Can mysql database store images?
Storing images in a MySQL database is feasible, but not best practice. MySQL uses BLOB type when storing images, but it can cause database volume swell, query speed and complex backups. A better solution is to store images on a file system and store only image paths in the database to optimize query performance and database volume.
Can images be stored in MySQL database? The answer is yes, but...
You ask if you can store images in the MySQL database? sure! But this is like asking if you can use a screwdriver to screw nails. Although it can be done, it may not be the best solution. I stuffed pictures directly into the database, which sounded simple and crude, but in actual operation, I had a secret. If I was not careful, I would fall into the pit.
Let's review the basics first. MySQL itself does not process image data directly, it processes binary data. Image files, whether they are JPG, PNG, or GIF, are essentially a combination of a series of bytes. So, what we store is actually a binary representation of the image file. Usually, we use BLOB
or MEDIUMBLOB
, LONGBLOB
and other data types to store these binary data. The size of the BLOB
family is incremented in turn, and which one is selected depends on your image size. Remember, a larger BLOB
type means greater storage space usage and will also affect query efficiency.
So, how does BLOB
work? Simply put, it is like a huge byte container, stuffing the entire image file into it. When querying, the database will read out the entire BLOB
data in one go and then hand it over to the application for decoding and displaying. It's like you stuff a whole encyclopedia into an envelope and send it out. Although it can be received, it is absolutely not efficient.
Let’s take a look at a simple example, suppose you use Python and MySQLdb libraries:
<code class="python">import mysql.connector from PIL import Image mydb = mysql.connector.connect( host="localhost", user="yourusername", password="yourpassword", database="mydatabase" ) mycursor = mydb.cursor() # 打开图像文件img = Image.open("myimage.jpg") img_bytes = img.tobytes() # 将图像数据插入数据库sql = "INSERT INTO images (image) VALUES (%s)" val = (img_bytes,) mycursor.execute(sql, val) mydb.commit() # 获取图像数据mycursor.execute("SELECT image FROM images WHERE id = 1") result = mycursor.fetchone() img_data = result[0] # 将二进制数据转换为图像img = Image.frombytes(img.mode, img.size, img_data) img.save("retrieved_image.jpg") mycursor.close() mydb.close()</code>
This code shows the basic storage and reading process. But, note that this is just the simplest example. In practical applications, you may need to handle exceptions, optimize database connections, and even consider transaction processing.
Now, let's explore the advantages and disadvantages of this solution and the potential pitfalls.
Advantages: Simple and direct, easy to manage images and other database data.
Disadvantages: The database volume swells, the query speed is as slow as a snail, and backup and recovery also become extremely painful. Just imagine your database is filled with thousands of HD pictures, and the time cost of backup and recovery is simply disastrous. Not to mention, the I/O pressure on database servers will also increase sharply.
What is a better solution? Usually, we choose to store the image on the file system and then store only the path to the image file in the database. In this way, the database only stores a small amount of text data, the query speed is greatly improved, and the database volume is effectively controlled. Of course, this requires you to handle file system management extra, but in the long run, it's a smarter choice. You can even consider using object storage services such as AWS S3 or Alibaba Cloud OSS to further improve scalability and performance.
In short, storing images in MySQL is not unfeasible, but it is usually not a best practice. Weigh the pros and cons and choose a solution that suits your application scenario is the best way to do it. Don’t be confused by the simplicity on the surface. Only by thinking deeply can you avoid falling into those headache-prone pits.
The above is the detailed content of Can mysql database store images?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
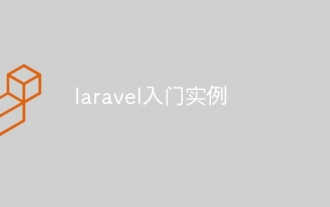
Laravel is a PHP framework for easy building of web applications. It provides a range of powerful features including: Installation: Install the Laravel CLI globally with Composer and create applications in the project directory. Routing: Define the relationship between the URL and the handler in routes/web.php. View: Create a view in resources/views to render the application's interface. Database Integration: Provides out-of-the-box integration with databases such as MySQL and uses migration to create and modify tables. Model and Controller: The model represents the database entity and the controller processes HTTP requests.
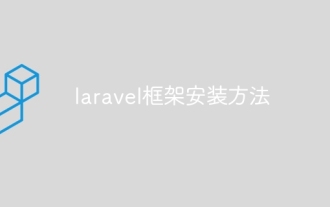
Article summary: This article provides detailed step-by-step instructions to guide readers on how to easily install the Laravel framework. Laravel is a powerful PHP framework that speeds up the development process of web applications. This tutorial covers the installation process from system requirements to configuring databases and setting up routing. By following these steps, readers can quickly and efficiently lay a solid foundation for their Laravel project.

MySQL and phpMyAdmin are powerful database management tools. 1) MySQL is used to create databases and tables, and to execute DML and SQL queries. 2) phpMyAdmin provides an intuitive interface for database management, table structure management, data operations and user permission management.
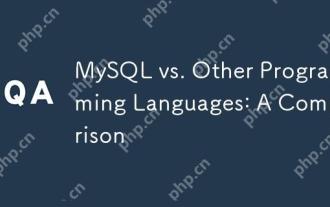
Compared with other programming languages, MySQL is mainly used to store and manage data, while other languages such as Python, Java, and C are used for logical processing and application development. MySQL is known for its high performance, scalability and cross-platform support, suitable for data management needs, while other languages have advantages in their respective fields such as data analytics, enterprise applications, and system programming.
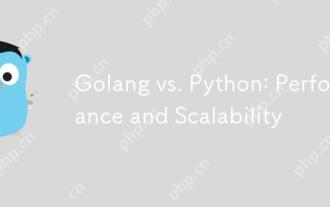
Golang is better than Python in terms of performance and scalability. 1) Golang's compilation-type characteristics and efficient concurrency model make it perform well in high concurrency scenarios. 2) Python, as an interpreted language, executes slowly, but can optimize performance through tools such as Cython.
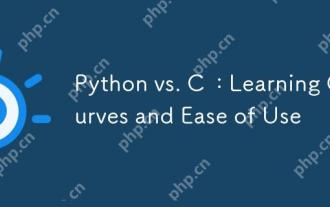
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
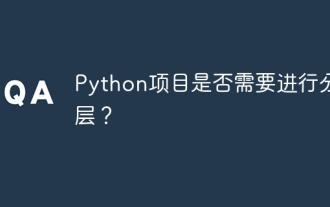
Discussion on Hierarchical Structure in Python Projects In the process of learning Python, many beginners will come into contact with some open source projects, especially projects using the Django framework...
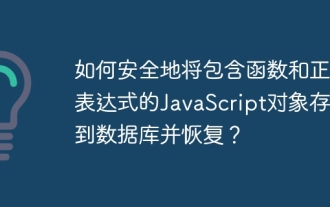
Safely handle functions and regular expressions in JSON In front-end development, JavaScript is often required...
