


Is there any difference between NULL in C and NULL in other languages?
NULL in C is essentially a macro, which represents a null pointer, pointing to an invalid memory address; while null values in other languages (such as Python and Java) are represented by special keywords or objects. When using NULL, you need to be careful not to replace 0 directly, be careful about pointer calculation, conduct null pointer checking, and pay attention to the processing method when interacting across languages. Best practices include clear code, strict error handling and a good programming style.
NULL in C and NULL in other languages: Detailed explanation of zero pointers
Many beginners, even some veterans, think that NULL is NULL, and there is only one NULL in the world. But in fact, it's like saying that all cats are the same - on the surface, but when you look at it carefully, the difference will be huge. Although the C language NULL is the same as other languages, the implementation and behavior behind it may be very different.
This article will explore this seemingly simple "NULL" that hides mystery in depth. We will start from the perspective of C language to see what its NULL is, and then compare it with the "NULL" of other languages (such as Python and Java), and finally talk about some easy pitfalls and best practices.
NULL in C: A magical macro
In C language, NULL is not a built-in type, but a macro. Its definition is usually found in the <stddef.h></stddef.h>
header file, generally like this:
<code class="c">#ifdef __cplusplus #define NULL 0 #else #define NULL ((void *)0) #endif</code>
You may be a little confused when you see this. Why do you need to define this? This starts with the pointer in C language.
A pointer to C language is essentially a memory address. NULL
means a null pointer, that is, it does not point to any valid memory address. ((void *)0)
This definition method ensures that NULL
can be converted into any type pointer without the problem of type mismatch. The #ifdef __cplusplus
part is dealing with C compatibility, because C handles NULL slightly differently.
"NULL" in other languages: each has its own advantages
Other languages have their own style of processing null values.
- Python: Python uses
None
to represent a null value, which is a singleton object, not a macro. You can useis
operator to determine whether a variable isNone
, which is safer and more Pythonic than pointers in C language. - Java: Java uses
null
to represent null values, which is also a special keyword that means that the reference variable does not point to any object. Java'snull
is conceptually similar to C'sNULL
, but Java's type system is more stringent, avoiding some potential pointer errors in C.
Trap and pit avoidance guide
By understanding the nature of C language NULL, we can better avoid some common mistakes.
- Don't just use 0 instead of NULL: Although
0
andNULL
are interchangeable in many cases, this is a bad programming habit. UsingNULL
is clearer and easier to maintain. - Be careful of pointer operation:
NULL
pointers cannot be dereferenced (for example*NULL
), otherwise the program will crash. The compiler usually does not report an error because it is a runtime error. - Null pointer check: Before using a pointer, be sure to check whether it is
NULL
to avoid accessing illegal memory. - Cross-language interaction: When interacting with other languages in C, pay special attention to how NULL is handled. NULLs in different languages may differ in the underlying representation and require type conversion or other processing.
Performance and best practices
From a performance point of view, NULL
is very efficient because it is just a macro replacement. Best practice is:
- Clear code: Use
NULL
instead of0
to make the code easier to read and understand. - Strict error handling: Check all pointers that may be
NULL
to prevent program crashes. - Good programming style: Follow consistent naming specifications and code styles to improve the maintainability of the code.
In short, although NULL in C language looks similar to “NULL” in other languages, there are slight differences in their underlying implementation and usage. Only by understanding these differences can you write more robust and safer code. Remember, the essence of programming lies in details, and details often determine success or failure.
The above is the detailed content of Is there any difference between NULL in C and NULL in other languages?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
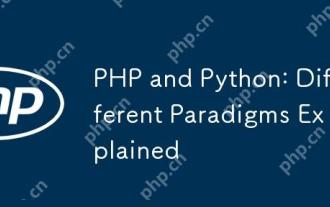
PHP is mainly procedural programming, but also supports object-oriented programming (OOP); Python supports a variety of paradigms, including OOP, functional and procedural programming. PHP is suitable for web development, and Python is suitable for a variety of applications such as data analysis and machine learning.
