Different Approaches for Creating a Staggered Animation
Basic animation is simple: define keyframes, name animations, and apply them to elements. But sometimes more complex techniques are required to achieve the ideal animation effect. For example, a sound equalizer might use the same animation for each bar, but they are interleaved to create the illusion of independent animations.
Recently when building a dashboard, I want the items of one of the widgets to be displayed in an interlaced animation.
Like the sound equalizer above, I started using the :nth-child
selector. I use an unordered list (<ul></ul>
) As the parent container, give it a class and use the :nth-child
pseudo-selector to offset the animation of each list item by animation-delay
.
.my-list li { animation: my-animation 300ms ease-out; } .my-list li:nth-child(1) { animation-delay: 100ms; } .my-list li:nth-child(2) { animation-delay: 200ms; } .my-list li:nth-child(3) { animation-delay: 300ms; } /* and so on*/
This technique does stagger items really well, especially if you know how many items are in the list at any given time. However, this approach fails when the number of items is unpredictable (this is the case with the widgets I built for the dashboard). I really don't want to return to this code every time the number of items in the list changes, so I wrote a quick Sass loop that can handle up to 50 items and add animation latency as each item increases:
.my-list { li { animation: my-animation 300ms ease-out; @for $i from 1 through 50 { &:nth-child(#{$i}) { animation-delay: 100ms * $i; } } } }
This should solve the problem! However, it feels too clumsy. Of course, it won't add too much weight to the file, but you know that compiled CSS will contain many unused selectors, such as nth-child(45)
.
There must be a better way. Usually I would turn to JavaScript to find all the projects and add delays, but… this time I spent some time exploring if it could be done alone with CSS.
How about CSS counters?
The first thing that comes to mind is to use a CSS counter with the calc()
function:
.my-list { counter-reset: my-counter; } .my-list li { counter-increment: my-counter; animation-delay: calc(counter(my-counter) * 100ms); }
Unfortunately, this doesn't work, as the specification states that the counter cannot be used in calc()
:
The component of
calc()
expression can be a literal value orattr()
orcalc()
expression.
It turns out that some people like the idea, but it is not out of the draft stage yet.
How about data attributes?
After reading the excerpt from the specification, I learned that calc()
can use attr()
. And, according to the CSS value and unit specification:
In CSS3, the
attr()
expression can return many different types of values
This reminds me; maybe the data attributes can solve the problem.
- Item 1
- Item 2
- Item 3
- Item 4
.my-list li { animation-delay: calc(attr(data-count) * 150ms); }
But my hopes are dashed because this browser support is very bad!
This browser supports data from Caniuse, which contains more details. The number indicates that the browser supports this feature in this version and later.
desktop
Mobile/Tablet PC
So, back to the drawing board.
How about custom properties?
My next idea is to use CSS to customize properties. It's not pretty, but it works?
It turns out it is flexible, too. For example, animations can be reversed:
It can also do something completely random and animate elements at the same time:
We can even go a step further and do a diagonal swing:
Browser support is not that bad ( pop up Internet Explorer ).
This browser supports data from Caniuse, which contains more details. The number indicates that the browser supports this feature in this version and later.
desktop
Mobile/Tablet PC
A great feature of CSS is that it ignores things it doesn't understand, thanks to cascading. This means that everything will be animate into the view together. If this is not your style, you can add a feature query to override the default animation:
.my-list li { animation: fallback-animation; } @supports (--variables) { .my-list li { animation: fancy-animation; animation-delay: calc(var(--animation-order) * 100ms); } }
Native CSS Long live
The more I paused to ask myself if I needed JavaScript, the more I was surprised at what CSS itself could do. Of course, if the CSS counter can be used in the calc()
function, that would be a very elegant solution. But for now, inline custom properties provide a powerful and flexible way to solve this problem.
The above is the detailed content of Different Approaches for Creating a Staggered Animation. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
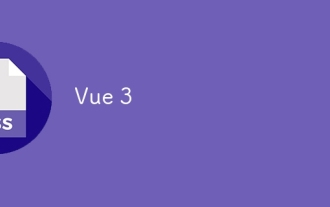
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
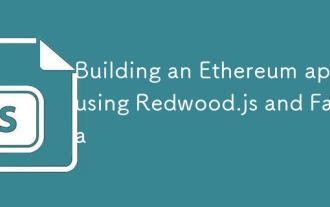
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
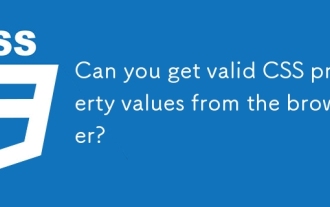
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
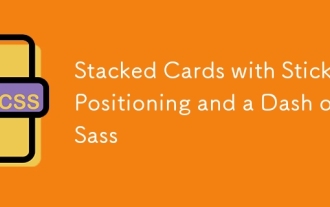
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
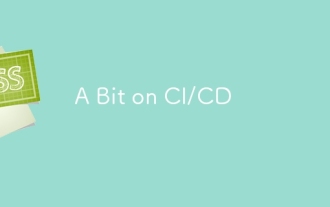
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
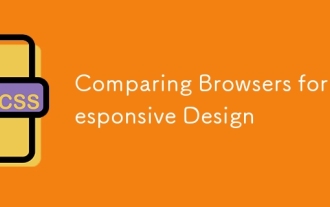
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
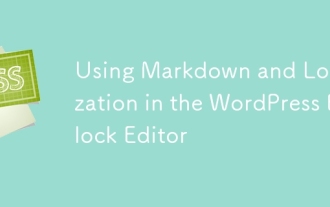
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
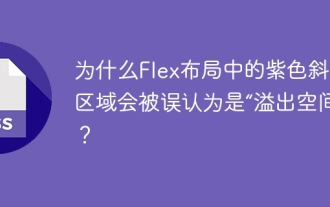
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
