


Explain the differences between multithreading, multiprocessing, and asynchronous programming in Python. When would you use each?
Explain the differences between multithreading, multiprocessing, and asynchronous programming in Python. When would you use each?
Multithreading:
Multithreading in Python refers to the ability of a program to execute multiple threads concurrently within the same process. Threads are lighter than processes and share the same memory space. The Global Interpreter Lock (GIL) in CPython, however, limits the true parallelism of threads, making them execute one at a time. Multithreading is suitable for I/O-bound tasks, where threads can switch between waiting for I/O operations. Examples include handling multiple client connections in a server, or GUI applications where responsiveness is crucial.
Multiprocessing:
Multiprocessing involves running multiple processes in parallel, each with its own memory space. Since processes do not share memory, they bypass the GIL and can utilize multiple CPU cores effectively. Multiprocessing is ideal for CPU-bound tasks, where you can distribute the workload across multiple processors. Use cases include scientific computing, data processing, and any computationally intensive operations where performance benefits from parallel execution.
Asynchronous Programming:
Asynchronous programming in Python, often implemented with asyncio
, allows for non-blocking operations. This means that a task can start an I/O operation and continue executing other tasks without waiting for the I/O to complete. When the I/O operation is finished, the task can resume where it left off. Asynchronous programming is perfect for I/O-bound tasks and scenarios where you need to handle multiple I/O operations concurrently, such as web scraping, API calls, or handling numerous network connections efficiently.
When to Use Each:
- Multithreading: Use for I/O-bound tasks where the overhead of creating processes is undesirable, and you need to maintain shared state easily. Ideal for GUI applications, simple network servers, and other scenarios where responsiveness is important.
- Multiprocessing: Use for CPU-bound tasks where you want to leverage the full potential of multiple cores. Suitable for data processing, scientific computing, and any task that benefits from parallel processing.
- Asynchronous Programming: Use for I/O-bound tasks that involve multiple concurrent I/O operations. Best for web applications, handling multiple network connections, and scenarios where non-blocking I/O can significantly improve performance.
What are the specific scenarios where multithreading is more advantageous than multiprocessing in Python?
Multithreading can be more advantageous than multiprocessing in several specific scenarios:
- Low Overhead Requirements: Creating threads is less resource-intensive than creating processes. If you have a task that requires many lightweight concurrent operations, multithreading will have less overhead.
- Shared State: Threads share the same memory space, making it easier to share data between threads without complex inter-process communication mechanisms. This is beneficial for scenarios where frequent data sharing is necessary, such as in certain GUI applications or shared resource management.
- I/O-Bound Operations: For I/O-bound tasks, multithreading can be more efficient because threads can switch to other tasks while waiting for I/O operations to complete. This makes multithreading ideal for applications like web servers or database clients that spend most of their time waiting for I/O.
- Responsiveness in GUI Applications: Multithreading is often used in GUI programming to keep the interface responsive while performing background tasks. By using threads, the UI thread can continue to handle user input while other threads perform time-consuming operations.
- Limited CPU Resources: In environments with limited CPU resources or on systems where you cannot utilize multiprocessing (due to licensing or other restrictions), multithreading is a better choice for achieving concurrency.
How does asynchronous programming improve the efficiency of I/O-bound tasks in Python?
Asynchronous programming improves the efficiency of I/O-bound tasks in Python through several mechanisms:
- Non-blocking I/O: Asynchronous programming allows I/O operations to be non-blocking. Instead of waiting for an I/O operation to complete, the program can continue executing other tasks. When the I/O operation finishes, the program can resume where it left off. This maximizes the utilization of system resources by minimizing idle time.
-
Event Loop: The
asyncio
library in Python uses an event loop to manage and schedule tasks. The event loop keeps track of all pending tasks and can switch between them efficiently. This allows multiple I/O operations to be in progress simultaneously, improving overall throughput. - Coroutines: Asynchronous programming in Python is often implemented using coroutines, which are special functions that can pause and resume execution. Coroutines allow a single thread to handle many tasks by switching between them based on I/O readiness. This approach can handle thousands of concurrent connections with minimal resource consumption.
- Reduced Resource Usage: Asynchronous programming typically requires fewer system resources than traditional multithreading or multiprocessing approaches. Since it uses a single thread (or a small number of threads) to manage many tasks, it is more efficient in terms of memory and CPU usage.
- Scalability: Asynchronous programming scales well with I/O-bound workloads. For example, a web server using asynchronous programming can handle a high number of simultaneous connections more efficiently than a traditional synchronous server.
By leveraging these mechanisms, asynchronous programming can significantly enhance the performance and efficiency of I/O-bound tasks, making it an excellent choice for applications dealing with network communication, file operations, or any scenario involving frequent I/O waits.
In what situations would you choose multiprocessing over multithreading or asynchronous programming in Python?
You would choose multiprocessing over multithreading or asynchronous programming in Python in the following situations:
- CPU-Bound Tasks: When dealing with computationally intensive tasks that can benefit from parallel execution across multiple CPU cores, multiprocessing is the best choice. Since processes bypass the GIL, they can truly run in parallel, making them ideal for tasks like data processing, scientific computing, and machine learning.
