PHP Code Optimization Techniques: Improving code efficiency.
PHP Code Optimization Techniques: Improving code efficiency
PHP code optimization is crucial for improving the performance and efficiency of web applications. Here are several techniques that can help in optimizing PHP code:
- Use Opcode Caching: Implement opcode caching with tools like OPcache, which stores precompiled script bytecode in memory, reducing the need to compile PHP scripts repeatedly.
- Efficient Database Queries: Optimize database queries by using indexes, avoiding unnecessary SELECTs, and ensuring that queries are as specific as possible to reduce execution time.
- Minimize HTTP Requests: Reduce the number of HTTP requests by combining files (CSS, JavaScript) and using techniques like CSS sprites for images.
- Lazy Loading: Implement lazy loading for images and content that is not immediately needed upon page load to reduce initial load times.
- Code Profiling and Optimization: Use profiling tools to identify slow parts of the code and optimize them. This could involve refactoring code to be more efficient or eliminating redundant operations.
- Use of Latest PHP Versions: Newer versions of PHP come with performance improvements and optimizations. Upgrading to the latest stable version can significantly enhance performance.
- Avoid Using Globals: Global variables can slow down the execution as they must be processed by PHP for every function call. Using local variables or passing variables as parameters is generally faster.
- Implement Caching Mechanisms: Use caching mechanisms like Memcached or Redis to store and retrieve data quickly, reducing the load on the database and PHP processor.
-
Optimize Loops: Ensure loops are as efficient as possible. Avoid unnecessary operations inside loops and consider using more efficient loop constructs like
foreach
instead offor
when appropriate. - Use Built-in Functions and Extensions: PHP's built-in functions and extensions are often optimized and faster than custom implementations. Use them whenever possible.
By applying these techniques, developers can significantly improve the efficiency of their PHP code, leading to faster and more responsive applications.
What are some effective strategies for reducing PHP script execution time?
Reducing PHP script execution time is essential for improving the user experience and server performance. Here are some effective strategies:
- Optimize Database Interactions: Ensure that database queries are optimized. Use indexes, limit the data retrieved, and avoid unnecessary queries. Consider using database query caching to speed up repeated queries.
- Implement Caching: Use caching mechanisms like OPcache for PHP opcode caching, and Memcached or Redis for data caching. This reduces the need to repeatedly process the same data or code.
- Minimize External HTTP Requests: Reduce the number of external HTTP requests by combining files, using CSS sprites, and implementing lazy loading for images and content.
- Use Asynchronous Processing: For tasks that do not need to be completed immediately, use asynchronous processing to offload work from the main execution thread.
- Optimize PHP Code: Refactor code to eliminate redundant operations, use efficient loop constructs, and leverage PHP's built-in functions and extensions which are often optimized for performance.
- Upgrade PHP Version: Newer versions of PHP often include performance enhancements. Upgrading to the latest stable version can reduce execution times.
- Use Content Delivery Networks (CDNs): For static content, use CDNs to serve files from servers closer to the user, reducing load times.
- Enable Gzip Compression: Compress your output using Gzip to reduce the amount of data transferred over the network, which can speed up page load times.
By implementing these strategies, you can significantly reduce the execution time of PHP scripts, leading to faster and more efficient web applications.
How can I minimize memory usage in my PHP applications?
Minimizing memory usage in PHP applications is important for improving performance and scalability. Here are some strategies to achieve this:
-
Use Unset() Function: After variables are no longer needed, use the
unset()
function to free up memory. This is particularly useful in loops or when dealing with large datasets. - Optimize Data Structures: Choose the right data structures for your needs. For example, use arrays instead of objects when possible, as arrays are generally more memory-efficient.
-
Limit Data Retrieval: When querying databases, retrieve only the data you need. Use
LIMIT
clauses and select specific columns instead of usingSELECT *
. - Implement Caching: Use caching mechanisms like Memcached or Redis to store data in memory, reducing the need to repeatedly load data from databases or files.
- Avoid Deep Nesting: Deeply nested arrays or objects can consume more memory. Flatten data structures where possible to reduce memory usage.
-
Use References: When passing large objects or arrays to functions, use references (
&
) to avoid copying data, which can save memory. -
Optimize PHP Configuration: Adjust PHP settings like
memory_limit
andmax_execution_time
to better suit your application's needs, but be cautious not to set them too low. - Use Streaming: For large files or datasets, use streaming techniques to process data in chunks rather than loading everything into memory at once.
- Profile Memory Usage: Use tools like Xdebug or Blackfire to profile your application and identify memory-intensive parts of your code, then optimize those areas.
By applying these techniques, you can effectively minimize memory usage in your PHP applications, leading to more efficient and scalable solutions.
What tools can help me identify performance bottlenecks in PHP code?
Identifying performance bottlenecks in PHP code is crucial for optimization. Here are some tools that can help:
- Xdebug: Xdebug is a powerful PHP extension that provides stack traces, code coverage analysis, and profiling capabilities. It can help you identify slow parts of your code by generating detailed performance reports.
- Blackfire: Blackfire is a comprehensive profiling tool that provides deep insights into PHP application performance. It offers real-time profiling, automated recommendations, and can help identify bottlenecks in both PHP code and database queries.
