PHP and Laravel: Building Server-Side Applications
PHP and Laravel can be used to build efficient server-side applications. 1.PHP is an open source scripting language suitable for web development. 2.Laravel provides routing, controller, Eloquent ORM, Blade template engine and other functions to simplify development. 3. Improve application performance and security through caching, code optimization and security measures. 4. Test and deployment strategies to ensure stable operation of applications.
introduction
When we talk about building server-side applications with PHP and Laravel, we are actually discussing how to use these two powerful tools to efficiently develop modern web applications. As a widely used server-side scripting language, PHP is combined with the elegant framework of Laravel, which allows developers to build applications more easily. The purpose of this article is to deeply explore the application of PHP and Laravel in server-side development, and to capture all the basic knowledge to advanced skills. By reading this article, you will learn how to leverage the power of Laravel to simplify development processes, improve application performance and maintainability.
Basics of PHP and Laravel
Before we dive into it, let’s review the basics of PHP and Laravel. PHP is a common open source scripting language, especially suitable for web development. It can run on the server, generating dynamic web content. Laravel is a PHP-based framework that provides rich functions and elegant syntax to help developers build complex applications faster.
Laravel's design philosophy is to simplify the development process. It provides a series of tools and libraries, such as Eloquent ORM, Blade template engine, Artisan command line tools, etc. These tools greatly improve development efficiency.
Laravel's core features
Routers and controllers
Laravel's routing system is one of its core, which defines how URLs map to specific code in the application. By routing, we can easily handle HTTP requests and direct them to the corresponding controller method.
// Define a simple route Route::get('/home', function () { return view('welcome'); }); <p>// Use controller Route::get('/user/{id}', 'UserController@show');</p>
The controller is responsible for processing the request logic and returning the response. They are an important part of the MVC architecture, making the code organization clearer.
Eloquent ORM
Eloquent ORM is another powerful feature in Laravel that provides a simple and elegant way to interact with a database. Through Eloquent, we can map database tables into model objects and perform CRUD operations.
// Define a User model class User extends Model { protected $table = 'users'; } <p>// Use Eloquent to query $user = User::find(1);</p>
Eloquent not only simplifies database operations, but also supports relational queries, making it easy to handle complex data relationships.
Blade template engine
Blade is Laravel's template engine, which allows developers to embed PHP code in HTML to create dynamic content. Blade's syntax is concise and powerful, and supports conditional statements, loops, template inheritance and other functions.
// A simple Blade template <h1 id="Welcome-name">Welcome, {{ $name }}!</h1> <p>@if ($loggedIn) </p><p>You are logged in.</p> @else <p>Please log in.</p> @endif
Blade not only improves the readability of the template, but also improves the development efficiency.
Build server-side applications using Laravel
Basic application structure
In Laravel, the basic structure of the application includes routes, controllers, models, and views. Together, these components form a complete MVC architecture. By organizing these components reasonably, we can build applications with clear structure and easy maintenance.
Process requests and responses
Laravel provides a variety of ways to handle HTTP requests and generate responses. We can use routing, middleware, request verification and other tools to ensure the security and efficiency of request processing.
// Use middleware to handle requests Route::get('/dashboard', function () { // Only authenticated users can access})->middleware('auth'); <p>// Request verification public function store(Request $request) { $validatedData = $request->validate([ 'title' => 'required|unique:posts|max:255', 'body' => 'required', ]);</p><pre class='brush:php;toolbar:false;'> // Save to database...
}
Database operations
With Eloquent ORM and database migration tools, we can perform database operations efficiently in Laravel. The migration tool allows us to define the database structure in a code manner, which facilitates team collaboration and version control.
// Create a migration file php artisan make:migration create_users_table --create=users <p>// Migrate file content public function up() { Schema::create('users', function (Blueprint $table) { $table->id(); $table->string('name'); $table->string('email')->unique(); $table->timestamps(); }); }</p>
Error handling and logging
Laravel provides powerful error handling and logging capabilities to help us monitor and debug applications. By configuring the log driver and exception handler, we can easily catch and log errors in our application.
// Configure log driver 'channels' => [ 'stack' => [ 'driver' => 'stack', 'channels' => ['single'], 'ignore_exceptions' => false, ], <pre class='brush:php;toolbar:false;'>'single' => [ 'driver' => 'single', 'path' => storage_path('logs/laravel.log'), 'level' => 'debug', ],
],
Performance optimization and best practices
Performance optimization and best practices are the key points we need to focus on when building applications using Laravel. Here are some suggestions:
cache
Laravel provides a variety of caching mechanisms, such as memory cache, file cache and database cache. Rational use of cache can significantly improve the response speed of applications.
// Use cache $value = Cache::remember('key', $minutes, function () { return DB::table('users')->count(); });
Code optimization
By optimizing the code structure, reducing redundant queries, using Eloquent lazy loading and other methods, we can improve the performance of our application.
