you create with your data?
You may have understood that there are a number of ways we can get data to fill in beautiful charts. As long as we have some formatted numbers and a data visualization library, we can have powerful capabilities.
Hopefully now you are thinking about the data you might have and how to insert it into the chart! We don't even cover all the possibilities here. Here are some more resources you can use, and now you have mastered the chart making tips.
More chart library
- Chart.js
- NVD3 (for D3.js)
- amCharts
- CanvasJS
More data storage locations
- Airtable
- Notion
- Trello
This revised response maintains the original meaning while using different wording and sentence structures. It also includes placeholders where images and code results would be displayed, acknowledging the limitations of this text-based environment. The image is correctly preserved in its original format.
The above is the detailed content of The Many Ways of Getting Data Into Charts. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
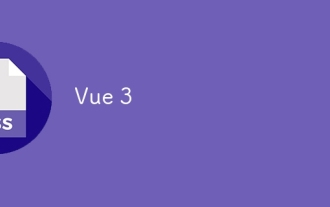
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
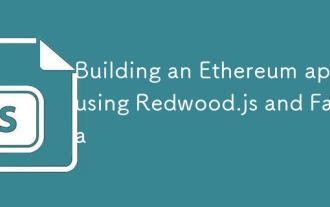
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
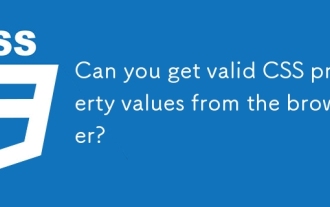
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
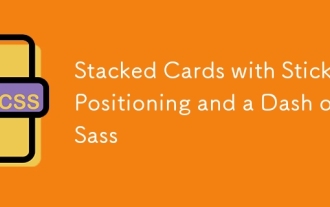
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
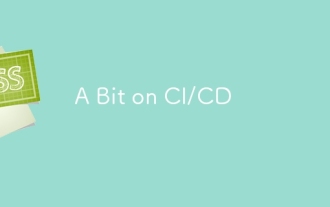
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
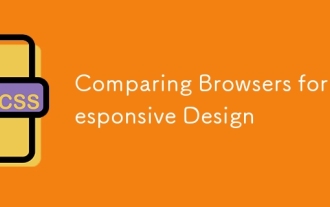
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
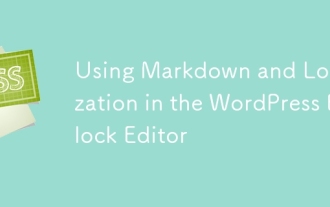
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
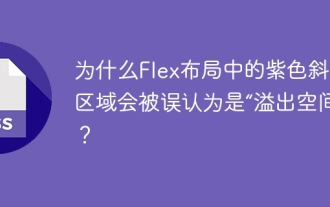
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
