The Power of React in HTML: Modern Web Development
The application of React in HTML improves the efficiency and flexibility of web development through componentization and virtual DOM. 1) React componentization idea breaks down the UI into reusable units to simplify management. 2) Virtual DOM optimization performance, minimize DOM operations through diffing algorithm. 3) JSX syntax allows writing HTML in JavaScript to improve development efficiency. 4) Use the useState hook to manage state and implement dynamic content updates. 5) Optimization strategies include using React.memo and useCallback to reduce unnecessary rendering.
introduction
In modern web development, React, as a powerful and flexible library, is reshaping the way we build user interfaces. You may have heard of React, but do you really understand its power in HTML? This article will take you into the deep understanding of React's application in HTML, exploring its advantages and best practices. Reading, you will learn how to use React to create efficient, dynamic and maintainable web applications.
Review of basic knowledge
React is a JavaScript library developed by Facebook to simplify user interface development. It allows developers to handle UI updates and management more efficiently through the concept of componentization and virtual DOM. HTML is the skeleton of web pages, and React is the tool to make this skeleton flexible and vivid.
In React, we use JSX, a syntax similar to HTML, but it is closer to JavaScript. This allows us to write HTML structures directly in JavaScript code, greatly simplifying the development process.
Core concept or function analysis
The definition and role of React in HTML
The core of React is its componentization idea, and each component can be regarded as an independent HTML unit. By breaking the UI into reusable components, we can manage and maintain complex interfaces more easily. React is that it allows us to describe the UI in a declarative manner, so that state changes can be automatically reflected on the interface.
A simple React component example:
function HelloWorld() { return <h1 id="Hello-World">Hello, World!</h1>; }
This component returns an HTML <h1>
tag, showing how React seamlessly combines JavaScript with HTML.
How React works
How React works mainly depends on virtual DOM and component lifecycle. A virtual DOM is a lightweight in-memory representation that allows React to calculate the smallest DOM operations to update the interface, thereby improving performance. The component life cycle defines the behavior of the component at different stages, such as mount, update, and uninstall.
In practical applications, React compares the differences between virtual DOM and actual DOM through diffing algorithm, and then updates only the necessary parts. This method greatly reduces DOM operations and improves application performance.
Example of usage
Basic usage
Let's look at a simple React application that shows a counter:
import React, { useState } from 'react'; function Counter() { const [count, setCount] = useState(0); Return ( <div> <p>You clicked {count} times</p> <button onClick={() => setCount(count 1)}>Click me</button> </div> ); }
This example shows how to use React's useState
hook to manage state and render HTML elements through JSX.
Advanced Usage
Now, let's look at a more complex example using conditional rendering and list rendering to show a to-do list:
import React, { useState } from 'react'; function TodoList() { const [todos, setTodos] = useState([]); const [input, setInput] = useState(''); const addTodo = () => { if (input.trim()) { setTodos([...todos, input.trim()]); setInput(''); } }; Return ( <div> <input value={input} onChange={(e) => setInput(e.target.value)} placeholder="Enter a todo" /> <button onClick={addTodo}>Add Todo</button> <ul> {todos.map((todo, index) => ( <li key={index}>{todo}</li> ))} </ul> </div> ); }
This example shows how to use useState
to manage multiple states, and how to use conditional rendering and list rendering to dynamically generate HTML content.
Common Errors and Debugging Tips
Common errors when using React include not handling status updates correctly, not using key
attributes in the list, and incorrect lifecycle management. Here are some debugging tips:
- Use React DevTools to check component tree and state changes.
- Make sure to provide unique
key
attributes for each element when rendering the list to avoid performance issues. - Understand and correctly use lifecycle methods such as
useEffect
hooks to avoid unnecessary side effects.
Performance optimization and best practices
In practical applications, it is crucial to optimize the performance of React applications. Here are some optimization strategies:
- Use
React.memo
oruseMemo
to avoid unnecessary re-rendering. - Optimize the function's pass through the
useCallback
hook to avoid unnecessary recreation. - Use code segmentation and lazy loading to reduce initial loading time.
In terms of best practice, maintain a single responsibility of the component and ensure the readability and maintainability of the code. Here is an optimized counter component example:
import React, { useState, useCallback } from 'react'; const Counter = React.memo(() => { const [count, setCount] = useState(0); const increment = useCallback(() => { setCount((prevCount) => prevCount 1); }, []); Return ( <div> <p>You clicked {count} times</p> <button onClick={increment}>Click me</button> </div> ); }); export default Counter;
This example shows how to use React.memo
and useCallback
to optimize component performance.
When using React, I found a common misunderstanding that is over-optimization. Premature optimization may lead to increased code complexity, but may not be needed in fact. The key is to continuously monitor and analyze the performance of the application during the development process, and then optimize it in a targeted manner.
Overall, React's application in HTML brings great convenience and flexibility to modern web development. By understanding its core concepts and best practices, you can build efficient, maintainable, and user-friendly web applications. I hope this article can help you better grasp the power of React and use it flexibly in actual projects.
The above is the detailed content of The Power of React in HTML: Modern Web Development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
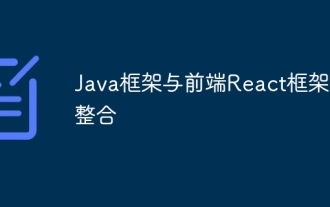
Integration of Java framework and React framework: Steps: Set up the back-end Java framework. Create project structure. Configure build tools. Create React applications. Write REST API endpoints. Configure the communication mechanism. Practical case (SpringBoot+React): Java code: Define RESTfulAPI controller. React code: Get and display the data returned by the API.
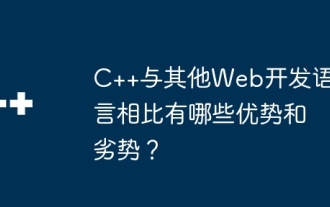
The advantages of C++ in web development include speed, performance, and low-level access, while limitations include a steep learning curve and memory management requirements. When choosing a web development language, developers should consider the advantages and limitations of C++ based on application needs.
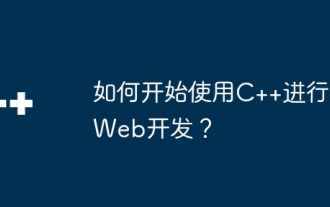
To use C++ for web development, you need to use frameworks that support C++ web application development, such as Boost.ASIO, Beast, and cpp-netlib. In the development environment, you need to install a C++ compiler, text editor or IDE, and web framework. Create a web server, for example using Boost.ASIO. Handle user requests, including parsing HTTP requests, generating responses, and sending them back to the client. HTTP requests can be parsed using the Beast library. Finally, a simple web application can be developed, such as using the cpp-netlib library to create a REST API, implementing endpoints that handle HTTP GET and POST requests, and using J

