


How can you use Go's built-in benchmarking tools to measure performance improvements?
How can you use Go's built-in benchmarking tools to measure performance improvements?
Go provides built-in benchmarking tools through its testing
package, which allows developers to measure and compare the performance of their code over time. To use these tools, you first need to write benchmark functions. These functions are defined similarly to test functions but start with the prefix Benchmark
instead of Test
. Here's a step-by-step guide on how to use these tools:
-
Write a Benchmark Function: Create a function that starts with
Benchmark
followed by a descriptive name. The function should accept a*testing.B
argument and call a function or code snippet you want to benchmark inside a loop that runsb.N
times. For example:func BenchmarkMyFunction(b *testing.B) { for i := 0; i < b.N; i { MyFunction() } }
Copy after login Run the Benchmark: Use the
go test
command with the-bench
flag to run your benchmarks. To run all benchmarks in a package, you can use:<code>go test -bench=.</code>
Copy after loginCopy after loginIf you want to run a specific benchmark, you can specify its name:
<code>go test -bench=BenchmarkMyFunction</code>
Copy after login- Analyze Results: The output of the benchmark will include the number of iterations
b.N
, the total time taken, and the time per operation. You can track these results over time to measure performance improvements. - Iterate and Optimize: Based on the benchmark results, you can make changes to your code and rerun the benchmarks to see if your optimizations have led to performance improvements.
What specific metrics can Go's benchmarking tools provide to help identify performance bottlenecks?
Go's benchmarking tools provide several metrics that can help identify performance bottlenecks:
- Iterations (N): The number of times the benchmark function was run. This is automatically set by Go to ensure accurate measurements.
- Time per Operation (ns/op): The average time in nanoseconds taken for a single operation. This is the primary metric used to measure the performance of a piece of code.
- Bytes per Operation (B/op): The average amount of memory, in bytes, allocated per operation. This helps identify memory usage patterns and potential memory bottlenecks.
- Allocs per Operation (allocs/op): The average number of memory allocations per operation. High numbers here might indicate excessive memory usage or inefficient allocation strategies.
- Benchmark Duration: The total time the benchmark took to run. This is useful for understanding the overall impact of your benchmark on the system.
These metrics can be used to pinpoint areas where performance can be improved, such as reducing the time per operation, minimizing memory allocations, or optimizing memory usage.
How can you set up and run benchmarks in Go to compare different versions of your code?
To compare different versions of your code using Go's benchmarking tools, follow these steps:
- Write Benchmark Functions: As mentioned earlier, define benchmark functions in your test files using the
Benchmark
prefix. Ensure that these functions cover the parts of your code you want to compare. - Version Control: Use version control systems like Git to manage different versions of your code. Create branches for each version you want to benchmark.
Run Benchmarks for Each Version: Switch between different versions (branches) and run the benchmarks. Use the
go test
command with the-bench
flag:<code>go test -bench=.</code>
Copy after loginCopy after loginMake sure to run the benchmarks multiple times to account for system variability and to get consistent results.
Store Benchmark Results: You can use tools like
benchstat
to help store and compare benchmark results. To installbenchstat
, run:<code>go get golang.org/x/perf/cmd/benchstat</code>
Copy after loginThen, you can redirect the benchmark output to a file for each version:
<code>go test -bench=. > version1.txt</code>
Copy after loginSwitch to the next version and run again:
<code>go test -bench=. > version2.txt</code>
Copy after loginCompare Results: Use
benchstat
to compare the benchmark results:<code>benchstat version1.txt version2.txt</code>
Copy after loginThis will provide a statistical comparison of the results, showing any significant differences between the versions.
How do you interpret benchmark results in Go to make informed decisions about performance optimization?
Interpreting benchmark results in Go involves understanding the metrics provided and making data-driven decisions about performance optimization. Here's how you can interpret these results:
-
Time per Operation (ns/op): This is the most critical metric. A lower
ns/op
indicates better performance. If you see a significant reduction inns/op
after optimizing your code, it means your optimizations have improved performance. -
Bytes per Operation (B/op) and Allocs per Operation (allocs/op): These metrics help you understand memory usage and allocation patterns. A decrease in
B/op
andallocs/op
suggests your code is using memory more efficiently. If these metrics are high, consider optimizing memory usage or reducing allocations. -
Statistical Significance: Use tools like
benchstat
to determine if the changes in benchmark results are statistically significant. This helps you avoid making decisions based on random fluctuations. - Consistency: Run benchmarks multiple times to ensure consistency. If results vary widely, it might indicate that the benchmark is sensitive to system conditions or that the code's performance is unstable.
