React and Frontend Development: A Comprehensive Overview
React is a JavaScript library developed by Facebook for building user interfaces. 1. It adopts componentized and virtual DOM technology to improve the efficiency and performance of UI development. 2. The core concepts of React include componentization, state management (such as useState and useEffect) and the working principle of virtual DOM. 3. In practical applications, React supports from basic component rendering to advanced asynchronous data processing. 4. Common errors such as forgetting to add key attributes or incorrect status updates can be debugged through React DevTools and logs. 5. Performance optimization and best practices include using React.memo, code segmentation and keeping code readable and maintainable.
introduction
In today's web development world, React has become the backbone of front-end development. As an experienced front-end developer, I know that React not only changes the way we build user interfaces, but also promotes the development of the entire front-end ecosystem. This article will take you into the deepest understanding of React and its applications in front-end development, from basics to advanced techniques, helping you master the essence of this powerful tool. After reading this article, you will be able to understand the core concepts of React, master its best practices, and be able to flexibly apply them in real-life projects.
Review of basic knowledge
React is a JavaScript library developed by Facebook to build user interfaces. It adopts the idea of componentization and splits the UI into independent, reusable components. At the heart of React is a virtual DOM, which improves performance through efficient DOM operations. In addition, React also introduced JSX syntax, making writing HTML in JavaScript more intuitive.
In front-end development, understanding HTML, CSS, and JavaScript is essential. React builds on these foundations, further simplifying the development process. Familiar with these basic technologies will help you better understand how React works and benefits.
Core concept or function analysis
React's componentization and state management
The core idea of React is componentization. Each component is an independent unit, responsible for its own state and rendering logic. This makes the code more modular and maintainable. State management is another key concept in React. With hook functions such as useState
and useEffect
, developers can easily manage the state and side effects of components.
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); Return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count 1)}> Click me </button> </div> ); }
This simple counter component shows how to use useState
to manage state. Each time the button is clicked, the value of count
increases and the component is triggered to re-render.
How React works
React works mainly relies on virtual DOM and reconciliation algorithms. A virtual DOM is a lightweight JavaScript object that describes the structure of a real DOM. When the state of the component changes, React generates a new virtual DOM tree and compares it with the old virtual DOM tree to find the difference (called "diffing"). React then updates only those DOM nodes that actually change, thus improving performance.
This approach, while very efficient in most cases, has some potential pitfalls. For example, frequent re-rendering can cause performance problems, especially in large applications. To avoid this problem, you can use React.memo
or shouldComponentUpdate
to optimize the rendering of components.
Example of usage
Basic usage
Let's look at a simple React component that shows a list of users:
import React from 'react'; function UserList({ users }) { Return ( <ul> {users.map(user => ( <li key={user.id}>{user.name}</li> ))} </ul> ); } const users = [ { id: 1, name: 'Alice' }, { id: 2, name: 'Bob' }, { id: 3, name: 'Charlie' } ]; function App() { return <UserList users={users} />; }
This example shows how to pass data using props, and how to render a list using map
function. Note that each list item requires a unique key
attribute to help React update the DOM efficiently.
Advanced Usage
In more complex scenarios, we may need to deal with asynchronous data loading and error handling. Here is an example of using useEffect
and useState
to load user data:
import React, { useState, useEffect } from 'react'; function UserList() { const [users, setUsers] = useState([]); const [loading, setLoading] = useState(true); const [error, setError] = useState(null); useEffect(() => { fetch('https://api.example.com/users') .then(response => response.json()) .then(data => { setUsers(data); setLoading(false); }) .catch(err => { setError(err.message); setLoading(false); }); }, []); if (loading) return <div>Loading...</div>; if (error) return <div>Error: {error}</div>; Return ( <ul> {users.map(user => ( <li key={user.id}>{user.name}</li> ))} </ul> ); }
This example shows how to use useEffect
to handle side effects, and how to manage loading and error states. This is a more realistic scenario that demonstrates the application of React in real projects.
Common Errors and Debugging Tips
Common errors when using React include:
- Forgot to add key attributes : When rendering a list, each element requires a unique key attribute. Without a key, React may experience performance issues when updating the list.
- Incorrect state update : When updating the state, make sure to use
setState
in the form of a function to avoid closure problems. For example,setCount(count 1)
may cause unexpected behavior and should be usedsetCount(prevCount => prevCount 1)
.
When debugging React applications, you can use React DevTools to view component trees and states. In addition, console.log
and console.error
can also help you track the execution process and error information of your code.
Performance optimization and best practices
In real projects, optimizing the performance of React applications is crucial. Here are some common optimization tips:
- Use
React.memo
: For pure function components, you can useReact.memo
to avoid unnecessary re-rendering. - Avoid unnecessary re-rendering : Use
shouldComponentUpdate
orReact.PureComponent
to optimize the rendering logic of the component. - Code segmentation : Use
React.lazy
andSuspense
to implement code segmentation to reduce the initial loading time.
import React, { lazy, Suspense } from 'react'; const UserList = lazy(() => import('./UserList')); function App() { Return ( <Suspense fallback={<div>Loading...</div>}> <UserList /> </Suspense> ); }
This example shows how to use React.lazy
and Suspense
to implement code segmentation to improve application loading speed.
