HTML: The Structure, CSS: The Style, JavaScript: The Behavior
introduction
<p> In the world of web development, HTML, CSS and JavaScript have jointly built the modern website we see. They are like the three pillars of architecture: HTML provides structure, like the framework of a house; CSS is responsible for the style, making the house beautiful; JavaScript gives behavior, making the house smart. This article will take you into the depth of the functions and collaboration of these three, helping you understand how to use them to build a dynamic and beautiful website. You will learn a comprehensive range of knowledge from basic concepts to practical applications, and learn how to use these techniques efficiently in your project.Review of basic knowledge
<p> HTML (HyperText Markup Language) is the cornerstone of building web pages, which uses tag tags to describe the structure of a web page. Imagine HTML is like a woodworker that frames your web pages, and these tags are wood and nails. CSS (Cascading Style Sheets) is responsible for beautifying this framework, which defines the appearance and layout of a web page, including everything from colors, fonts to animation effects. JavaScript is the soul of web pages, which makes web pages no longer static images, but a dynamic entity that can interact with users.Core concept or function analysis
HTML: The Art of Building Structure
<p> The role of HTML is to define the structure and content of a web page. Through a series of tags, HTML can organize elements such as text, images, and links to form a logical structure. Its advantages are its simplicity and easy to learn, and good readability and maintainability.<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>My First Webpage</title> </head> <body> <h1 id="Welcome-to-My-Website">Welcome to My Website</h1> <p>This is a paragraph of text.</p> <img src="/static/imghw/default1.png" data-src="image.jpg" class="lazy" alt="An image"> <a href="https://www.example.com">Visit Example.com</a> </body> </html>
CSS: The color that gives life to web pages
<p> The function of CSS is to control the appearance and layout of a web page. It defines the style of elements through selectors and attributes, making the web page not only clear structure, but also beautiful and elegant. The advantage of CSS is that it can be independent of HTML content, separate styles and structures, and improves the maintainability and reusability of the code.body { font-family: Arial, sans-serif; background-color: #f0f0f0; } h1 { color: #333; text-align: center; } p { color: #666; line-height: 1.5; } img { max-width: 100%; height: auto; } a { color: #0066cc; text-decoration: none; } a:hover { text-decoration: underline; }
JavaScript: Magic to make web pages move
<p> The role of JavaScript is to add dynamic behavior to web pages. It can respond to user actions, modify the DOM, process data, and even communicate with the server. The advantage of JavaScript is that it can make web pages more interactive and intelligent and improve user experience.document.addEventListener('DOMContentLoaded', function() { const button = document.querySelector('button'); button.addEventListener('click', function() { alert('Button clicked!'); }); const input = document.querySelector('input'); input.addEventListener('input', function() { console.log('Input value:', input.value); }); });
Example of usage
Basic usage of HTML
<p> The basic usage of HTML is to define the structure of a web page through tags. Here is a simple HTML page example:<!DOCTYPE html> <html lang="en"> <head> <meta charset="UTF-8"> <title>My First Webpage</title> </head> <body> <h1 id="Welcome-to-My-Website">Welcome to My Website</h1> <p>This is a paragraph of text.</p> <img src="/static/imghw/default1.png" data-src="image.jpg" class="lazy" alt="An image"> <a href="https://www.example.com">Visit Example.com</a> </body> </html>
<h1>
defines a title, <p>
defines a paragraph, <img alt="HTML: The Structure, CSS: The Style, JavaScript: The Behavior" >
inserts an image, and <a>
creates a link.Advanced usage of CSS
<p> Advanced usage of CSS includes using Flexbox or Grid to create complex layouts, and using animations and transition effects to enhance the user experience. Here is an example using Flexbox:.container { display: flex; justify-content: space-between; align-items: center; padding: 20px; } .item { flex: 1; margin: 10px; padding: 20px; background-color: #eee; border: 1px solid #ddd; transition: all 0.3s ease; } .item:hover { transform: scale(1.05); box-shadow: 0 0 10px rgba(0, 0, 0, 0.1); }
Common Errors and Debugging Tips for JavaScript
<p> Common errors in JavaScript development include syntax errors, type errors, and logical errors. Here are some common errors and their debugging tips:- Syntax error : Use the browser's developer tools to view the console output and find the specific location of the error.
- Type error : Use
typeof
operator to check the variable type to ensure that the operators and methods are used correctly. - Logical error : Use
console.log
ordebugger
statement to track the code execution process and find out the logical problem.
// Syntax error example function greet(name) { console.log('Hello, ' name!); // Error: There is an exclamation mark} // Type error example let number = '123'; // String type console.log(number 10); // Output '12310' instead of 133 // Logical error example function calculateTotal(price, taxRate) { let total = price taxRate; // Error: price * taxRate should be used return total; }
Performance optimization and best practices
<p> In practical applications, optimizing HTML, CSS and JavaScript code can significantly improve the performance and user experience of web pages. Here are some recommendations for optimization and best practices:- HTML optimization : Use semantic tags to reduce nesting levels and compress HTML code.
- CSS optimization : Use efficient selectors to avoid overuse!important, compress CSS code.
- JavaScript optimization : Reduce DOM operations, use event delegates, asynchronous loading of scripts, and compress JavaScript code.
// Unoptimized version function slowFunction() { let result = 0; for (let i = 0; i < 1000000; i ) { result = i; } return result; } // Optimized version function fastFunction() { return (1000000 * 999999) / 2; // Use mathematical formulas to calculate directly} console.time('slowFunction'); slowFunction(); console.timeEnd('slowFunction'); console.time('fastFunction'); fastFunction(); console.timeEnd('fastFunction');
The above is the detailed content of HTML: The Structure, CSS: The Style, JavaScript: The Behavior. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
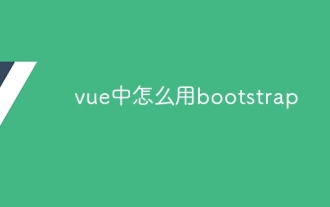
Using Bootstrap in Vue.js is divided into five steps: Install Bootstrap. Import Bootstrap in main.js. Use the Bootstrap component directly in the template. Optional: Custom style. Optional: Use plug-ins.

