Laravel: A Framework for Modern Web Development
Laravel is a modern PHP-based framework that follows the MVC architecture pattern, provides rich tools and functions, and simplifies the web development process. 1) It contains Eloquent ORM for database interaction, 2) Artisan command line interface for fast code generation, 3) Blade template engine for efficient view development, 4) Powerful routing system for defining URL structures, 5) Authentication system for user management, 6) Event listening and broadcast for real-time functions, 7) Cache and queue systems for performance optimization, making it easier and more efficient to build and maintain modern web applications.
introduction
When I first came into contact with Laravel, I was deeply attracted by its elegance and efficiency. As a modern PHP framework, Laravel not only simplifies the web development process, but also provides developers with rich tools and features, making it easier to build powerful and maintainable applications. In this article, we will dive into the core features of Laravel, from basic knowledge to advanced applications, and let’s see why Laravel has become the first choice for many developers.
By reading this article, you will learn about the basic concepts of Laravel, how it works, and how to use it to build modern web applications. In addition, you will learn some practical tips and best practices to help you better use Laravel in real-world projects.
Review of basic knowledge
Laravel is a PHP-based framework that follows the MVC (Model-View-Controller) architecture pattern. The MVC pattern separates the application's logic, data and user interface, making the code more structured and maintainable. Laravel also introduces many convenient features, such as Eloquent ORM (Object Relational Mapping), which makes interacting with a database very simple.
In addition, Laravel also integrates many modern development tools, such as the Artisan command line interface, which can help developers quickly generate code and execute common tasks. The Blade template engine is another highlight, which provides powerful template functions, making the development of view layers more efficient.
Core concept or function analysis
The core functions and functions of Laravel
One of the core features of Laravel is its routing system, which allows developers to define the URL structure of an application and map these URLs to specific controller actions. This design makes the application structure clearer and easier to manage.
// Define a simple route Route::get('/home', function () { return 'Welcome to the home page!'; });
Another key feature is Eloquent ORM, which provides an elegant way to interact with the database. Through Eloquent, developers can use an object-oriented approach to manipulating database tables and records, which greatly simplifies the complexity of data operations.
// Create and query user using Eloquent ORM $user = new App\Models\User(); $user->name = 'John Doe'; $user->email = 'john@example.com'; $user->save(); <p>$users = App\Models\User::all();</p>
How Laravel works
How Laravel works can be understood from its request lifecycle. When a request arrives in a Laravel application, it is first processed by middleware and then routed to the corresponding controller action. In the controller, developers can call models to interact with the database, process business logic, and finally pass the data to the view for rendering.
// Request lifecycle example Route::get('/user/{id}', function ($id) { return view('user.profile', ['user' => App\Models\User::findOrFail($id)]); });
In terms of performance, Laravel adopts a caching mechanism to improve the response speed of applications. It also supports queueing systems, allowing time-consuming tasks to be executed asynchronously, thereby improving the user experience.
Example of usage
Basic usage
Let's start with a simple CRUD (create, read, update, delete) operation to show the basic usage of Laravel. We will create a simple blog system where users can create, view, edit and delete articles.
// Define the article model namespace App\Models; <p>use Illuminate\Database\Eloquent\Model;</p><p> class Post extends Model { protected $fillable = ['title', 'content']; }</p><p> // Define the controller namespace App\Http\Controllers;</p><p> use App\Models\Post; use Illuminate\Http\Request;</p><p> class PostController extends Controller { public function index() { $posts = Post::all(); return view('posts.index', compact('posts')); }</p><pre class='brush:php;toolbar:false;'> public function create() { return view('posts.create'); } public function store(Request $request) { $post = new Post(); $post->title = $request->input('title'); $post->content = $request->input('content'); $post->save(); return redirect('/posts'); } // Other CRUD methods...
}
Advanced Usage
In actual projects, we may encounter more complex needs, such as implementing user authentication and authorization. Laravel provides a powerful certification system that can be easily integrated into applications.
// Use Laravel's authentication system to use Illuminate\Support\Facades\Auth; <p>Route::get('/login', function () { return view('auth.login'); });</p><p> Route::post('/login', function (Request $request) { $credentials = $request->only(['email', 'password']); if (Auth::attempt($credentials)) { return redirect()->intended('/dashboard'); } return back()->withErrors(['email' => 'Invalid credentials']); });</p>
In addition, Laravel supports event monitoring and broadcasting, allowing developers to easily implement real-time features such as live chat or notification systems.
Common Errors and Debugging Tips
When using Laravel, developers may encounter some common errors, such as errors in migration files, errors in model relationships, etc. Here are some debugging tips:
- Use
php artisan migrate:status
command to view the migration status to ensure that all migration files are executed correctly. - Use the
dd()
function in the model to debug the data to see if the model loads the data correctly. - Use Laravel's logging system to record the operation of the application and help locate problems.