- High-Performance Computing: In scenarios where you need to maximize the utilization of available CPU resources, multiprocessing can provide significant performance improvements. This is particularly important in applications such as financial modeling, video encoding, and other high-performance computing tasks.
- Memory-Intensive Applications: If your application requires large amounts of memory, and you need to distribute the memory usage across multiple processes, multiprocessing is more suitable. Each process has its own memory space, which can help manage memory more effectively.
- Isolation and Stability: When you need to ensure that different parts of your application do not interfere with each other, multiprocessing can provide better isolation. If one process crashes, it will not affect the others, making it a safer choice for mission-critical applications.
- Distributed Computing: In scenarios where you need to distribute tasks across multiple machines or in a distributed computing environment, multiprocessing can be extended to utilize multiple nodes. This is common in big data processing and cloud computing environments.
- Complex Workflows: If you have a workflow that involves multiple independent tasks that can be executed in parallel, multiprocessing can efficiently manage and coordinate these tasks. This is often seen in pipeline-based data processing systems.
In summary, multiprocessing is the preferred choice when you need true parallelism for CPU-bound tasks, need to isolate parts of your application, or when working in high-performance computing environments where leveraging multiple cores is crucial.
The above is the detailed content of Explain the differences between multithreading, multiprocessing, and asynchronous programming in Python. When would you use each?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
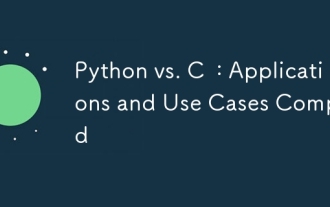
Python is suitable for data science, web development and automation tasks, while C is suitable for system programming, game development and embedded systems. Python is known for its simplicity and powerful ecosystem, while C is known for its high performance and underlying control capabilities.
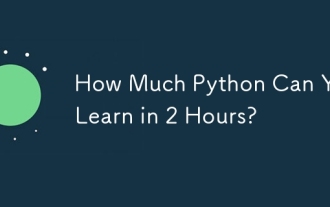
You can learn the basics of Python within two hours. 1. Learn variables and data types, 2. Master control structures such as if statements and loops, 3. Understand the definition and use of functions. These will help you start writing simple Python programs.
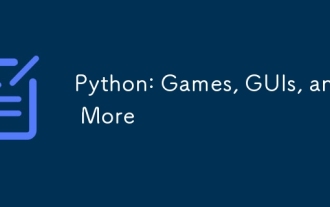
Python excels in gaming and GUI development. 1) Game development uses Pygame, providing drawing, audio and other functions, which are suitable for creating 2D games. 2) GUI development can choose Tkinter or PyQt. Tkinter is simple and easy to use, PyQt has rich functions and is suitable for professional development.
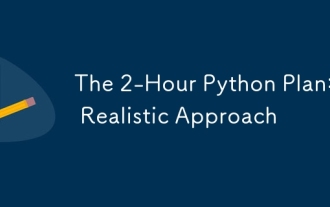
You can learn basic programming concepts and skills of Python within 2 hours. 1. Learn variables and data types, 2. Master control flow (conditional statements and loops), 3. Understand the definition and use of functions, 4. Quickly get started with Python programming through simple examples and code snippets.
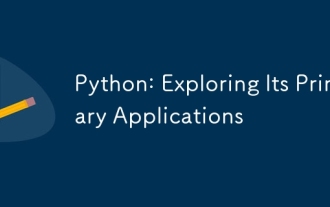
Python is widely used in the fields of web development, data science, machine learning, automation and scripting. 1) In web development, Django and Flask frameworks simplify the development process. 2) In the fields of data science and machine learning, NumPy, Pandas, Scikit-learn and TensorFlow libraries provide strong support. 3) In terms of automation and scripting, Python is suitable for tasks such as automated testing and system management.
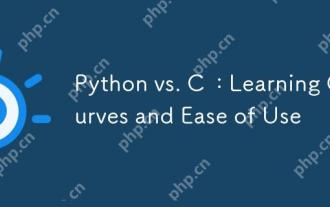
Python is easier to learn and use, while C is more powerful but complex. 1. Python syntax is concise and suitable for beginners. Dynamic typing and automatic memory management make it easy to use, but may cause runtime errors. 2.C provides low-level control and advanced features, suitable for high-performance applications, but has a high learning threshold and requires manual memory and type safety management.
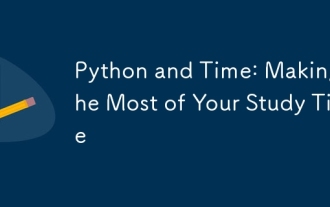
To maximize the efficiency of learning Python in a limited time, you can use Python's datetime, time, and schedule modules. 1. The datetime module is used to record and plan learning time. 2. The time module helps to set study and rest time. 3. The schedule module automatically arranges weekly learning tasks.
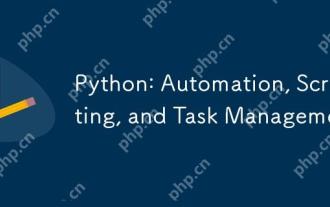
Python excels in automation, scripting, and task management. 1) Automation: File backup is realized through standard libraries such as os and shutil. 2) Script writing: Use the psutil library to monitor system resources. 3) Task management: Use the schedule library to schedule tasks. Python's ease of use and rich library support makes it the preferred tool in these areas.
