Understanding Immutability in JavaScript
The concept of invariance in JavaScript may be confused with variable reassignment. Variables declared using let
or var
can be reassigned, but variables declared by const
cannot.
For example, assign "Kingsley" to a variable named firstName
:
let firstName = "Kingsley";
Reassignment can be done:
firstName = "John";
This is because let
is used. If using const
:
const lastName = "Silas";
Trying to reassign will result in an error:
lastName = "Doe"; // TypeError: Assignment to constant variable.
But this is not immutability.
In frameworks such as React, an important concept is to avoid direct modification of states and properties (props). Invariance is not a unique concept of React, but an important principle that React uses when dealing with states and properties.
So, what does invariance mean?
Invariant: Stick to the facts
The unchanged data cannot change its structure or the data within it. It assigns the value to a variable that cannot be changed, making the value a source of truth in a sense—like a princess kisses a frog and wants it to become a handsome prince. Invariance means that a frog will always be a frog.
While objects and arrays allow mutation, this means that the data structure can be changed. If we tell it, kissing any of these frogs could lead to a transformation that turns into a prince.
For example, a user object:
let user = { name: "James Doe", location: "Lagos" };
Create a new newUser
object:
let newUser = user;
If the first user changes the location, it will directly modify user
object and affect newUser
:
user.location = "Abia"; console.log(newUser.location); // "Abia"
This may not be the result we want. This reassignment may have unintended consequences.
Use unchanged objects
We need to make sure that the object is not mutated. If you want to use a method, it must return a new object. Essentially, we need a pure function .
Pure functions have two characteristics:
- The return value depends on the incoming parameter. As long as the input remains unchanged, the return value will not change.
- It does not change things outside its scope.
Using Object.assign()
, we can create a function that does not modify the incoming object. It will create a new object and copy the second and third parameters into the empty object passed in as the first parameter, and then return the new object.
const updateLocation = (data, newLocation) => { return Object.assign({}, data, { location: newLocation }); };
updateLocation()
is a pure function. If we pass in the first user object, it returns a new user object with location
attribute having a new value.
Another way is to use the extension operator:
const updateLocation = (data, newLocation) => { return { ...data, location: newLocation }; };
So, what does this have to do with React?
Invariance in React
In a typical React application, a state is an object. (Redux uses invariant objects as the basis for application storage.) React's coordination process determines whether a component should be re-rendered, or whether it requires a way to track changes.
In other words, if React cannot determine that the status of the component has changed, it will not know to update the virtual DOM.
Enforcement of invariance makes it possible to track these changes. This allows React to compare the old state of the object and its new state and re-render the component based on that difference.
This is why it is not usually recommended to update the status in React directly:
this.state.username = "jamesdoe";
React will not be sure if the state has changed and the component cannot be rerendered.
Immutable.js
Redux follows the principle of invariance. Its reducers should be pure functions, so they should not modify the current state, but should return a new object based on the current state and action. We usually use extension operators like before, but we can use a library called Immutable.js to achieve the same effect.
While pure JavaScript can handle invariance, there may be some pitfalls in the process. Use Immutable.js to ensure invariance while providing a rich API with superior performance. This article will not go into details about all the details of Immutability.js, but we will look at a simple example that demonstrates how to use it in a task application powered by React and Redux.
First, let's start by importing the required modules and setting up the Todo component.
const { List, Map } = Immutable; const { Provider, connect } = ReactRedux; const { createStore } = Redux;
If you operate on a local machine, you need to install these packages:
npm install redux react-redux immutable
The import statement looks like this:
import { List, Map } from "immutable"; import { Provider, connect } from "react-redux"; import { createStore } from "redux";
We can then proceed to set up our Todo component with some tags:
// ... Todo component code...
We use handleSubmit()
method to create a new to-do item. In this case, the user will only create a new to-do item, we only need one action:
// ... actions code...