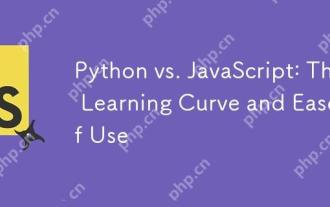
Python is more suitable for beginners, with a smooth learning curve and concise syntax; JavaScript is suitable for front-end development, with a steep learning curve and flexible syntax. 1. Python syntax is intuitive and suitable for data science and back-end development. 2. JavaScript is flexible and widely used in front-end and server-side programming.
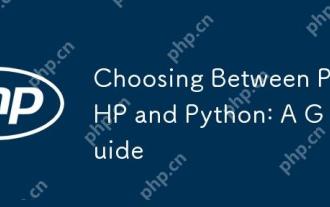
PHP is suitable for web development and rapid prototyping, and Python is suitable for data science and machine learning. 1.PHP is used for dynamic web development, with simple syntax and suitable for rapid development. 2. Python has concise syntax, is suitable for multiple fields, and has a strong library ecosystem.
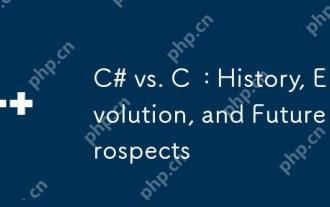
The history and evolution of C# and C are unique, and the future prospects are also different. 1.C was invented by BjarneStroustrup in 1983 to introduce object-oriented programming into the C language. Its evolution process includes multiple standardizations, such as C 11 introducing auto keywords and lambda expressions, C 20 introducing concepts and coroutines, and will focus on performance and system-level programming in the future. 2.C# was released by Microsoft in 2000. Combining the advantages of C and Java, its evolution focuses on simplicity and productivity. For example, C#2.0 introduced generics and C#5.0 introduced asynchronous programming, which will focus on developers' productivity and cloud computing in the future.
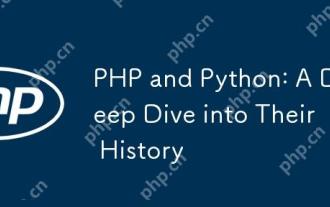
PHP originated in 1994 and was developed by RasmusLerdorf. It was originally used to track website visitors and gradually evolved into a server-side scripting language and was widely used in web development. Python was developed by Guidovan Rossum in the late 1980s and was first released in 1991. It emphasizes code readability and simplicity, and is suitable for scientific computing, data analysis and other fields.
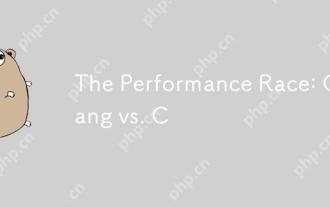
Golang and C each have their own advantages in performance competitions: 1) Golang is suitable for high concurrency and rapid development, and 2) C provides higher performance and fine-grained control. The selection should be based on project requirements and team technology stack.
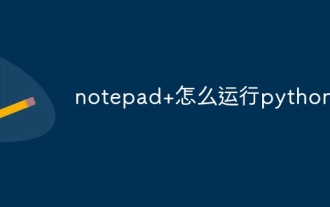
Running Python code in Notepad requires the Python executable and NppExec plug-in to be installed. After installing Python and adding PATH to it, configure the command "python" and the parameter "{CURRENT_DIRECTORY}{FILE_NAME}" in the NppExec plug-in to run Python code in Notepad through the shortcut key "F6".
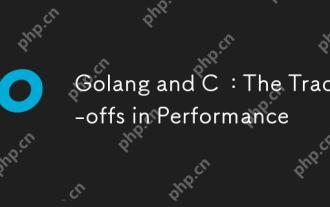
The performance differences between Golang and C are mainly reflected in memory management, compilation optimization and runtime efficiency. 1) Golang's garbage collection mechanism is convenient but may affect performance, 2) C's manual memory management and compiler optimization are more efficient in recursive computing.