-
Contextual Analysis: Consider the context of your application. For example, if your application is CPU-bound, focus on reducing
ns/op
. If it's memory-bound, focus onB/op
andallocs/op
. -
Holistic Approach: Look at all the metrics together. Sometimes, optimizing one metric (e.g., reducing
ns/op
) might increase another (e.g., increasingB/op
). Ensure that your optimizations balance these metrics appropriately.
By carefully analyzing these metrics and understanding their implications, you can make informed decisions about where to focus your optimization efforts and how to improve your code's performance.
The above is the detailed content of How can you use Go's built-in benchmarking tools to measure performance improvements?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
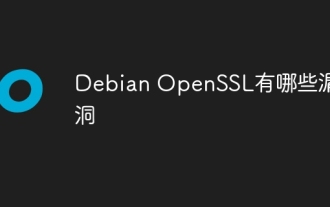
OpenSSL, as an open source library widely used in secure communications, provides encryption algorithms, keys and certificate management functions. However, there are some known security vulnerabilities in its historical version, some of which are extremely harmful. This article will focus on common vulnerabilities and response measures for OpenSSL in Debian systems. DebianOpenSSL known vulnerabilities: OpenSSL has experienced several serious vulnerabilities, such as: Heart Bleeding Vulnerability (CVE-2014-0160): This vulnerability affects OpenSSL 1.0.1 to 1.0.1f and 1.0.2 to 1.0.2 beta versions. An attacker can use this vulnerability to unauthorized read sensitive information on the server, including encryption keys, etc.
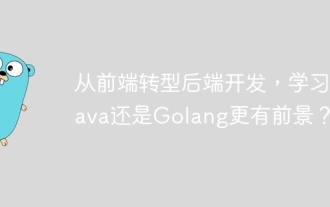
Backend learning path: The exploration journey from front-end to back-end As a back-end beginner who transforms from front-end development, you already have the foundation of nodejs,...
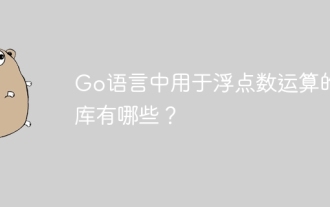
The library used for floating-point number operation in Go language introduces how to ensure the accuracy is...
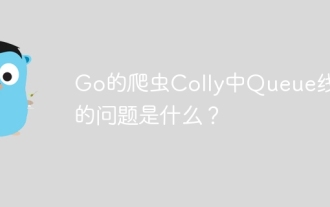
Queue threading problem in Go crawler Colly explores the problem of using the Colly crawler library in Go language, developers often encounter problems with threads and request queues. �...
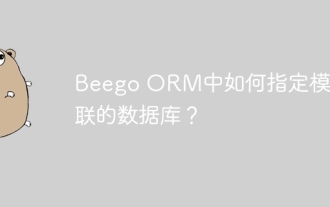
Under the BeegoORM framework, how to specify the database associated with the model? Many Beego projects require multiple databases to be operated simultaneously. When using Beego...
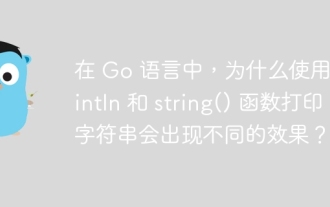
The difference between string printing in Go language: The difference in the effect of using Println and string() functions is in Go...
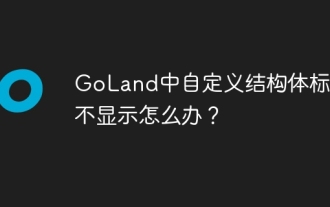
What should I do if the custom structure labels in GoLand are not displayed? When using GoLand for Go language development, many developers will encounter custom structure tags...
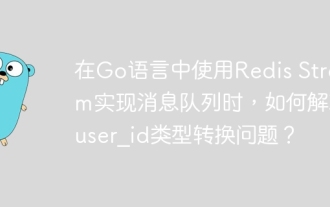
The problem of using RedisStream to implement message queues in Go language is using Go language and Redis...