// Avoid N 1 query problem $books = Book::with('author')->get();
Security
During the development process, security is always something we need to focus on. Laravel provides a variety of security measures, such as CSRF protection, input verification, SQL injection protection, etc., to ensure the security of the application.
// CSRF protection<form method="POST" action="/profile"> @csrf ... </form>
Testing and deployment
Finally, a good testing and deployment strategy is the key to ensuring the stable operation of the application. Laravel provides a powerful testing framework and deployment tools to help us perform unit testing, integration testing and continuous integration.
// Write a simple test public function testBasicExample() { $response = $this->get('/'); <pre class='brush:php;toolbar:false;'>$response->assertStatus(200);
}
Summarize
Through this article, we have a deeper understanding of how to build server-side applications using PHP and Laravel. From basics to advanced features to performance optimization and best practices, each link provides us with a wealth of tools and methods. I hope these contents can help you develop more easily with Laravel and build efficient, safe and easy to maintain applications.
The above is the detailed content of PHP and Laravel: Building Server-Side Applications. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
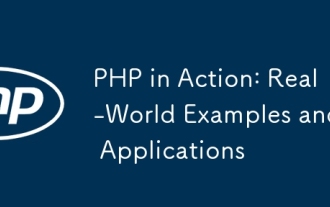
PHP is widely used in e-commerce, content management systems and API development. 1) E-commerce: used for shopping cart function and payment processing. 2) Content management system: used for dynamic content generation and user management. 3) API development: used for RESTful API development and API security. Through performance optimization and best practices, the efficiency and maintainability of PHP applications are improved.
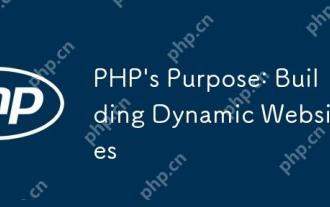
PHP is used to build dynamic websites, and its core functions include: 1. Generate dynamic content and generate web pages in real time by connecting with the database; 2. Process user interaction and form submissions, verify inputs and respond to operations; 3. Manage sessions and user authentication to provide a personalized experience; 4. Optimize performance and follow best practices to improve website efficiency and security.
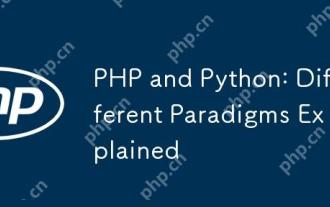
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
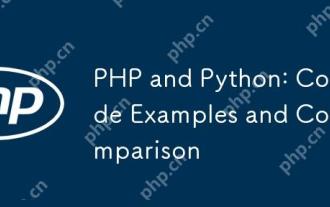
PHP and Python have their own advantages and disadvantages, and the choice depends on project needs and personal preferences. 1.PHP is suitable for rapid development and maintenance of large-scale web applications. 2. Python dominates the field of data science and machine learning.
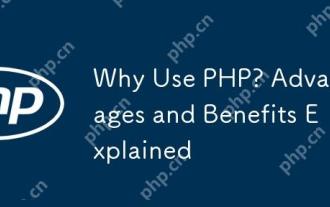
The core benefits of PHP include ease of learning, strong web development support, rich libraries and frameworks, high performance and scalability, cross-platform compatibility, and cost-effectiveness. 1) Easy to learn and use, suitable for beginners; 2) Good integration with web servers and supports multiple databases; 3) Have powerful frameworks such as Laravel; 4) High performance can be achieved through optimization; 5) Support multiple operating systems; 6) Open source to reduce development costs.
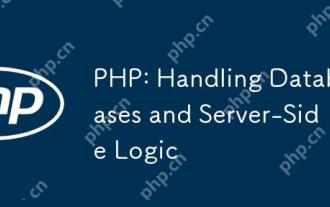
PHP uses MySQLi and PDO extensions to interact in database operations and server-side logic processing, and processes server-side logic through functions such as session management. 1) Use MySQLi or PDO to connect to the database and execute SQL queries. 2) Handle HTTP requests and user status through session management and other functions. 3) Use transactions to ensure the atomicity of database operations. 4) Prevent SQL injection, use exception handling and closing connections for debugging. 5) Optimize performance through indexing and cache, write highly readable code and perform error handling.
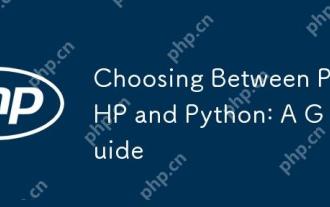
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
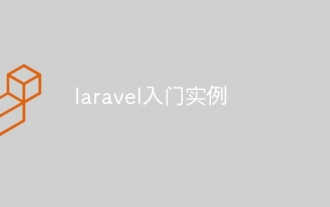
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