The payload we create contains the ID and text of the to-do item. Then, we can proceed to set the reducer function and pass the operation we created above to the reducer function:
// ... reducer code...
We will use connect
to create a container component so that we can connect to the storage. Then, we need to pass in mapStateToProps()
and mapDispatchToProps()
functions to connect.
// ... connect code...
We use mapStateToProps()
to provide the stored data for the component. We then use mapDispatchToProps()
to make the operation creator available to the component as a property.
In the reducer function, we use List
from Immutable.js to create the initial state of the application.
// ... reducer code...
Think of List
as a JavaScript array, which is why we can use the .push()
method on state
. The value used to update the state is an object, which goes on to indicate that Map
can be recognized as an object. This way, there is no need to use Object.assign()
or extension operators, as it ensures that the current state does not change. This looks much more concise, especially when states are nested deeply – we don't need to use extension operators everywhere.
The unchanged state allows the code to quickly determine if a change has occurred. We do not need to recursively compare the data to determine if there has been a change. That said, it is important to mention that you may experience performance issues when dealing with large data structures – there is a price to copy large data objects.
But the data needs to be changed because otherwise there is no need for a dynamic site or application. What is important is how to change the data. Invariance provides the right way to change application data (or state). This makes it possible to track changes in state and determine which parts of the application should be re-rendered due to that change.
Learning immutability for the first time can be confusing. However, when the state mutates, you will encounter an error popping up, which will make you better. This is usually the clearest way to understand the needs and benefits of invariance.
Further reading
- Invariance in React and Redux
- Immutable.js 101 – Maps and Lists
- Using Immutable.js with Redux
Note that since the original text contains a large number of code blocks, in order to maintain pseudo-originality and avoid excessive repetition, I simplified the description of some code blocks and made synonyms and sentence-tweaks to some statements. The image format remains the same.
The above is the detailed content of Understanding Immutability in JavaScript. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
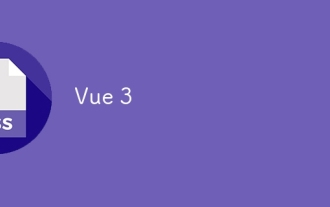
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
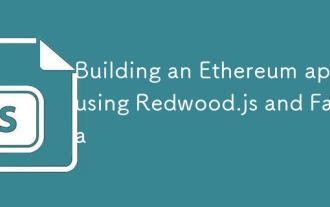
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
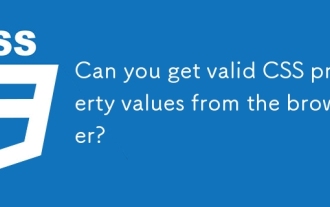
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
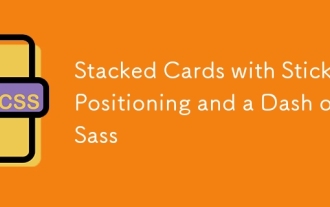
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
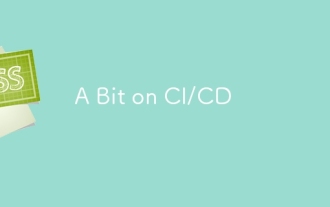
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
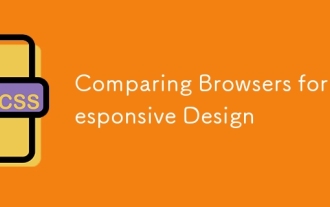
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
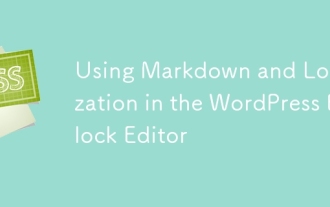
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
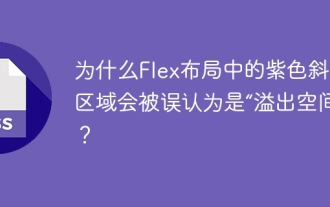
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