Performance optimization and best practices
In practical applications, performance optimization is crucial. Laravel provides a variety of ways to improve the performance of your application, such as using cache, optimizing database queries, etc.
// Use cache to optimize performance use Illuminate\Support\Facades\Cache; <p>Route::get('/posts', function () { return Cache::remember('posts', 3600, function () { return App\Models\Post::all(); }); });</p>
Following best practices when writing code can improve the readability and maintenance of your code. For example, use namespaces to organize your code, use comments to interpret complex logic, and use Eloquent's query builder to optimize database queries.
Overall, Laravel is a powerful and easy-to-use framework that provides a solid foundation for modern web development. By gaining insight into its core concepts and features, developers can better leverage Laravel to build efficient, maintainable web applications.
The above is the detailed content of Laravel: A Framework for Modern Web Development. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
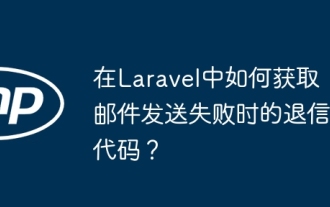
Method for obtaining the return code when Laravel email sending fails. When using Laravel to develop applications, you often encounter situations where you need to send verification codes. And in reality...
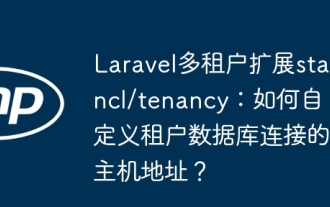
Custom tenant database connection in Laravel multi-tenant extension package stancl/tenancy When building multi-tenant applications using Laravel multi-tenant extension package stancl/tenancy,...
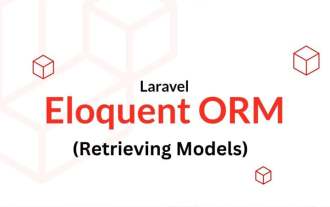
LaravelEloquent Model Retrieval: Easily obtaining database data EloquentORM provides a concise and easy-to-understand way to operate the database. This article will introduce various Eloquent model search techniques in detail to help you obtain data from the database efficiently. 1. Get all records. Use the all() method to get all records in the database table: useApp\Models\Post;$posts=Post::all(); This will return a collection. You can access data using foreach loop or other collection methods: foreach($postsas$post){echo$post->
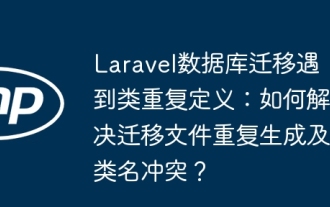
A problem of duplicate class definition during Laravel database migration occurs. When using the Laravel framework for database migration, developers may encounter "classes have been used...
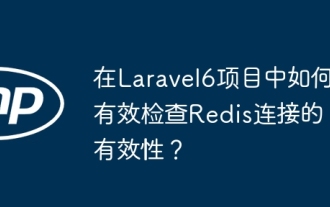
How to check the validity of Redis connections in Laravel6 projects is a common problem, especially when projects rely on Redis for business processing. The following is...
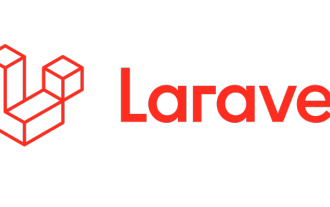
Efficiently process 7 million records and create interactive maps with geospatial technology. This article explores how to efficiently process over 7 million records using Laravel and MySQL and convert them into interactive map visualizations. Initial challenge project requirements: Extract valuable insights using 7 million records in MySQL database. Many people first consider programming languages, but ignore the database itself: Can it meet the needs? Is data migration or structural adjustment required? Can MySQL withstand such a large data load? Preliminary analysis: Key filters and properties need to be identified. After analysis, it was found that only a few attributes were related to the solution. We verified the feasibility of the filter and set some restrictions to optimize the search. Map search based on city

How does Laravel play a role in backend logic? It simplifies and enhances backend development through routing systems, EloquentORM, authentication and authorization, event and listeners, and performance optimization. 1. The routing system allows the definition of URL structure and request processing logic. 2.EloquentORM simplifies database interaction. 3. The authentication and authorization system is convenient for user management. 4. The event and listener implement loosely coupled code structure. 5. Performance optimization improves application efficiency through caching and queueing.
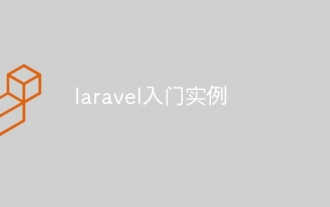
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