When writing React code, it is also very important to keep the code readable and maintainable. Here are some best practices:
- Use meaningful component names : Component names should clearly express their functions, such as
UserList
instead ofList
. - Keep a single responsibility of a component : Each component should be responsible for only one function, avoiding components being overly complex.
- Type checking with PropTypes : During the development process, using PropTypes can help you catch type errors and improve the robustness of your code.
import PropTypes from 'prop-types'; function UserList({ users }) { // ... } UserList.propTypes = { users: PropTypes.arrayOf( PropTypes.shape({ id: PropTypes.number.isRequired, name: PropTypes.string.isRequired }) ).isRequired };
This example shows how to use PropTypes to perform type checking to ensure that the incoming users
attribute is consistent with the expected structure.
In short, React is a powerful tool that can help you build efficient and maintainable front-end applications. By understanding its core concepts and best practices, you will be able to flexibly use React in real-world projects to create a great user experience.
The above is the detailed content of React and Frontend Development: A Comprehensive Overview. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
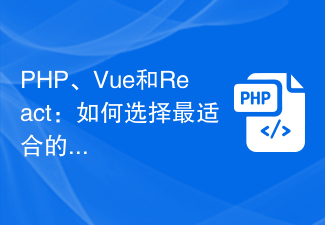
PHP, Vue and React: How to choose the most suitable front-end framework? With the continuous development of Internet technology, front-end frameworks play a vital role in Web development. PHP, Vue and React are three representative front-end frameworks, each with its own unique characteristics and advantages. When choosing which front-end framework to use, developers need to make an informed decision based on project needs, team skills, and personal preferences. This article will compare the characteristics and uses of the three front-end frameworks PHP, Vue and React.
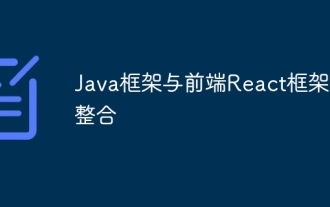
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
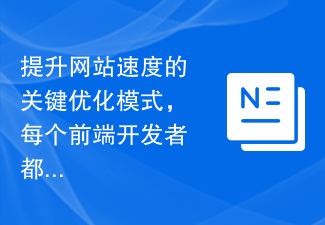
A must-have for front-end developers: master these optimization modes and make your website fly! With the rapid development of the Internet, websites have become one of the important channels for corporate promotion and communication. A well-performing, fast-loading website not only improves user experience, but also attracts more visitors. As a front-end developer, it is essential to master some optimization patterns. This article will introduce some commonly used front-end optimization techniques to help developers better optimize their websites. Compressed files In website development, commonly used file types include HTML, CSS and J
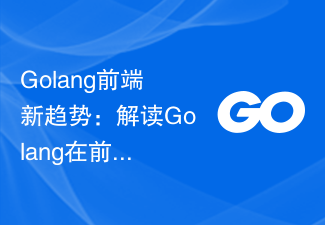
New trends in Golang front-end: Interpretation of the application prospects of Golang in front-end development. In recent years, the field of front-end development has developed rapidly, and various new technologies have emerged in an endless stream. As a fast and reliable programming language, Golang has also begun to emerge in front-end development. Golang (also known as Go) is a programming language developed by Google. It is famous for its efficient performance, concise syntax and powerful functions, and is gradually favored by front-end developers. This article will explore the application of Golang in front-end development.

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
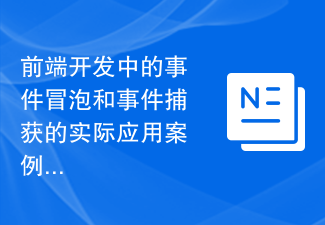
Application cases of event bubbling and event capturing in front-end development Event bubbling and event capturing are two event delivery mechanisms that are often used in front-end development. By understanding and applying these two mechanisms, we can handle interactive behaviors in the page more flexibly and improve user experience. This article will introduce the concepts of event bubbling and event capturing, and combine them with specific code examples to demonstrate their application cases in front-end development. 1. The concepts of event bubbling and event capture. Event bubbling (EventBubbling) refers to the process of triggering an element.
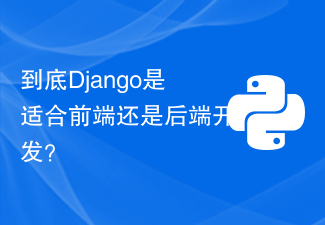
Django is a web application framework built in Python that helps developers quickly build high-quality web applications. The development process of Django usually involves two aspects: front-end and back-end, but which aspect of development is Django more suitable for? This article will explore the advantages of Django in front-end and back-end development and provide specific code examples. Advantages of Django in back-end development Django, as a back-end framework, has many advantages, as follows:
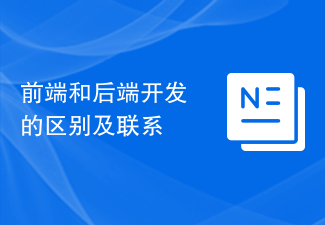
Front-end and back-end development are two essential aspects of building a complete web application. There are obvious differences between them, but they are closely related. This article will analyze the differences and connections between front-end and back-end development. First, let’s take a look at the specific definitions and tasks of front-end development and back-end development. Front-end development is mainly responsible for building the user interface and user interaction part, that is, what users see and operate in the browser. Front-end developers typically use technologies such as HTML, CSS, and JavaScript to implement the design and functionality of web pages
