C# and the .NET Runtime: How They Work Together
C# and .NET runtime work closely together to empower developers to efficient, powerful and cross-platform development capabilities. 1) C# is a type-safe and object-oriented programming language designed to integrate seamlessly with the .NET framework. 2) The .NET runtime manages the execution of C# code, provides garbage collection, type safety and other services, and ensures efficient and cross-platform operation.
introduction
In the programming world, C# and .NET runtime are like a pair of golden partners, and their close collaboration allows developers to create efficient, powerful, and cross-platform applications. Today we will dive into how this pair works and how they give us so many possibilities. You will learn about the core features of the .NET runtime, the uniqueness of the C# language, and how they work together to improve development efficiency and performance.
Review of basic knowledge
C# is a modern object-oriented programming language released by Microsoft in 2000 to integrate seamlessly with the .NET framework. The .NET runtime is an environment that manages code execution, which provides garbage collection, type safety, and a range of libraries and services, allowing developers to quickly build applications. Understanding the relationship between the two can help us better utilize their advantages.
Core concept or function analysis
Definition and function of C# and .NET runtime
C# is a type-safe and object-oriented programming language that enables developers to write code in a concise and powerful way. The .NET runtime is the execution environment of C# code, which is responsible for compiling C# code into an intermediate language (IL) and then converting it into local machine code execution through instant compilation (JIT). The .NET runtime also provides a range of services such as garbage collection, exception handling, and type safety, which greatly simplify the development process.
Take a look at this simple C# code that shows how to use C# in the .NET runtime:
using System; class Program { static void Main(string[] args) { Console.WriteLine("Hello, .NET Runtime!"); } }
How it works
When we write C# code, first it will be compiled into an intermediate language (IL). .NET runtime uses the instant compiler (JIT) to convert IL to platform-specific machine code when the program is running, meaning your C# code can run on any platform that supports .NET. This process not only provides cross-platform capabilities, but also improves execution efficiency, because the JIT compiler can be optimized based on the operating environment.
The .NET runtime is also responsible for memory management, and automatically recycles objects that are no longer used through the garbage collection mechanism, thus avoiding the problem of memory leakage. At the same time, the .NET runtime provides a powerful type safety mechanism to ensure that the code does not experience type-related errors when it is run.
Example of usage
Basic usage
Let's look at a simple example showing how to use the .NET runtime features in C#:
using System; class Program { static void Main(string[] args) { // Garbage collection using .NET string message = "This will be garbage collected"; Console.WriteLine(message); // Type-safe using .NET int number = 10; Console.WriteLine(number); } }
In this example, we show how to use garbage collection and type safety features of the .NET runtime. Strings and integers are managed by the .NET runtime to ensure effective memory usage and type safety.
Advanced Usage
Now let's look at a more advanced example showing how to take advantage of asynchronous programming in the .NET runtime:
using System; using System.Threading.Tasks; class Program { static async Task Main(string[] args) { await DoSomethingAsync(); Console.WriteLine("Async operation completed"); } static async Task DoSomethingAsync() { await Task.Delay(1000); // Simulate an asynchronous operation Console.WriteLine("Async task done"); } }
This example shows how to use the asynchronous programming model in the .NET runtime, allowing us to write efficient non-blocking code to improve the responsiveness of our application.
Common Errors and Debugging Tips
Common problems may occur when running with C# and .NET, such as memory leaks, deadlocks, or type conversion errors. Here are some debugging tips:
- Use .NET's memory analysis tool to detect memory leaks
- Use the debugger to track code execution and find deadlocks or performance bottlenecks
- Double-check type conversion to make sure you use the correct conversion method, such as
as
oris
keywords
Performance optimization and best practices
In practical applications, it is crucial to optimize the performance of C# and .NET runtimes. Here are some optimization tips and best practices:
- Use
using
statements to ensure the correct release of resources and avoid resource leakage - Use asynchronous programming rationally to improve the concurrency and responsiveness of applications
- Identify and optimize performance bottlenecks with .NET's performance analysis tools
For example, compare the performance differences using synchronous and asynchronous methods:
using System; using System.Diagnostics; using System.Threading.Tasks; class Program { static void Main(string[] args) { // Synchronous method var swSync = Stopwatch.StartNew(); DoSomethingSync(); swSync.Stop(); Console.WriteLine($"Sync operation took {swSync.ElapsedMilliseconds} ms"); // Asynchronous method var swAsync = Stopwatch.StartNew(); DoSomethingAsync().Wait(); swAsync.Stop(); Console.WriteLine($"Async operation took {swAsync.ElapsedMilliseconds} ms"); } static void DoSomethingSync() { for (int i = 0; i < 1000000; i ) { // Simulate a time-consuming operation} } static async Task DoSomethingAsync() { await Task.Run(() => { for (int i = 0; i < 1000000; i ) { // Simulate a time-consuming operation} }); } }
With this example, we can see how asynchronous programming can significantly improve the performance of an application.
