Using Formik to Handle Forms in React
Web forms are essential for websites and applications. While native HTML forms offer basic functionality, managing their state, validation, and submission can become complex. React, with its component-driven approach, simplifies form handling but can still lead to verbose code. Formik, a lightweight library, elegantly addresses these challenges, simplifying state management, validation, and submission.
This article demonstrates building a React form with Formik, showcasing its capabilities for handling state, validation, and submission. We'll start with a basic React component and then integrate Formik.
Building a Controlled React Form
React components rely on state and props. HTML form elements maintain internal state through their value
attribute. This makes them uncontrolled components—the DOM manages the state. However, controlled components, where React manages the state, offer better control and a single source of truth.
A simple uncontrolled HTML form might look like this:
<div class="formRow"> <label for="email">Email address</label> <input type="email" id="email"> </div> <div class="formRow"> <label for="password">Password</label> <input type="password" id="password"> </div> <button type="submit">Submit</button>
Converting this to a controlled React component:
function HTMLForm() { const [email, setEmail] = React.useState(""); const [password, setPassword] = React.useState(""); return (
This approach provides a single source of truth for form values, enabling easier validation and performance optimization.
Why Choose Formik?
Numerous form management libraries exist for React (e.g., React Hook Form, Redux Form). Formik distinguishes itself through:
- Declarative Approach: Abstraction simplifies state, validation, and submission.
- Escape Hatch: Provides control when needed, despite its abstraction.
- Co-located State: Keeps form-related logic within the component.
- Adaptability: Allows using as much or as little of Formik as required.
- Ease of Use: Simple and effective.
Let's integrate Formik into our form component.
Implementing Formik
We'll create a basic login form, exploring three Formik usage methods:
-
useFormik
hook - Formik with React context (
<formik></formik>
) -
withFormik
higher-order component
A demo requires Formik and Yup packages.
Method 1: useFormik
Hook
Our current form is non-functional. The useFormik
hook provides Formik functions and variables. Logging the returned value reveals its contents.
We'll use useFormik
with initialValues
and an onSubmit
handler:
// React component function BaseFormik() { const formik = useFormik({ initialValues: { email: "", password: "" }, onSubmit(values) { // Form submission logic } }); return (
Binding Formik to form elements:
// React component function BaseFormik() { const formik = useFormik({ initialValues: { email: "", password: "" }, onSubmit(values) { // Form submission logic } }); return (
This handles submission (onSubmit={formik.handleSubmit}
) and input state (value
, onChange
via formik.getFieldProps
). We avoid manual state management and event handling. Destructuring getFieldProps
and handleSubmit
further improves code clarity.
The <formik></formik>
component offers further abstraction with built-in components like <field></field>
, <form></form>
, and <errormessage></errormessage>
. These components utilize the render props pattern.
import { Formik, Field, Form } from 'formik'; function FormikRenderProps() { const initialValues = { email: "", password: "" }; const onSubmit = (values) => { alert(JSON.stringify(values, null, 2)); }; return ( <formik initialvalues="{initialValues}" onsubmit="{onSubmit}"> {() => ( <form> <field classname="email formField" name="email" type="email"></field> {/* ... other form elements */} </form> )} </formik> ); }
Formik handles state and submission; we focus on form structure.
Form Validation with Formik
Formik offers validation at the form level (using validate
or validationSchema
), field level, or with manual triggers. Form-level validation validates the entire form at once. validate
is for custom validation, while validationSchema
uses a library like Yup.
Example using validate
:
const formik = useFormik({ // ... validate: () => { const errors = {}; if (!formik.values.email) { errors.email = "Required"; } // ... other validations return errors; }, // ... });
Example using validationSchema
with Yup:
const formik = useFormik({ // ... validationSchema: Yup.object().shape({ email: Yup.string().email("Invalid email").required("Required"), // ... other validations }), // ... });
Field-level and manual validation are also possible.
Method 3: withFormik
Higher-Order Component
withFormik
is a higher-order component that wraps your form component.
Practical Examples
Let's illustrate displaying error messages and generating a username.
Displaying Error Messages
Formik simplifies error display. Check the errors
object and display messages:
<label htmlfor="email"> Email address {formik.touched.email && formik.errors.email} </label>
Or use <errormessage></errormessage>
:
<errormessage name="email"></errormessage>
Generating a Username
Formik's setValues
can be used to generate a username from the email:
onSubmit(values) { formik.setValues({ ...values, username: `@${values.email.split("@")[0]}` }); }
Conclusion
Formik significantly simplifies React form handling. This article covers the basics; explore Formik's documentation for advanced features and use cases.
The above is the detailed content of Using Formik to Handle Forms in React. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
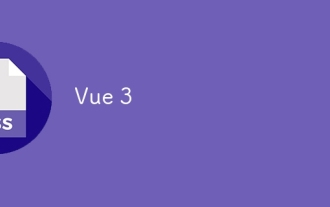
It's out! Congrats to the Vue team for getting it done, I know it was a massive effort and a long time coming. All new docs, as well.
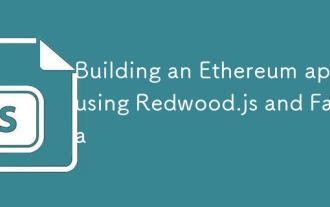
With the recent climb of Bitcoin’s price over 20k $USD, and to it recently breaking 30k, I thought it’s worth taking a deep dive back into creating Ethereum
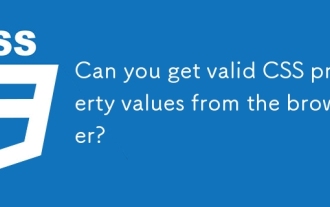
I had someone write in with this very legit question. Lea just blogged about how you can get valid CSS properties themselves from the browser. That's like this.
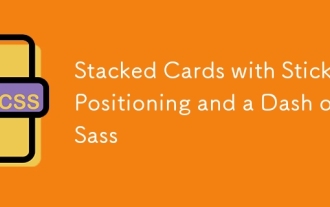
The other day, I spotted this particularly lovely bit from Corey Ginnivan’s website where a collection of cards stack on top of one another as you scroll.
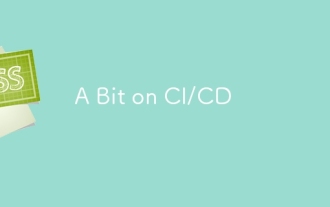
I'd say "website" fits better than "mobile app" but I like this framing from Max Lynch:
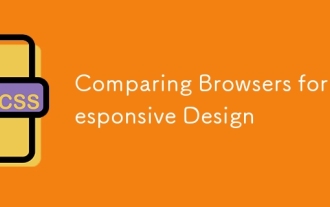
There are a number of these desktop apps where the goal is showing your site at different dimensions all at the same time. So you can, for example, be writing
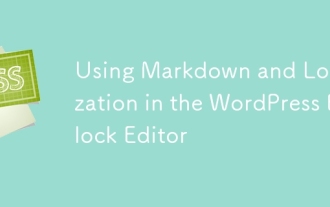
If we need to show documentation to the user directly in the WordPress editor, what is the best way to do it?
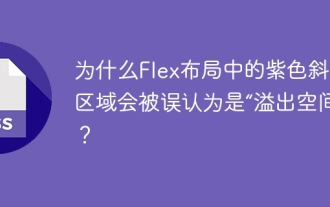
Questions about purple slash areas in Flex layouts When using Flex layouts, you may encounter some confusing phenomena, such as in the developer tools (d...
