How do I use recursive CTEs in SQL for hierarchical data?
How do I use recursive CTEs in SQL for hierarchical data?
Recursive Common Table Expressions (CTEs) are powerful tools in SQL used for handling hierarchical data structures like organizational charts, file systems, or category trees. Here's a step-by-step guide on how to use them:
-
Define the Anchor Member: The first part of a recursive CTE is the anchor member, which defines the starting point of the recursion. This is a non-recursive query that returns a set of initial rows.
WITH RECURSIVE EmployeeHierarchy AS ( SELECT id, name, manager_id, 0 AS level FROM Employees WHERE manager_id IS NULL -- Start from the top level (e.g., CEO)
Copy after login Define the Recursive Member: Following the anchor member, the recursive member defines how the recursion proceeds. It references the CTE itself to build upon the rows returned from the previous iteration.
UNION ALL SELECT e.id, e.name, e.manager_id, level 1 FROM Employees e INNER JOIN EmployeeHierarchy m ON e.manager_id = m.id )
Copy after loginCombine the Results: The recursive CTE keeps building on itself until no new rows are generated. You then query the CTE to get the desired results.
SELECT id, name, level FROM EmployeeHierarchy;
Copy after login
This example builds an employee hierarchy starting from the top (where manager_id
is NULL
) and recursively adds subordinates to each level until all employees are included.
What are the best practices for optimizing recursive CTEs in SQL?
Optimizing recursive CTEs involves several strategies to improve performance and reduce resource usage:
Limit the Depth of Recursion: Be aware of the depth of your recursion. If possible, implement a
WHERE
clause to cap the maximum depth.WHERE level < 10
Copy after loginCopy after login- Use Indexes: Ensure that columns used in the recursive joins and filters are indexed. For the example above, index
manager_id
andid
in theEmployees
table. - Materialized Paths or Nested Sets: If possible, consider using alternative hierarchical models like materialized paths or nested sets, which can be more performant for certain queries.
- Avoid Cartesian Products: Make sure your recursive member doesn't inadvertently create a Cartesian product, which could exponentially increase the result set.
- Optimize Anchor and Recursive Queries: Ensure that both the anchor and recursive parts of the CTE are as optimized as possible. Use efficient join types and limit the columns selected.
- Testing and Profiling: Regularly test and profile your queries to identify and resolve performance bottlenecks.
How can I troubleshoot common errors when using recursive CTEs for hierarchical data?
When working with recursive CTEs, you may encounter several types of errors. Here are some common issues and how to troubleshoot them:
Infinite Loops: If the recursive part of the CTE keeps referencing itself without a stopping condition, it can cause an infinite loop. Ensure that your recursion has a clear termination condition.
WHERE level < 10
Copy after loginCopy after login- Data Inconsistencies: If the data in your hierarchical structure has inconsistencies (e.g., cycles), it can cause issues. Validate your data to ensure there are no self-referencing entries or cycles.
- Performance Issues: If the CTE is taking too long to execute, check if there are unnecessary joins or if you're querying too much data. Optimize the query as suggested in the best practices section.
- Syntax Errors: Ensure that the syntax for your recursive CTE is correct. The anchor and recursive members should be separated by
UNION ALL
, and the recursive reference should be in theFROM
clause of the recursive member. - Stack Overflow: Depending on your database system, deep recursions can cause stack overflow errors. Implement a maximum depth as a safeguard.
What are some alternatives to recursive CTEs for managing hierarchical data in SQL?
While recursive CTEs are powerful for handling hierarchical data, there are alternative methods that may be more suitable depending on your specific use case:
Adjacency List Model: This model stores the immediate parent-child relationship. It is simple but may require multiple queries or self-joins to navigate the hierarchy.
CREATE TABLE Employees ( id INT PRIMARY KEY, name VARCHAR(100), manager_id INT, FOREIGN KEY (manager_id) REFERENCES Employees(id) );
Copy after loginMaterialized Path: This model stores the entire path from the root to each node as a string. It is good for quick retrieval of entire paths but can become complex with frequent updates.
CREATE TABLE Categories ( id INT PRIMARY KEY, name VARCHAR(100), path VARCHAR(1000) );
Copy after loginNested Sets: This model assigns left and right values to each node, which can be used to determine parent-child relationships efficiently. It's good for queries that need to traverse hierarchies quickly but can be tricky to update.
CREATE TABLE Categories ( id INT PRIMARY KEY, name VARCHAR(100), lft INT, rgt INT );
Copy after loginClosure Table: This model stores all ancestor-descendant relationships, making it efficient for queries involving paths but requiring more storage space.
CREATE TABLE EmployeeHierarchy ( ancestor INT, descendant INT, PRIMARY KEY (ancestor, descendant), FOREIGN KEY (ancestor) REFERENCES Employees(id), FOREIGN KEY (descendant) REFERENCES Employees(id) );
Copy after login
Each of these models has its strengths and weaknesses, and the choice depends on the specific needs of your application, including the type of queries you need to perform and the frequency of data changes.
The above is the detailed content of How do I use recursive CTEs in SQL for hierarchical data?. For more information, please follow other related articles on the PHP Chinese website!

