React and HTML: Rendering Data and Handling Events
React efficiently renders data through state and props, and handles user events through the synthesis event system. 1) Use useState to manage state, such as the counter example. 2) Event processing is implemented by adding functions in JSX, such as button clicks. 3) The key attribute is required to render the list, such as the TodoList component. 4) For form processing, useState and e.preventDefault(), such as Form components.
introduction
In modern front-end development, React has become the preferred library for building user interfaces, and its combination with HTML makes data rendering and event processing extremely efficient and intuitive. I chose to write this because the collaborative work of React and HTML has fascinated me throughout my development career, not only simplifying my workflow, but also allowing me to create more interactive applications. Through this article, you will learn how to effectively render data and handle user events in React, which will greatly improve your capabilities and efficiency in front-end development.
Review of basic knowledge
React is a JavaScript library for building user interfaces. It allows developers to build UIs in a modular way through componentization. HTML is the standard markup language for web page content. They are mainly combined in React through JSX syntax, allowing you to write HTML structures directly in JavaScript code.
In React, each component can be regarded as a separate small HTML document, dynamically controlling its content through props and state. Understanding these basic concepts is crucial to mastering subsequent rendering and event handling.
Core concept or function analysis
Data rendering in React
In React, data rendering is implemented through the component's state and props. state is used to manage the state inside a component, while props are data passed from the parent component to the child component. Through these two mechanisms, React can dynamically update the UI to reflect changes in data.
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); Return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count 1)}>Click me</button> </div> ); }
In this simple counter example, the useState
hook is used to manage the state of count
. Whenever the user clicks a button, the value of count
increases and React will automatically re-render the component to reflect the latest state.
Event handling
Event handling in React is similar to traditional HTML event handling, but it is implemented by directly adding event handlers in JSX. React uses a synthetic event system, which means that the event objects are created by React itself, not the browser's native event objects, which ensure consistency across different browsers.
function MyComponent() { function handleClick() { alert('Button was clicked!'); } Return ( <button onClick={handleClick}>Click me</button> ); }
In this example, the handleClick
function is called when the button is clicked. This way of handling events by React makes the code easier to manage and test.
Example of usage
Basic usage
Let's look at a more practical example showing how to render a list in React:
function TodoList({ todos }) { Return ( <ul> {todos.map((todo, index) => ( <li key={index}>{todo}</li> ))} </ul> ); } const todos = ['Learn React', 'Build a project', 'Deploy to production'];
This component takes a todo
array as props and renders each todo item into a list item through map
function. Pay attention to using the key
attribute, which is necessary for React to update the list efficiently.
Advanced Usage
Now, let's look at a more complex example showing how to handle form input and status updates:
function Form() { const [name, setName] = useState(''); const [email, setEmail] = useState(''); const handleSubmit = (e) => { e.preventDefault(); alert(`Submitted: ${name} - ${email}`); }; Return ( <form onSubmit={handleSubmit}> <input type="text" value={name} onChange={(e) => setName(e.target.value)} placeholder="Name" /> <input type="email" value={email} onChange={(e) => setEmail(e.target.value)} placeholder="Email" /> <button type="submit">Submit</button> </form> ); }
In this form component, we use the useState
hook to manage the state of name
and email
, and update these states through the onChange
event handler. The handleSubmit
function will be called when the form is submitted.
Common Errors and Debugging Tips
Common errors when processing data and events in React include:
- Forgot to add
key
attribute to the list item, which will cause React to fail to update the list effectively. - Forgot to call
e.preventDefault()
in the event handler function, which may cause the form to refresh the page when submitted. - Modify the state directly instead of using
setState
oruseState
hook, which will cause React to not detect state changes.
For these questions, my advice is:
- Always add a unique
key
attribute to the list item, and you can usually use a unique identifier in the data. - When processing form submission, remember to call
e.preventDefault()
to prevent the default behavior. - Make sure to update the state through the API provided by React so that React can respond correctly to state changes.
Performance optimization and best practices
In practical applications, it is crucial to optimize the performance of React applications. Here are some optimization tips I have summarized from my experience:
- Use
useMemo
anduseCallback
hooks to optimize performance, especially when there are complex calculations or frequent event processing in the component. - Avoid unnecessary re-rendering and optimize it with the
React.memo
wrapper component. - Use virtual lists (such as
react-window
) to handle rendering of large amounts of data, avoiding rendering all data at once.
Regarding best practices, I suggest:
- Keeping a single responsibility of a component, each component does only one thing, which can improve the maintainability of the code.
- Use meaningful naming and clear annotations to make the code easy to understand and maintain.
- Minimize the depth of nesting of components and simplify structure by improving public logic into higher-level components.
In my development experience, I found that these techniques and practices not only improve the performance of the application, but also make team collaboration more efficient. Hopefully these sharing will help you go further in the journey of React and HTML.
The above is the detailed content of React and HTML: Rendering Data and Handling Events. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
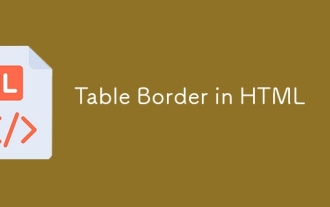
Guide to Table Border in HTML. Here we discuss multiple ways for defining table-border with examples of the Table Border in HTML.
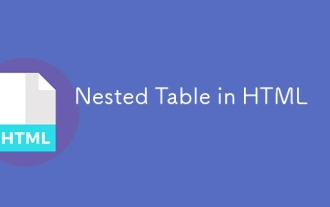
This is a guide to Nested Table in HTML. Here we discuss how to create a table within the table along with the respective examples.
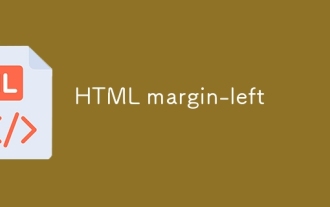
Guide to HTML margin-left. Here we discuss a brief overview on HTML margin-left and its Examples along with its Code Implementation.
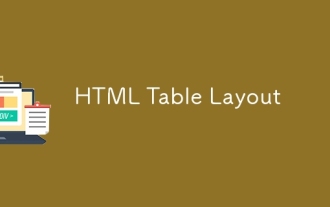
Guide to HTML Table Layout. Here we discuss the Values of HTML Table Layout along with the examples and outputs n detail.
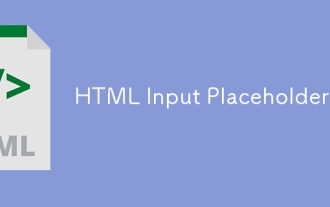
Guide to HTML Input Placeholder. Here we discuss the Examples of HTML Input Placeholder along with the codes and outputs.
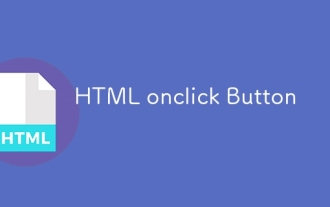
Guide to HTML onclick Button. Here we discuss their introduction, working, examples and onclick Event in various events respectively.
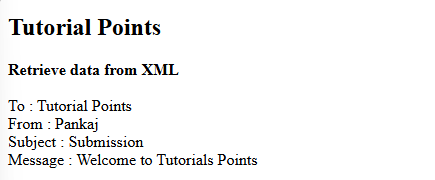
This tutorial demonstrates how to efficiently process XML documents using PHP. XML (eXtensible Markup Language) is a versatile text-based markup language designed for both human readability and machine parsing. It's commonly used for data storage an

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
