Solve the challenges of RabbitMQ message consumption using Composer
In project development, I need to consume messages from the RabbitMQ message queue and execute different processing logic based on the message content, and finally store the processing results in MySQL and Elasticsearch. This process seems simple, but it is full of challenges in practice. First, the message in the message queue only contains id
in MySQL and some extra information, which means I need to read the details from MySQL before processing and storing. In addition, different projects may have different processing logic and storage requirements, which makes the reusability and maintainability of the code very important.
To solve these problems, I chose to use the mysic/phpamqplib-consumer
library. This library provides a flexible framework that allows me to customize message consumption and processing logic based on different project needs. Here are the steps to install and configure this library using Composer:
-
Installing the library : Installing
mysic/phpamqplib-consumer
through Composer is very simple, just execute it on the command line:<code>composer require mysic/phpamqplib-consumer</code>
Copy after login -
Configure the project structure : After the installation is completed, organize the project code according to the directory structure of the library. The core files and classes are located in
core/
directory, while the specific business logic of each project is placed in the corresponding folder undertask/
directory. For example:<code>/ core/ Db.php Dispatcher.php MqConnector.php Processor.php Storage.php task/ project_1/ config/ processor/ storage/ project_n/ config/ processor/ storage/ run.php</code>
Copy after login -
Configuration file : In the
config/
folder in each project directory, configure the relevant parameters of the data source, message queue, and data storage. For example:<code>config/ db.php messageQueue.php storage.php</code>
Copy after login -
Write processing logic : In
processor/
folder, write specific message processing logic. For example, for the logic that handles the storage of documents into Elasticsearch, it can be implemented inDocument.php
:<code class="php">// Document.php class Document extends Processor { public function process($message) { // 从MySQL中读取详细信息$data = $this->db->fetch($message['id']); // 处理数据并存储到Elasticsearch $this->storage->save($data, $message['extra']); } }</code>
Copy after login -
Run Consumer : Finally, start the message consumer through the
run.php
file, specifying the project name, processor name, and storage name:<code>php run.php project_name processor_name storage_name</code>
Copy after login
After using the mysic/phpamqplib-consumer
library, I was able to easily manage and extend the message consumption logic. Its modular design allows me to flexibly add new processors and memory according to the needs of different projects, greatly improving the maintainability and reusability of the code. In addition, the Dispatcher
class provided by the library can effectively manage the distribution and processing of messages, ensuring the stability and efficiency of the message queue.
In general, mysic/phpamqplib-consumer
library not only solves the RabbitMQ message consumption problem encountered in my project, but also provides a solid foundation for future expansion. If you are also dealing with similar message queue consumption needs, you might as well try this library, it will help you get twice the result with half the effort.
The above is the detailed content of Solve the challenges of RabbitMQ message consumption using Composer. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
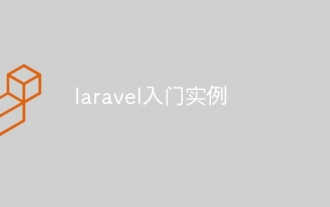
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
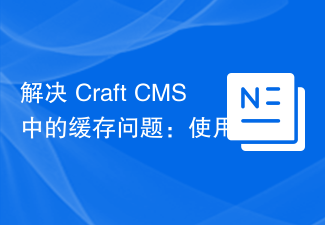
When developing websites using CraftCMS, you often encounter resource file caching problems, especially when you frequently update CSS and JavaScript files, old versions of files may still be cached by the browser, causing users to not see the latest changes in time. This problem not only affects the user experience, but also increases the difficulty of development and debugging. Recently, I encountered similar troubles in my project, and after some exploration, I found the plugin wiejeben/craft-laravel-mix, which perfectly solved my caching problem.
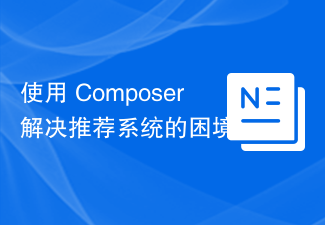
When developing an e-commerce website, I encountered a difficult problem: how to provide users with personalized product recommendations. Initially, I tried some simple recommendation algorithms, but the results were not ideal, and user satisfaction was also affected. In order to improve the accuracy and efficiency of the recommendation system, I decided to adopt a more professional solution. Finally, I installed andres-montanez/recommendations-bundle through Composer, which not only solved my problem, but also greatly improved the performance of the recommendation system. You can learn composer through the following address:
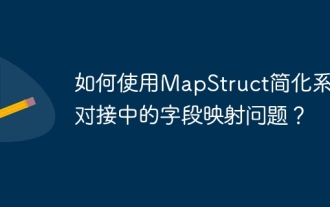
Field mapping processing in system docking often encounters a difficult problem when performing system docking: how to effectively map the interface fields of system A...
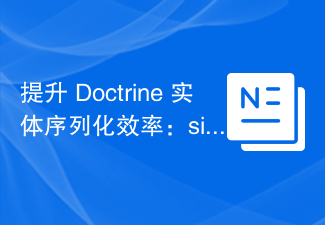
I had a tough problem when working on a project with a large number of Doctrine entities: Every time the entity is serialized and deserialized, the performance becomes very inefficient, resulting in a significant increase in system response time. I've tried multiple optimization methods, but it doesn't work well. Fortunately, by using sidus/doctrine-serializer-bundle, I successfully solved this problem, significantly improving the performance of the project.
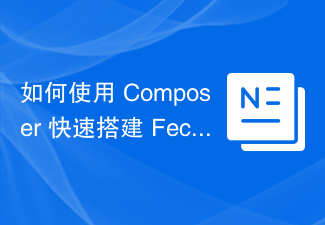
When developing an e-commerce platform, it is crucial to choose the right framework and tools. Recently, when I was trying to build a feature-rich e-commerce website, I encountered a difficult problem: how to quickly build a scalable and fully functional e-commerce platform. I tried multiple solutions and ended up choosing Fecmall's advanced project template (fecmall/fbbcbase-app-advanced). By using Composer, this process becomes very simple and efficient. Composer can be learned through the following address: Learning address
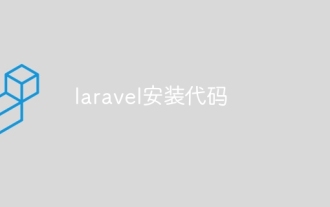
To install Laravel, follow these steps in sequence: Install Composer (for macOS/Linux and Windows) Install Laravel Installer Create a new project Start Service Access Application (URL: http://127.0.0.1:8000) Set up the database connection (if required)
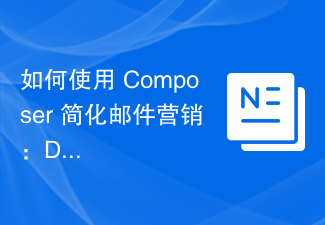
I'm having a tricky problem when doing a mail marketing campaign: how to efficiently create and send mail in HTML format. The traditional approach is to write code manually and send emails using an SMTP server, but this is not only time consuming, but also error-prone. After trying multiple solutions, I discovered DUWA.io, a simple and easy-to-use RESTAPI that helps me create and send HTML mail quickly. To further simplify the development process, I decided to use Composer to install and manage DUWA.io's PHP library - captaindoe/duwa.