Hot AI Tools

Undresser.AI Undress
AI-powered app for creating realistic nude photos

AI Clothes Remover
Online AI tool for removing clothes from photos.

Undress AI Tool
Undress images for free

Clothoff.io
AI clothes remover

Video Face Swap
Swap faces in any video effortlessly with our completely free AI face swap tool!

Hot Article

Hot Tools

Notepad++7.3.1
Easy-to-use and free code editor

SublimeText3 Chinese version
Chinese version, very easy to use

Zend Studio 13.0.1
Powerful PHP integrated development environment

Dreamweaver CS6
Visual web development tools

SublimeText3 Mac version
God-level code editing software (SublimeText3)

Hot Topics
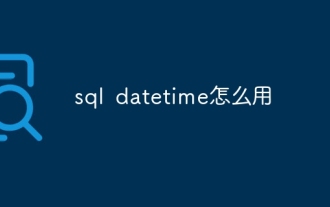
The DATETIME data type is used to store high-precision date and time information, ranging from 0001-01-01 00:00:00 to 9999-12-31 23:59:59.99999999, and the syntax is DATETIME(precision), where precision specifies the accuracy after the decimal point (0-7), and the default is 3. It supports sorting, calculation, and time zone conversion functions, but needs to be aware of potential issues when converting precision, range and time zones.
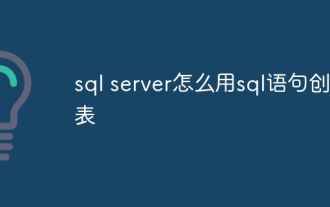
How to create tables using SQL statements in SQL Server: Open SQL Server Management Studio and connect to the database server. Select the database to create the table. Enter the CREATE TABLE statement to specify the table name, column name, data type, and constraints. Click the Execute button to create the table.
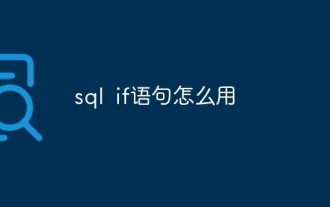
SQL IF statements are used to conditionally execute SQL statements, with the syntax as: IF (condition) THEN {statement} ELSE {statement} END IF;. The condition can be any valid SQL expression, and if the condition is true, execute the THEN clause; if the condition is false, execute the ELSE clause. IF statements can be nested, allowing for more complex conditional checks.

Common SQL optimization methods include: Index optimization: Create appropriate index-accelerated queries. Query optimization: Use the correct query type, appropriate JOIN conditions, and subqueries instead of multi-table joins. Data structure optimization: Select the appropriate table structure, field type and try to avoid using NULL values. Query Cache: Enable query cache to store frequently executed query results. Connection pool optimization: Use connection pools to multiplex database connections. Transaction optimization: Avoid nested transactions, use appropriate isolation levels, and batch operations. Hardware optimization: Upgrade hardware and use SSD or NVMe storage. Database maintenance: run index maintenance tasks regularly, optimize statistics, and clean unused objects. Query
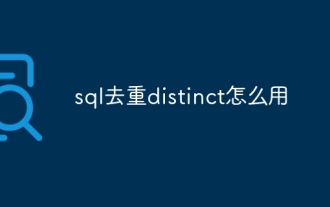
There are two ways to deduplicate using DISTINCT in SQL: SELECT DISTINCT: Only the unique values of the specified columns are preserved, and the original table order is maintained. GROUP BY: Keep the unique value of the grouping key and reorder the rows in the table.
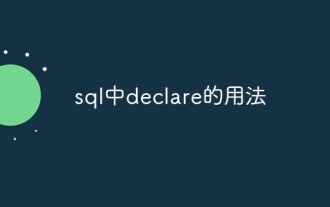
The DECLARE statement in SQL is used to declare variables, that is, placeholders that store variable values. The syntax is: DECLARE <Variable name> <Data type> [DEFAULT <Default value>]; where <Variable name> is the variable name, <Data type> is its data type (such as VARCHAR or INTEGER), and [DEFAULT <Default value>] is an optional initial value. DECLARE statements can be used to store intermediates

SQL paging is a technology that searches large data sets in segments to improve performance and user experience. Use the LIMIT clause to specify the number of records to be skipped and the number of records to be returned (limit), for example: SELECT * FROM table LIMIT 10 OFFSET 20; advantages include improved performance, enhanced user experience, memory savings, and simplified data processing.
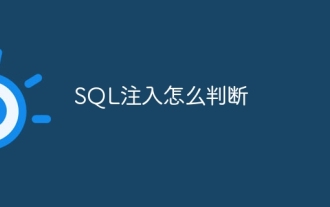
Methods to judge SQL injection include: detecting suspicious input, viewing original SQL statements, using detection tools, viewing database logs, and performing penetration testing. After the injection is detected, take measures to patch vulnerabilities, verify patches, monitor regularly, and improve developer awareness.