- New Relic: New Relic is an application performance monitoring tool that provides detailed metrics on PHP application performance. It can help identify slow transactions, database queries, and other performance bottlenecks.
- Tideways: Tideways is a PHP profiler that offers detailed performance analysis and can help identify bottlenecks in your code. It provides insights into CPU and memory usage, helping you optimize your application.
- PHPStorm Profiler: PHPStorm, an integrated development environment (IDE), includes a built-in profiler that can help you identify performance issues directly within your development environment.
- Webgrind: Webgrind is a web-based Xdebug profiling viewer that allows you to analyze profiling data generated by Xdebug. It provides a user-friendly interface to explore performance bottlenecks.
- Zend Server: Zend Server includes a code tracing and profiling tool that can help identify performance issues in PHP applications. It provides detailed reports on execution time and resource usage.
- DebugBar: DebugBar is a PHP library that adds a debug toolbar to your application, providing real-time insights into performance metrics, database queries, and more.
By using these tools, you can effectively identify and address performance bottlenecks in your PHP code, leading to more efficient and responsive applications.
The above is the detailed content of PHP Code Optimization Techniques: Improving code efficiency.. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
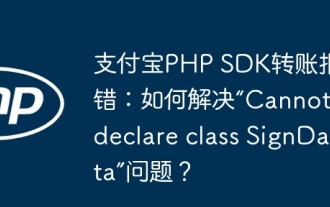
Alipay PHP...
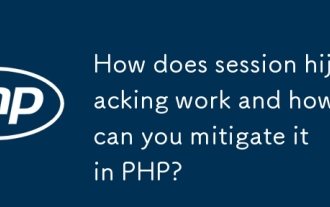
Session hijacking can be achieved through the following steps: 1. Obtain the session ID, 2. Use the session ID, 3. Keep the session active. The methods to prevent session hijacking in PHP include: 1. Use the session_regenerate_id() function to regenerate the session ID, 2. Store session data through the database, 3. Ensure that all session data is transmitted through HTTPS.
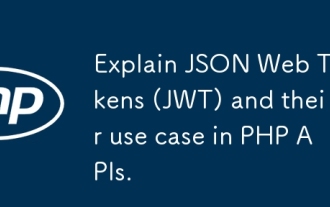
JWT is an open standard based on JSON, used to securely transmit information between parties, mainly for identity authentication and information exchange. 1. JWT consists of three parts: Header, Payload and Signature. 2. The working principle of JWT includes three steps: generating JWT, verifying JWT and parsing Payload. 3. When using JWT for authentication in PHP, JWT can be generated and verified, and user role and permission information can be included in advanced usage. 4. Common errors include signature verification failure, token expiration, and payload oversized. Debugging skills include using debugging tools and logging. 5. Performance optimization and best practices include using appropriate signature algorithms, setting validity periods reasonably,
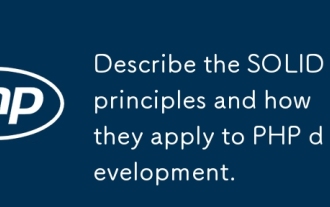
The application of SOLID principle in PHP development includes: 1. Single responsibility principle (SRP): Each class is responsible for only one function. 2. Open and close principle (OCP): Changes are achieved through extension rather than modification. 3. Lisch's Substitution Principle (LSP): Subclasses can replace base classes without affecting program accuracy. 4. Interface isolation principle (ISP): Use fine-grained interfaces to avoid dependencies and unused methods. 5. Dependency inversion principle (DIP): High and low-level modules rely on abstraction and are implemented through dependency injection.
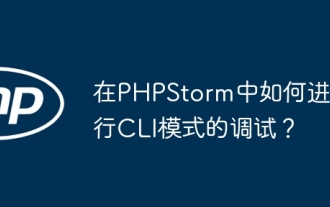
How to debug CLI mode in PHPStorm? When developing with PHPStorm, sometimes we need to debug PHP in command line interface (CLI) mode...
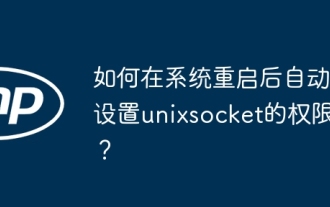
How to automatically set the permissions of unixsocket after the system restarts. Every time the system restarts, we need to execute the following command to modify the permissions of unixsocket: sudo...
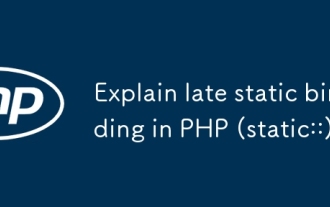
Static binding (static::) implements late static binding (LSB) in PHP, allowing calling classes to be referenced in static contexts rather than defining classes. 1) The parsing process is performed at runtime, 2) Look up the call class in the inheritance relationship, 3) It may bring performance overhead.
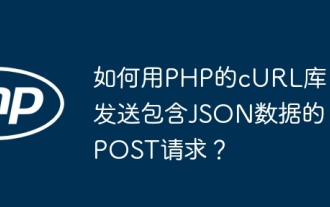
Sending JSON data using PHP's cURL library In PHP development, it is often necessary to interact with external APIs. One of the common ways is to use cURL library to send POST�...