HTML defines the web structure, CSS is responsible for style and layout, and JavaScript gives dynamic interaction. The three perform their duties in web development and jointly build a colorful website.

React combines JSX and HTML to improve user experience. 1) JSX embeds HTML to make development more intuitive. 2) The virtual DOM mechanism optimizes performance and reduces DOM operations. 3) Component-based management UI to improve maintainability. 4) State management and event processing enhance interactivity.

WebdevelopmentreliesonHTML,CSS,andJavaScript:1)HTMLstructurescontent,2)CSSstylesit,and3)JavaScriptaddsinteractivity,formingthebasisofmodernwebexperiences.
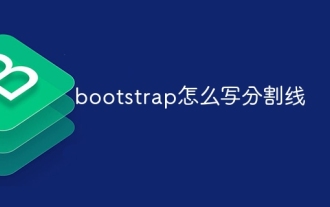
There are two ways to create a Bootstrap split line: using the tag, which creates a horizontal split line. Use the CSS border property to create custom style split lines.
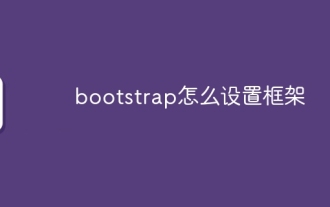
To set up the Bootstrap framework, you need to follow these steps: 1. Reference the Bootstrap file via CDN; 2. Download and host the file on your own server; 3. Include the Bootstrap file in HTML; 4. Compile Sass/Less as needed; 5. Import a custom file (optional). Once setup is complete, you can use Bootstrap's grid systems, components, and styles to create responsive websites and applications.
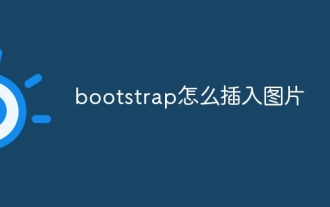
There are several ways to insert images in Bootstrap: insert images directly, using the HTML img tag. With the Bootstrap image component, you can provide responsive images and more styles. Set the image size, use the img-fluid class to make the image adaptable. Set the border, using the img-bordered class. Set the rounded corners and use the img-rounded class. Set the shadow, use the shadow class. Resize and position the image, using CSS style. Using the background image, use the background-image CSS property.
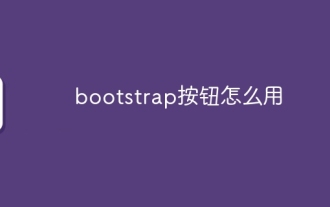
How to use the Bootstrap button? Introduce Bootstrap CSS to create button elements and add Bootstrap button class to add button text