Overall, the close collaboration between C# and .NET runtime provides developers with powerful tools and flexibility. By understanding how they interact, we can better leverage their strengths and create efficient and reliable applications. Hopefully this article will help you understand the workings of C# and .NET runtimes and apply this knowledge in actual development.
The above is the detailed content of C# and the .NET Runtime: How They Work Together. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
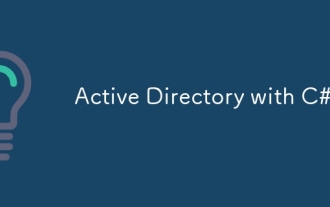
Guide to Active Directory with C#. Here we discuss the introduction and how Active Directory works in C# along with the syntax and example.
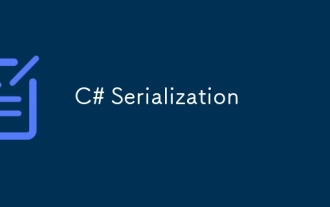
Guide to C# Serialization. Here we discuss the introduction, steps of C# serialization object, working, and example respectively.
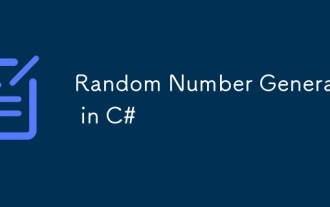
Guide to Random Number Generator in C#. Here we discuss how Random Number Generator work, concept of pseudo-random and secure numbers.
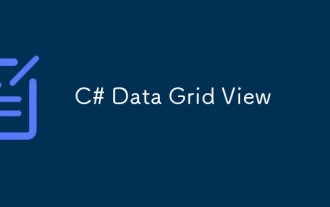
Guide to C# Data Grid View. Here we discuss the examples of how a data grid view can be loaded and exported from the SQL database or an excel file.
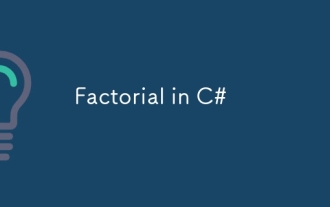
Guide to Factorial in C#. Here we discuss the introduction to factorial in c# along with different examples and code implementation.
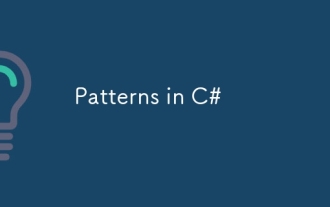
Guide to Patterns in C#. Here we discuss the introduction and top 3 types of Patterns in C# along with its examples and code implementation.
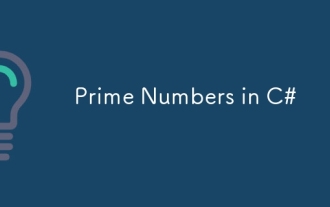
Guide to Prime Numbers in C#. Here we discuss the introduction and examples of prime numbers in c# along with code implementation.
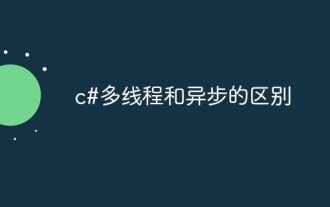
The difference between multithreading and asynchronous is that multithreading executes multiple threads at the same time, while asynchronously performs operations without blocking the current thread. Multithreading is used for compute-intensive tasks, while asynchronously is used for user interaction. The advantage of multi-threading is to improve computing performance, while the advantage of asynchronous is to not block UI threads. Choosing multithreading or asynchronous depends on the nature of the task: Computation-intensive tasks use multithreading, tasks that interact with external resources and need to keep UI responsiveness use asynchronous.