Vue.js is suitable for small and medium-sized projects and fast iterations, while React is suitable for large and complex applications. 1) Vue.js is easy to use and is suitable for situations where the team is insufficient or the project scale is small. 2) React has a richer ecosystem and is suitable for projects with high performance and complex functional needs.
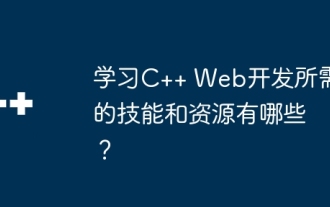
C++ Web development requires mastering the basics of C++ programming, network protocols, and database knowledge. Necessary resources include web frameworks such as cppcms and Pistache, database connectors such as cppdb and pqxx, and auxiliary tools such as CMake, g++, and Wireshark. By learning practical cases, such as creating a simple HTTP server, you can start your C++ Web development journey.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

HTML, CSS and JavaScript are the three pillars of web development. 1. HTML defines the web page structure and uses tags such as, etc. 2. CSS controls the web page style, using selectors and attributes such as color, font-size, etc. 3. JavaScript realizes dynamic effects and interaction, through event monitoring and DOM operations.

PHP remains important in modern web development, especially in content management and e-commerce platforms. 1) PHP has a rich ecosystem and strong framework support, such as Laravel and Symfony. 2) Performance optimization can be achieved through OPcache and Nginx. 3) PHP8.0 introduces JIT compiler to improve performance. 4) Cloud-native applications are deployed through Docker and Kubernetes to improve flexibility and scalability.
